Beyond Testing: 5 Signs Your Code is Truly Production-Ready
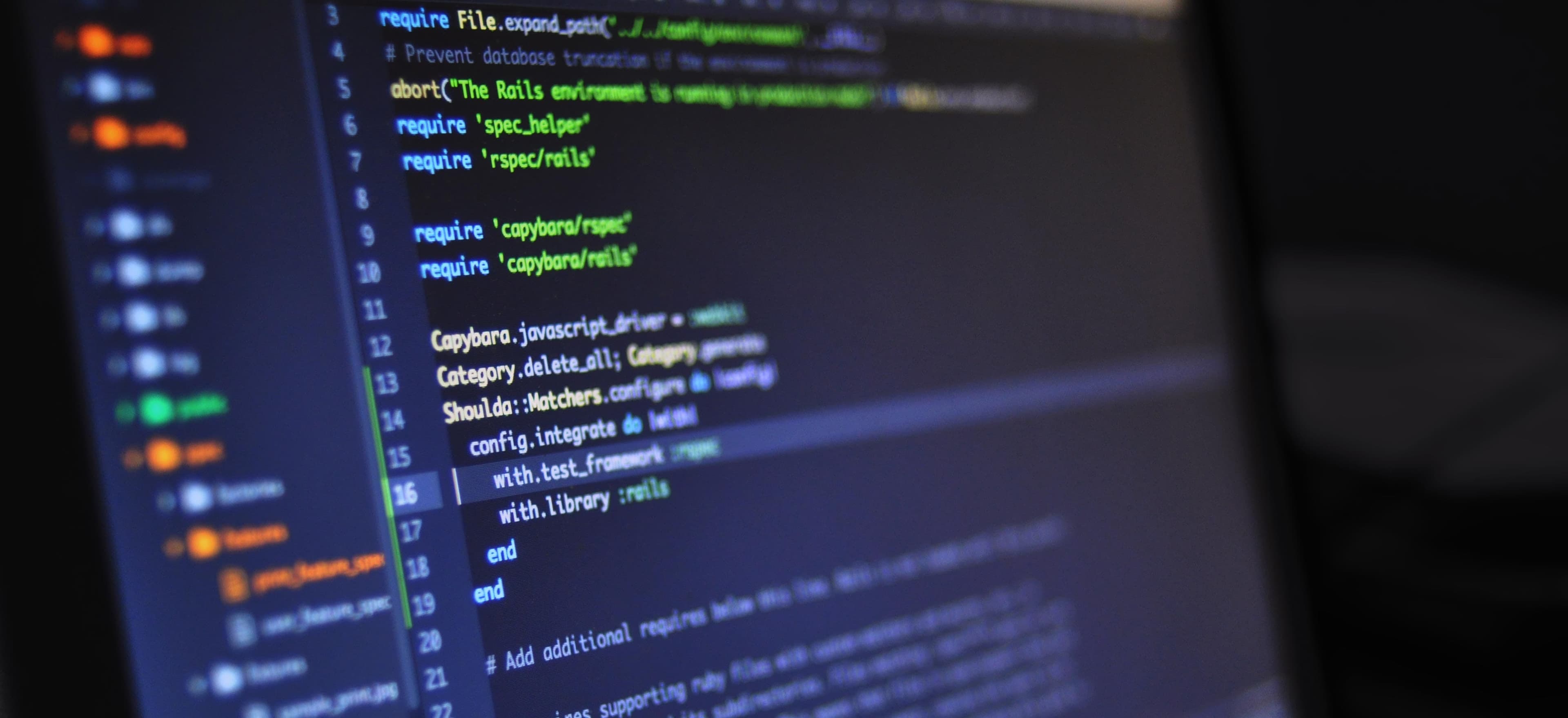
- Published on
Beyond Testing: 5 Signs Your Code is Truly Production-Ready
When it comes to programming, writing code is just the beginning. As a Java developer, you must ensure that your code not only works as intended but is also robust enough to handle the challenges of a production environment. While testing is a crucial part of the development process, it's important to look beyond that and consider other factors that contribute to the readiness of your code for production. In this post, we'll explore five signs that indicate your Java code is truly production-ready.
