Boost Your Microservices Speed: Essential Performance Tips
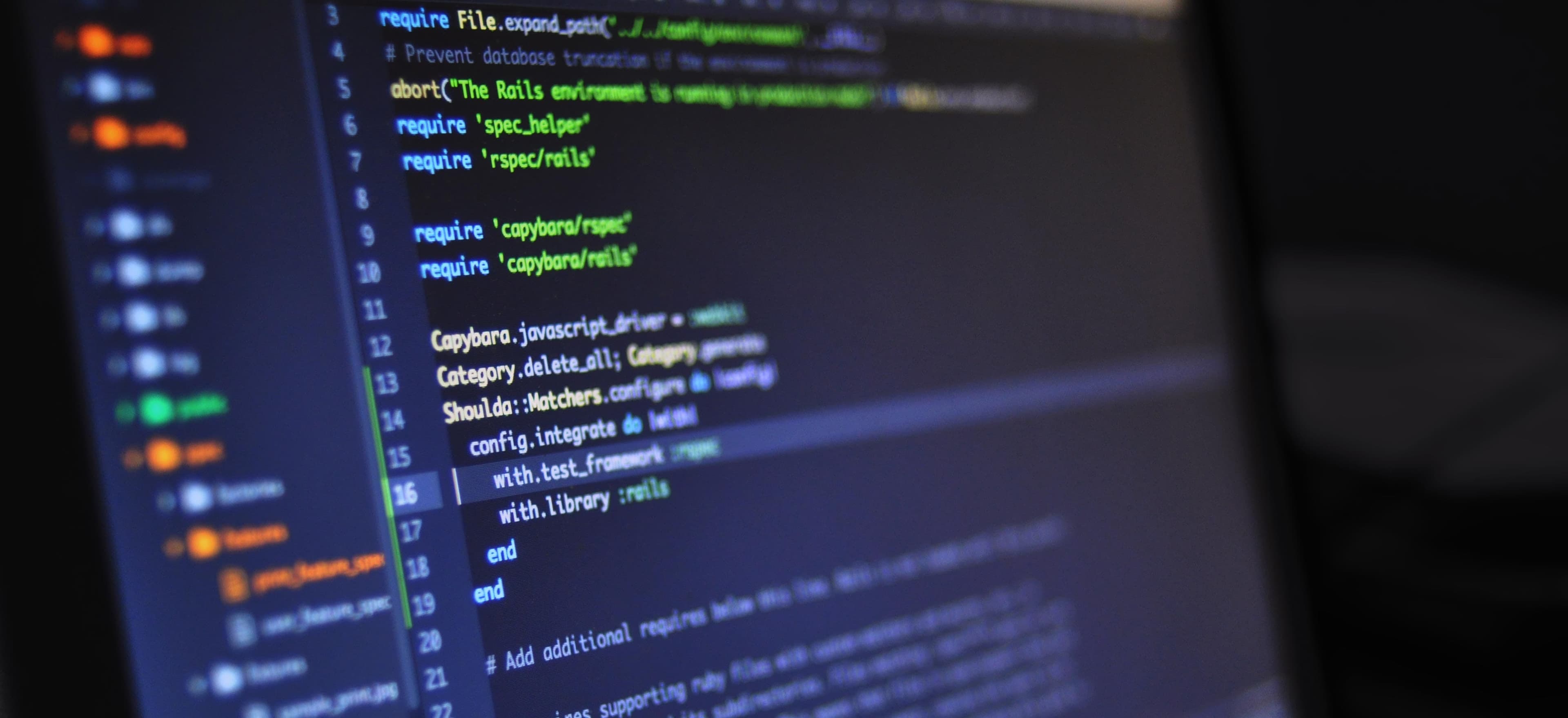
- Published on
Boost Your Microservices Speed: Essential Performance Tips
Microservices architecture has gained significant popularity for its ability to enable continuous delivery and deployment, scalability, and fault isolation. However, achieving optimal performance in a microservices environment can be challenging, given its distributed nature.
