Inheritance Flaws in OOP: Unraveling Solutions for 2023
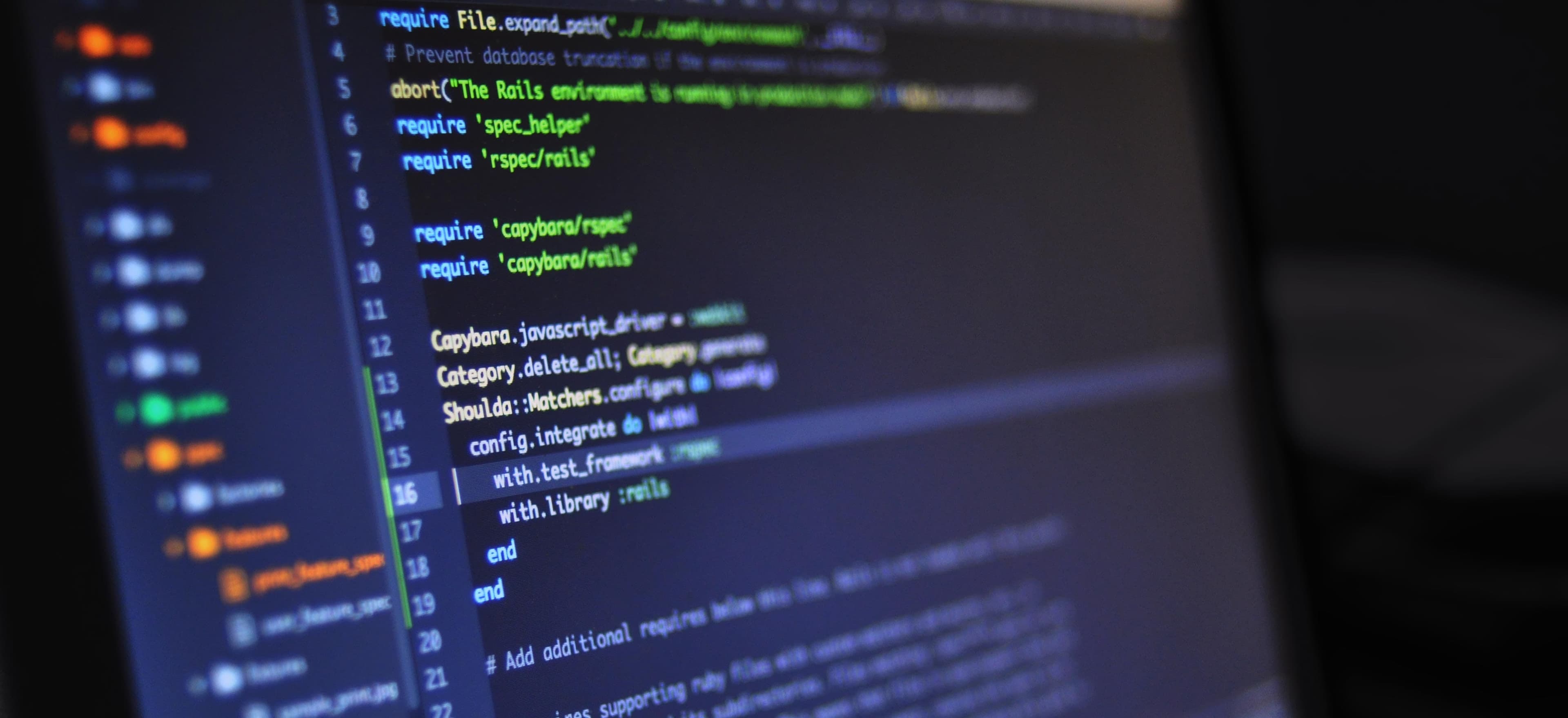
- Published on
Unveiling Inheritance Flaws in Object-Oriented Programming and Solutions for 2023
Object-oriented programming (OOP) has been a cornerstone in software development for decades. At the heart of OOP lies the concept of inheritance, allowing classes to inherit properties and behaviors from other classes. However, as we march into 2023, it's becoming increasingly apparent that the traditional use of inheritance in OOP has its flaws, leading to brittle and tightly-coupled code. In this article, we'll dissect the flaws of inheritance in OOP and propose modern solutions to address these issues.
Understanding the Flaws of Inheritance
Tight Coupling
Inheritance creates a strong coupling between the subclasses and superclasses, making it challenging to modify the superclass without affecting the subclasses. This tight coupling diminishes the flexibility and maintainability of the codebase.
Fragile Base Class Problem
The fragile base class problem occurs when modifications to a base class adversely affect the subclasses. This can lead to unforeseen bugs and ripple effects throughout the codebase, making it arduous to introduce changes.
Hierarchical Coupling
Inheritance hierarchies can become excessively deep and overly complex, resulting in a rigid class structure that is difficult to extend and maintain.
Modern Solutions to Inheritance Flaws
Composition Over Inheritance
Instead of relying solely on inheritance, favoring composition allows for more flexible and loosely-coupled designs. By composing classes with other classes or interfaces, developers can create modular and easily maintainable code.
public interface Engine {
void start();
}
public class ElectricEngine implements Engine {
public void start() {
// Start the electric engine
}
}
public class Car {
private Engine engine;
public Car(Engine engine) {
this.engine = engine;
}
public void start() {
engine.start();
}
}
The use of composition enables classes to encapsulate behavior and delegate tasks to other classes, promoting code reusability and reducing the impact of changes.
Favoring Interfaces
Embracing interfaces over concrete base classes allows for polymorphism without introducing the issues associated with deep inheritance hierarchies. Interfaces define contracts for behavior, enabling classes to adhere to these contracts without being tightly bound to a specific implementation.
public interface Shape {
double calculateArea();
}
public class Circle implements Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
public double calculateArea() {
return Math.PI * radius * radius;
}
}
By using interfaces, classes can exhibit polymorphic behavior without the drawbacks of traditional inheritance, fostering a more agile and adaptable codebase.
Applying the Decorator Pattern
The decorator pattern offers an alternative to subclassing for extending the behavior of classes at runtime. By dynamically adding new functionality to objects, the decorator pattern promotes code flexibility and scalability, without resorting to deep class hierarchies.
public interface Pizza {
String getDescription();
double cost();
}
public class Margherita implements Pizza {
public String getDescription() {
return "Margherita Pizza";
}
public double cost() {
return 100.0;
}
}
public class ExtraCheeseDecorator implements Pizza {
private Pizza pizza;
public ExtraCheeseDecorator(Pizza pizza) {
this.pizza = pizza;
}
public String getDescription() {
return pizza.getDescription() + ", Extra Cheese";
}
public double cost() {
return pizza.cost() + 20.0;
}
}
By leveraging the decorator pattern, classes can be extended with new functionalities in a modular and composable manner, mitigating the need for extensive inheritance chains.
Key Takeaways
As the software development landscape continues to evolve, it is imperative to reevaluate established practices and seek modern solutions to perennial issues. Inheritance has long been a fundamental concept in OOP, but its flaws have become increasingly evident. By embracing composition, interfaces, and the decorator pattern, developers can transcend the limitations of traditional inheritance, crafting resilient, maintainable, and adaptable codebases in 2023 and beyond.
In light of this, it's crucial for developers to stay updated with the latest trends and best practices in Java programming. Stay tuned for more insightful content on modern Java development.
Checkout our other articles