Mastering App Tests: Key Scenarios for Web & Mobile Success
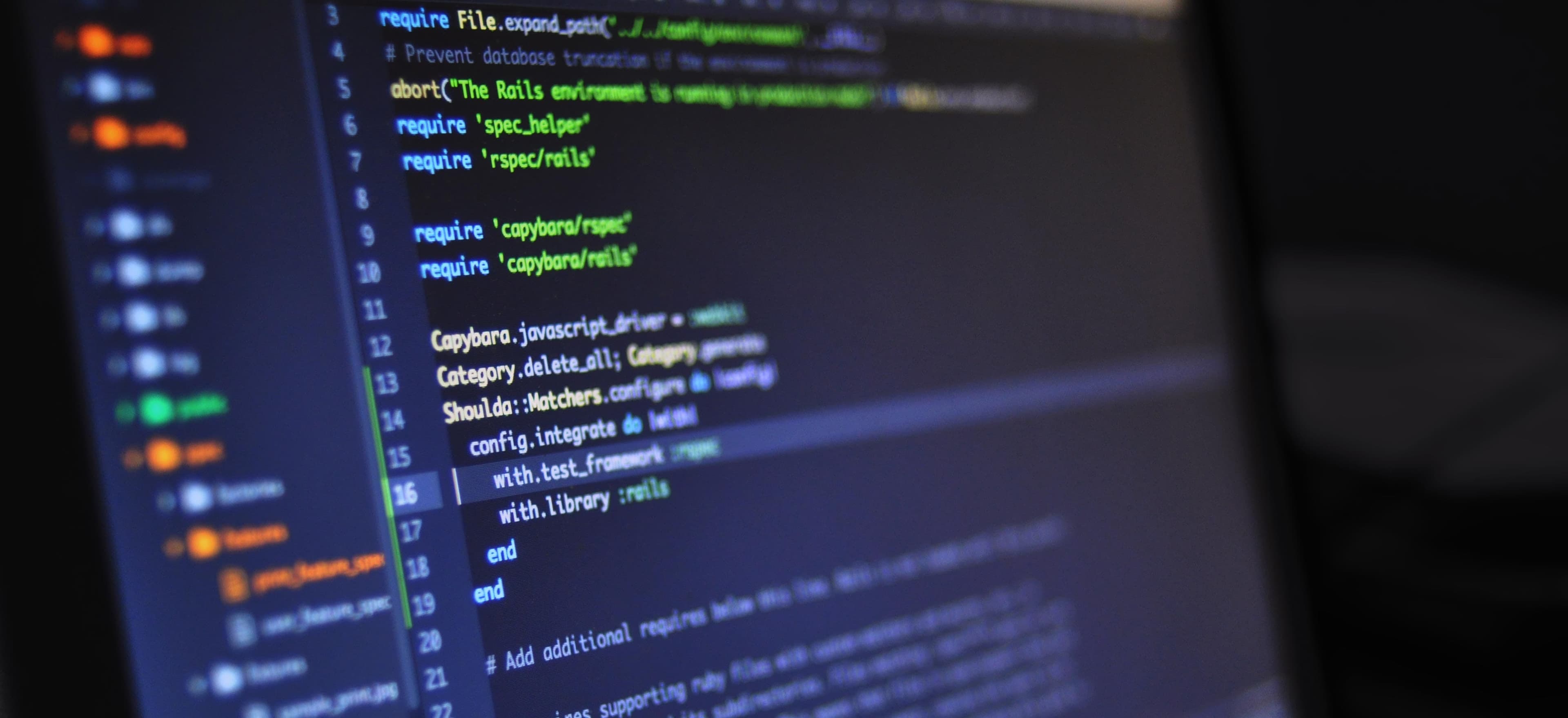
- Published on
Mastering App Tests: Key Scenarios for Web & Mobile Success
In today's hyper-competitive digital landscape, delivering flawless web and mobile applications is critical for businesses to stand out. One of the most fundamental practices for ensuring high-quality applications is thorough testing. Testing your Java web and mobile applications not only helps in identifying and fixing bugs but also enhances the overall user experience.
In this article, we will delve into key test scenarios for Java web and mobile applications that will help you master the art of app testing and set your projects up for success.
Understanding Key Test Scenarios
Web Application Test Scenarios
-
User Authentication
- Scenario: Testing the login and logout functionality.