1. Performance Optimization
Writing code that works is one thing, but writing code that works efficiently is another. In a production environment, your code will be subjected to real-world workloads and user interactions. It's essential to optimize your code for performance to ensure that it can handle the expected load without experiencing significant slowdowns or resource bottlenecks.
Example:
public class PerformanceOptimizationExample {
public void processLargeDataset(List<Data> dataset) {
// Perform operations on the dataset
}
}
In the above example, the processLargeDataset
method should be optimized to handle large datasets efficiently, possibly by using parallel processing or optimizing loop structures. This optimization ensures that the code can perform well even with a substantial amount of data, making it production-ready.
2. Error Handling and Logging
Production environments are unpredictable, and errors are bound to occur. It's crucial to have robust error handling mechanisms in place to gracefully recover from failures and provide meaningful feedback for debugging purposes. Additionally, logging is essential for monitoring the behavior of the code in production and diagnosing issues when they arise.
Example:
public class ErrorHandlingExample {
public void performOperation() {
try {
// Code that may throw an exception
} catch (Exception e) {
// Handle the exception and log the error
logger.error("An error occurred while performing the operation", e);
}
}
}
In the above example, the performOperation
method includes a try-catch block to handle exceptions, ensuring that the code can recover from errors. Additionally, the error is logged using a logging framework, providing valuable information for troubleshooting in a production environment.
3. Security Measures
Security should be a top priority when preparing code for production. Java developers should follow best practices for secure coding, such as input validation, data encryption, and protection against common vulnerabilities like SQL injection and cross-site scripting (XSS). Integrating security measures into the code helps safeguard the application against malicious attacks in a production environment.
Example:
public class SecurityExample {
public void validateInput(String input) {
// Validate the input to prevent potential security risks
}
}
In the above example, the validateInput
method should include input validation logic to ensure that the input is safe from potential security threats. By implementing such security measures throughout the codebase, it becomes more resilient in the face of real-world security challenges.
4. Scalability and Resource Management
As the user base and workload of an application grow in a production environment, the code should be able to scale accordingly. This includes efficient management of resources such as memory, CPU, and database connections. Writing code that can gracefully handle scalability requirements ensures that the application remains responsive and stable under increased demand.
Example:
public class ScalabilityExample {
public void manageDatabaseConnection() {
// Efficiently manage database connections to prevent resource exhaustion
}
}
In the above example, the manageDatabaseConnection
method should implement connection pooling or other resource management techniques to ensure that the application can scale without running into resource limitations.
5. Code Maintenance and Readability
Production-ready code is not only about functionality but also about maintainability. Well-structured, readable code is easier to maintain and extend, allowing for seamless updates and bug fixes in a production environment. Following coding standards, documenting the code, and utilizing design patterns contribute to the readability and maintainability of the codebase.
Example:
public class ReadabilityExample {
// Good coding practices such as meaningful variable names, comments, and adherence to coding standards
private int itemCount; // Descriptive variable name
// Method documentation
/**
* Performs the specified operation
*/
public void performOperation() {
// Method body
}
}
In the above example, the code demonstrates good coding practices, including meaningful variable names and method documentation. Such practices enhance the readability of the code, making it easier for developers to understand and maintain in a production setting.
Wrapping Up
Testing is certainly an essential aspect of ensuring code quality, but true production readiness goes beyond passing tests. By considering performance optimization, error handling, security measures, scalability, and code maintainability, Java developers can prepare their code to excel in the demanding environment of production. By addressing these aspects, you can be confident that your Java code is truly production-ready and capable of meeting the challenges of real-world usage.
Remember, the journey to production readiness is an ongoing process, and continuous improvement is key to maintaining a robust and reliable codebase.
Are you interested in gaining more insights into Java programming? Check out this comprehensive guide on Java best practices to elevate your coding skills.
Checkout our other articles