Avoid Bugs: Master Type-Safe Empty Collections in Java
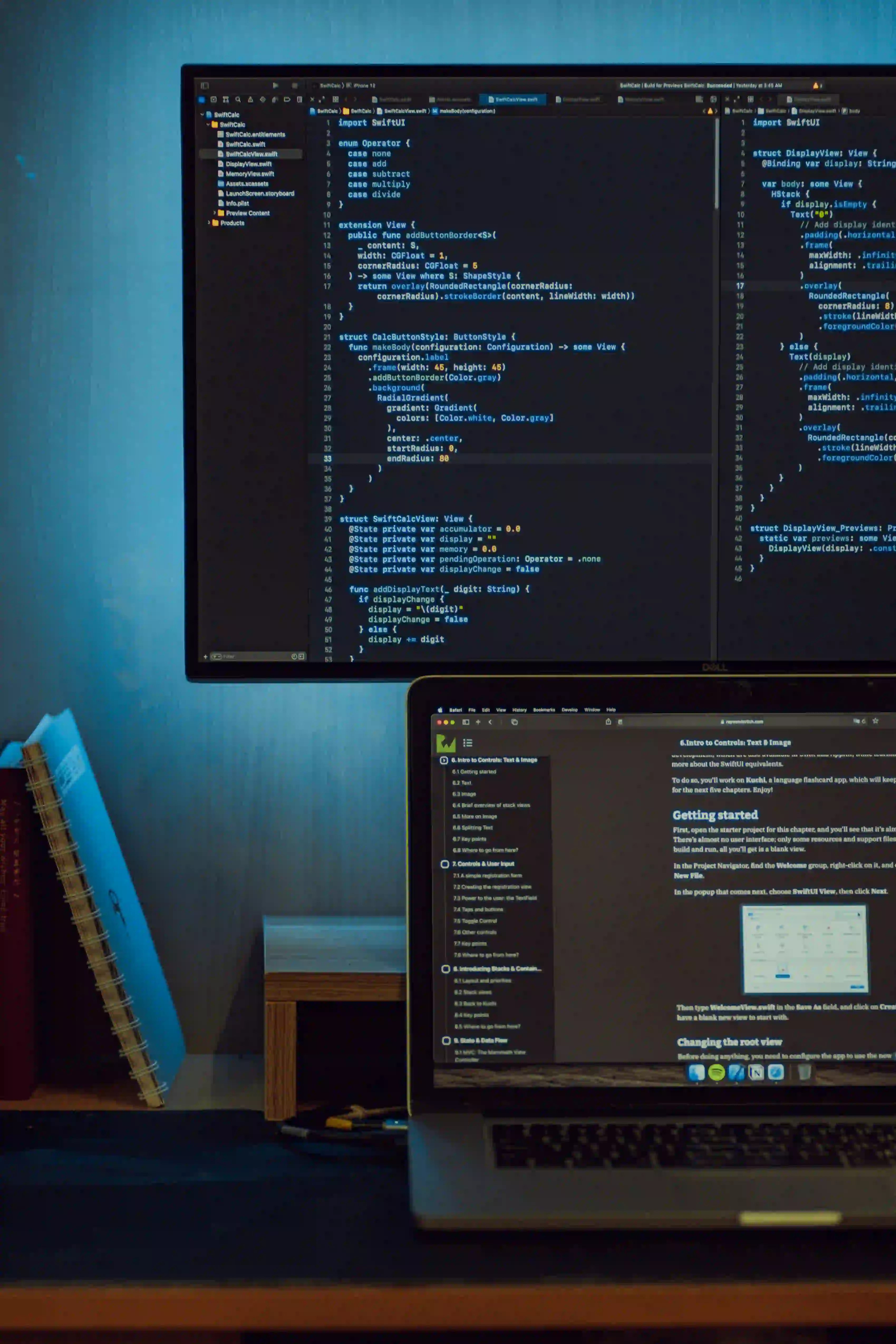
Avoid Bugs: Master Type-Safe Empty Collections in Java
Empty collections are a common and useful tool in Java programming. They represent a collection that contains no elements, allowing for cleaner code and better performance in certain scenarios. However, it can be tricky to create type-safe empty collections in Java, and doing so incorrectly can introduce bugs and errors into your code.
In this article, we'll explore the importance of type-safe empty collections, common pitfalls to avoid, and best practices for mastering them in your Java code. By the end, you'll have a solid understanding of how to leverage type-safe empty collections effectively to write cleaner, safer, and more performant Java code.