- Importance: Verifying that users can securely log in and out of the system is essential for protecting sensitive data and maintaining user privacy.
- Sample Code:
@Test public void testUserAuthentication() { // Simulate user login // Assert login success // Simulate user logout // Assert logout success }
-
Input Validation
- Scenario: Testing form submissions with invalid data.
- Importance: Ensuring that the application properly handles and validates user input, preventing potential security vulnerabilities and data corruption.
- Sample Code:
@Test public void testInputValidation() { // Simulate form submission with invalid data // Assert error messages or validation constraints }
-
Integration with APIs
- Scenario: Testing interactions with external APIs.
- Importance: Verifying that the web application properly communicates with external services and handles responses accordingly.
- Sample Code:
@Test public void testApiIntegration() { // Mock external API responses // Assert the web application's behavior based on the responses }
Mobile Application Test Scenarios
-
Installation and Launch
- Scenario: Testing the installation and launch process of the mobile app.
- Importance: Ensuring a smooth installation and launch experience for users is crucial for the overall app impression and user retention.
- Sample Code:
@Test public void testAppInstallationAndLaunch() { // Simulate app installation // Assert successful installation // Launch the app // Assert the app launches and loads content }
-
Device Compatibility
- Scenario: Testing the app on various devices and screen sizes.
- Importance: Ensuring that the app functions correctly across different devices and screen resolutions to provide a consistent user experience.
- Sample Code:
@Test public void testDeviceCompatibility() { // Run the app on different emulators and devices // Assert consistent behavior and UI across different configurations }
-
Offline Mode
- Scenario: Testing the app's behavior when offline or with limited connectivity.
- Importance: Verifying that the app gracefully handles offline scenarios with proper data caching and minimal disruption to the user experience.
- Sample Code:
@Test public void testOfflineMode() { // Simulate offline mode // Assert that essential app features still function or provide proper offline notifications }
Tools for Testing
Web Application Testing Tools
-
JUnit: A popular Java testing framework for writing and running repeatable tests.
- Why: JUnit provides annotations for test methods, assertions for testing expected results, and test runners for executing tests.
-
Selenium: A powerful tool for automating web browser testing.
- Why: Selenium allows for automated testing of web applications across different browsers and platforms, enabling comprehensive regression testing.
-
Mockito: A mocking framework that allows the creation of mock objects in automated unit tests for Java.
- Why: Mockito simplifies the testing of interactions between classes and is particularly useful for web application testing involving dependencies.
Mobile Application Testing Tools
-
Appium: An open-source tool for automating mobile, native, and hybrid applications on iOS and Android platforms.
- Why: Appium enables code reusability across iOS and Android, making it efficient for testing mobile apps on multiple platforms.
-
Espresso: A testing framework for writing concise, beautiful, and reliable Android UI tests.
- Why: Espresso simplifies the testing of Android user interfaces by providing a fluent API for writing UI tests, ensuring a smooth user experience.
-
XCTest: Apple's native framework for testing iOS applications.
- Why: XCTest offers robust support for testing iOS apps, including performance and behavior validation under varying conditions.
Best Practices for Test Automation
-
Prioritize Test Coverage: Identify critical user flows and functionalities to ensure comprehensive test coverage, balancing between unit tests, integration tests, and end-to-end tests.
-
Use Page Object Model (POM): Implement the POM design pattern for web applications to create reusable and maintainable test code, enhancing test readability and maintenance.
-
Continuous Integration and Deployment (CI/CD): Integrate automated tests into CI/CD pipelines to ensure that tests are run with every code change, providing rapid feedback to developers.
-
Parameterized Tests: Utilize parameterized tests to run the same test with different inputs, reducing code duplication and improving the maintainability of test suites.
In Conclusion, Here is What Matters
Mastering the testing of Java web and mobile applications is crucial in delivering exceptional user experiences and maintaining the reliability of digital products. By understanding key test scenarios, utilizing relevant testing tools, and following best practices for test automation, developers and QA teams can elevate their app testing capabilities to ensure success in the competitive landscape of web and mobile applications.
Incorporating these key test scenarios and best practices into your app testing strategy will not only boost the quality of your Java applications but also contribute to increased user satisfaction and loyalty.
Remember, testing is not just about finding bugs – it's about crafting exceptional user experiences and building trust in your applications.
So, are you ready to elevate your testing game and master the art of app testing for Java web and mobile applications?
Let's make your apps shine!
Checkout our other articles