In this article, we'll explore essential performance tips to boost your microservices speed, improving response times, and overall efficiency.
1. Efficient Use of Data Storage
When dealing with microservices, efficient data storage and retrieval are crucial for maintaining optimal performance. Consider implementing a database per service pattern. This ensures that each microservice has its own database, preventing any single service from impacting the performance of others. Additionally, using specialized databases, such as Redis or Cassandra for specific data needs, can further enhance performance.
Example:
// Using a dedicated database for each microservice
@Service
public class ProductService {
@Autowired
private ProductRepository productRepository;
// Service logic
}
In the example above, the ProductService
utilizes its dedicated database through the ProductRepository
, ensuring isolation and enhancing performance.
2. Asynchronous Communication
Utilize asynchronous communication patterns, such as message queues or event sourcing, to decouple services and improve overall system responsiveness. By employing asynchronous communication, services can continue processing requests without waiting for immediate responses, leading to better performance and fault tolerance.
Example:
// Publishing an event using message queue
@Autowired
private MessageQueue messageQueue;
public void publishEvent(Event event) {
messageQueue.publish(event);
}
In this example, the publishEvent
method utilizes a message queue for asynchronous event publishing, allowing the service to continue processing without waiting for the event to be consumed.
3. Caching
Implement caching at various levels, such as application-level caching with tools like Redis or Memcached, to reduce the load on backend services and improve response times for frequently accessed data. By caching data, you can significantly reduce the latency introduced by repeated data retrieval operations.
Example:
// Implementing caching using Redis
@Autowired
private RedisCache redisCache;
public Product getProductById(String productId) {
Product product = redisCache.get(productId);
if (product == null) {
// Fetch product from database
product = productService.getProductById(productId);
// Store product in cache
redisCache.put(productId, product);
}
return product;
}
In the above code snippet, caching is implemented using Redis to store and retrieve the Product entity, reducing the need for repeated database access.
4. Efficient Error Handling
Proper error handling is essential for maintaining performance and reliability in a microservices architecture. Implementing circuit breakers, fallback methods, and graceful degradation strategies can prevent cascading failures and improve overall system responsiveness.
Example:
// Using Hystrix for circuit breaking
@HystrixCommand(fallbackMethod = "fallbackMethod")
public String serviceCall() {
// Service logic
}
public String fallbackMethod() {
// Fallback logic
}
In this example, the @HystrixCommand
annotation is used to handle potential failures and invoke the fallbackMethod
in case of service unavailability, preventing performance degradation.
5. Efficient Service-to-Service Communication
Optimize service-to-service communication by utilizing efficient protocols like gRPC or implementing a reactive communication approach with technologies like Project Reactor. These approaches can significantly reduce latency and overhead in communication between microservices.
Example:
// Implementing service communication with gRPC
ManagedChannel channel = ManagedChannelBuilder.forAddress("service-host", servicePort)
.usePlaintext()
.build();
YourServiceGrpc.YourServiceBlockingStub stub = YourServiceGrpc.newBlockingStub(channel);
YourResponse response = stub.yourMethod(YourRequest.newBuilder().build());
In the example above, gRPC is utilized for efficient and high-performance communication between microservices.
Wrapping Up
Enhancing microservices speed and performance requires a comprehensive approach that encompasses efficient data storage, communication, caching, error handling, and service communication. By implementing these essential performance tips, you can ensure your microservices architecture operates at optimal efficiency, delivering exceptional responsiveness and scalability.
Remember, continuously monitoring and analyzing your microservices' performance is crucial for identifying bottlenecks and further optimizing the system for peak efficiency.
By following these performance tips, you can master the art of maximizing microservices' speed and efficiency, empowering your applications to deliver exceptional user experiences.
We hope these tips help you enhance the performance of your microservices architecture! Happy coding!
To delve deeper into microservices performance optimization, check out Microservices Performance: Best Practices and Pitfalls for additional insights.
Checkout our other articles