Why Use Empty Collections?
Empty collections provide a convenient and efficient way to represent the absence of elements in a collection. Consider scenarios where returning null
to signify an empty collection can lead to null pointer exceptions and additional null checks. By using empty collections, you can avoid these issues and produce more robust and predictable code.
// Returning null to signify an empty collection
List<String> getNames() {
if (someCondition) {
return null;
} else {
return Arrays.asList("Alice", "Bob", "Charlie");
}
}
// Using an empty collection instead
List<String> getNames() {
if (someCondition) {
return Collections.emptyList();
} else {
return Arrays.asList("Alice", "Bob", "Charlie");
}
}
By consistently using empty collections instead of null
, you can avoid null pointer exceptions and improve the overall quality of your code.
The Problem with Basic Empty Collections
While Collections.emptyList()
and similar methods are a step in the right direction, they suffer from a lack of type safety. It returns a List
with an unbounded wildcard type (List<?>
), which can lead to potential bugs and unexpected runtime errors. Consider the following scenario:
List<String> names = Collections.emptyList();
names.add("David"); // Compiles, but throws UnsupportedOperationException at runtime
The code compiles without error, but a runtime exception occurs when trying to add an element to the empty, unmodifiable list. This lack of type safety can introduce subtle bugs that are difficult to catch during development.
Mastering Type-Safe Empty Collections
To overcome the limitations of basic empty collections, we can leverage Java's generics to create type-safe empty collections for different types. Let's take a look at how we can achieve this for List
, Set
, and Map
types.
Type-Safe Empty List
To create a type-safe empty List
, we can utilize the diamond operator (<>
) introduced in Java 7. This allows the compiler to infer the type based on the context, ensuring type safety.
List<String> emptyList = new ArrayList<>();
By omitting the type parameter on the right-hand side of the assignment, the compiler infers the type to be List<String>
, providing type safety without additional verbosity.
Type-Safe Empty Set
Similar to creating an empty List
, we can use the diamond operator to create a type-safe empty Set
.
Set<Integer> emptySet = new HashSet<>();
This concise approach ensures type safety while maintaining clarity in the code.
Type-Safe Empty Map
For empty maps, we can employ the diamond operator to create a type-safe empty Map
.
Map<String, Integer> emptyMap = new HashMap<>();
The diamond operator allows us to create a type-safe empty map without redundant type declarations.
Utilizing Optional for Empty Single Values
In addition to empty collections, Java 8 introduced Optional
to represent an optional value that may or may not be present. It can be used to avoid returning null
for single values and explicitly handle the absence of a value.
Optional<String> optionalName = Optional.ofNullable(getName());
By wrapping the potentially null value in an Optional
, we can safely work with it using methods like isPresent()
, ifPresent()
, and orElse()
. This approach promotes more explicit and predictable code, reducing the likelihood of null pointer exceptions.
Best Practices for Using Type-Safe Empty Collections
When working with type-safe empty collections in Java, it's important to adhere to best practices to ensure consistency and maintainability across your codebase. Here are some recommendations to consider:
1. Use Type Inference
Leverage type inference (e.g., diamond operator) to create type-safe empty collections without explicitly specifying the type on the right-hand side of the assignment. This promotes cleaner and more concise code while ensuring type safety.
2. Prefer Immutable Collections
Consider using immutable empty collections from libraries like Google's Guava or the Collections
utility class to prevent accidental modifications. Immutable collections provide additional safety against unintended state changes.
3. Document Empty Collections Appropriately
When returning empty collections from methods, document the behavior clearly to convey that an empty collection is intentionally being returned. This helps other developers understand the expected behavior and prevents potential misunderstandings.
4. Handle Optional Values Expressively
When dealing with potentially absent single values, opt for Optional
instead of returning null
. Expressive use of Optional
promotes explicit handling of absence and enhances code readability.
Closing the Chapter
In Java programming, mastering type-safe empty collections is crucial for writing robust, predictable, and bug-free code. By leveraging type inference, immutability, and well-documented practices, you can ensure that empty collections are used effectively throughout your codebase. Additionally, embracing Optional
for single values can further enhance the safety and clarity of your code.
By incorporating type-safe empty collections and Optional
into your Java programming toolkit, you can minimize bugs, improve code quality, and create more maintainable software.
Start incorporating type-safe empty collections and Optional
into your Java code today to elevate the quality of your software and avoid common pitfalls associated with null references and basic empty collections.
References: