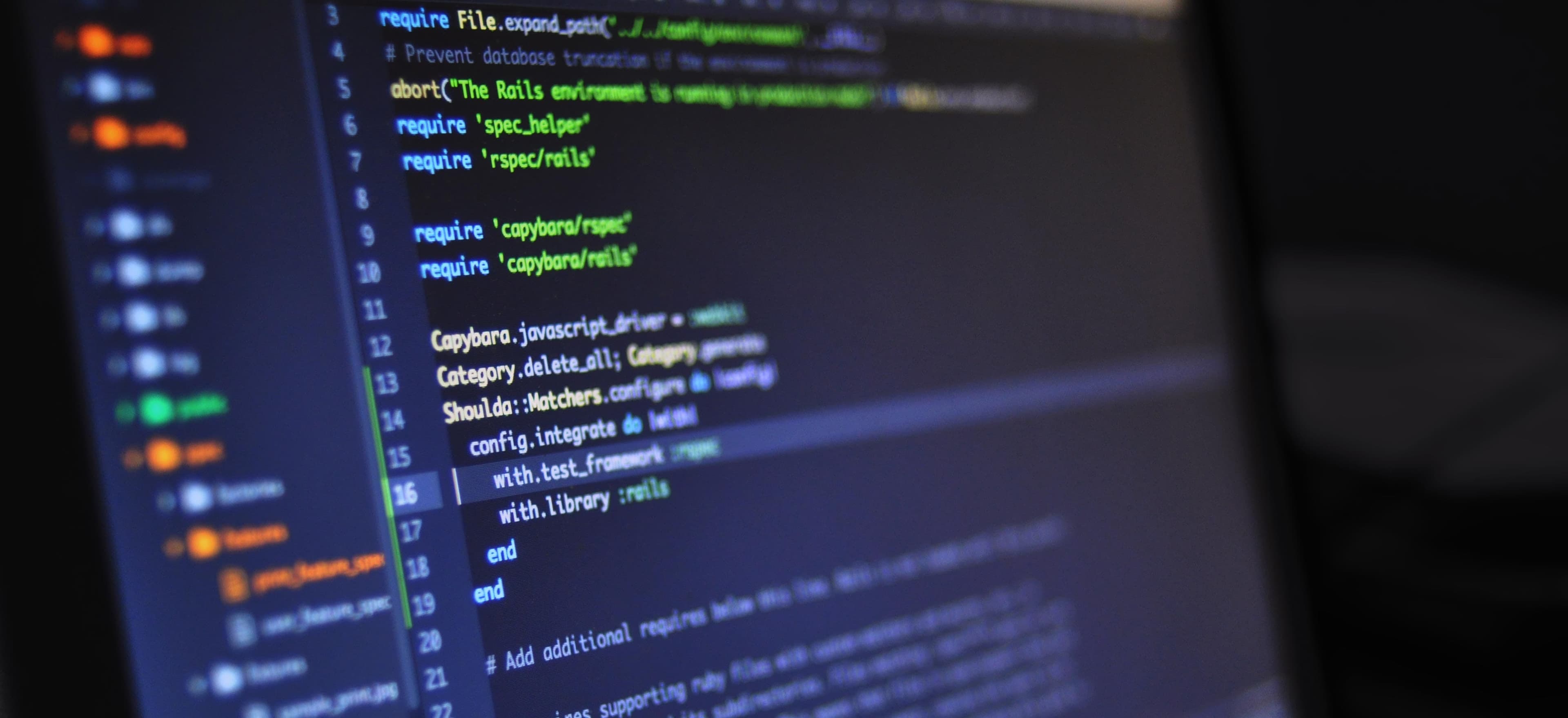
- Published on
Simplifying Security: JPA in Spring MVC with JavaConfig
In the realm of web application development, security and data management are two pillars that uphold the integrity and functionality of a project. Among the myriad of frameworks and technologies that developers have at their disposal, Spring MVC and Java Persistence API (JPA) stand out for their robustness, versatility, and developer-friendly approach. In this blog post, we will explore how to simplify your project's security and enhance its data management by integrating JPA into a Spring MVC application using Java-based Configuration (JavaConfig).
Understanding the Basics
Before diving into the nuts and bolts of integrating JPA and Spring MVC, let's clarify what these technologies are and why they matter.
Spring MVC
Spring MVC is a part of the larger Spring Framework, an open-source application framework for the Java platform that provides comprehensive infrastructure support for developing Java-based applications. Spring MVC enables the easy development of web applications through the Model-View-Controller (MVC) design pattern, benefiting developers with a flexible and decoupled architecture.
Check out the official Spring MVC documentation for an in-depth exploration: Spring MVC Documentation.
Java Persistence API (JPA)
JPA is a Java application programming interface specification that describes the management of relational data in applications using Java Platform, Standard Edition, and Java Platform, Enterprise Edition. JPA allows for a simplified, object-relational mapping to handle data persistence, making it a preferred choice for Java developers working with data-driven applications.
Dig deeper into JPA through the official Java documentation: JPA Overview.
Integrating JPA in Spring MVC with JavaConfig
The integration of JPA into a Spring MVC project leverages JavaConfig, offering a type-safe approach to configure the beans and repositories necessary for your application. This method replaces the traditional XML-based configuration, providing a more modern and straightforward way to set up your project.
Step 1: Set Up Your Project Dependencies
First, you need to include the necessary dependencies in your pom.xml
file if you're using Maven, or in your build.gradle
if you're working with Gradle. Here's a quick snippet for Maven:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
This setup includes Spring Boot's starter for JPA, an in-memory H2 database for demonstration purposes, and the web starter kit.
Step 2: Configure Your DataSource and EntityManager
Using JavaConfig allows you to programmatically define your beans and configurations. In a new configuration class, define your DataSource
, EntityManagerFactory
, and TransactionManager
beans:
import javax.sql.DataSource;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.jdbc.datasource.DriverManagerDataSource;
import org.springframework.orm.jpa.JpaTransactionManager;
import org.springframework.orm.jpa.LocalContainerEntityManagerFactoryBean;
import org.springframework.transaction.PlatformTransactionManager;
@Configuration
public class PersistenceJPAConfig{
@Bean
public LocalContainerEntityManagerFactoryBean entityManagerFactory() {
LocalContainerEntityManagerFactoryBean em = new LocalContainerEntityManagerFactoryBean();
em.setDataSource(dataSource());
em.setPackagesToScan(new String[] { "com.yourpackage.model" });
// Additional JPA properties can be set here
return em;
}
@Bean
public DataSource dataSource(){
DriverManagerDataSource dataSource = new DriverManagerDataSource();
dataSource.setDriverClassName("org.h2.Driver");
dataSource.setUrl("jdbc:h2:mem:testdb");
dataSource.setUsername("sa");
dataSource.setPassword("");
return dataSource;
}
@Bean
public PlatformTransactionManager transactionManager(){
JpaTransactionManager transactionManager = new JpaTransactionManager();
transactionManager.setEntityManagerFactory(entityManagerFactory().getObject());
return transactionManager;
}
}
Step 3: Create Your Entities and Repositories
With your DataSource and EntityManager configured, it's time to define your entities and repositories. Entities in JPA are simple POJOs (Plain Old Java Objects) annotated to map to the database tables.
Here's an example entity:
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
private String name;
private String email;
// Constructor, Getters, and Setters
}
For the repository, you can extend Spring JPA’s JpaRepository
interface:
import org.springframework.data.jpa.repository.JpaRepository;
public interface UserRepository extends JpaRepository<User, Long> {}
Step 4: Develop Your Controllers
Now, structure your MVC by developing controllers to handle user requests and interact with your repositories. For instance:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class UserController {
@Autowired
UserRepository userRepository;
@RequestMapping("/")
public String users(Model model) {
model.addAttribute("users", userRepository.findAll());
return "users";
}
}
Step 5: Secure Your Application
Spring Security can be easily added to manage the security of your web application. Include the Spring Security dependency and configure it using JavaConfig, defining aspects like WebSecurityConfigurerAdapter, UserDetailsService, and PasswordEncoder implementations.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
Security configuration example:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
@Configuration
@EnableWebSecurity
public class WebSecurityConfig { // Your security configurations here
@Bean
public BCryptPasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
This setup establishes a foundation for incorporating JPA into a Spring MVC application while bolstering its security using JavaConfig—a method that not only simplifies the development process but also enhances code clarity and maintainability.
By intelligently combining these powerful Java technologies, you can build highly efficient, secure, and scalable web applications that stand the test of time.
For those eager to explore further, the Spring Security Reference and Java Persistence Tutorial prove invaluable resources in your journey towards mastering Java web application development.
Checkout our other articles