Unveiling Hidden Gems: 10 Java 8 Features You Missed
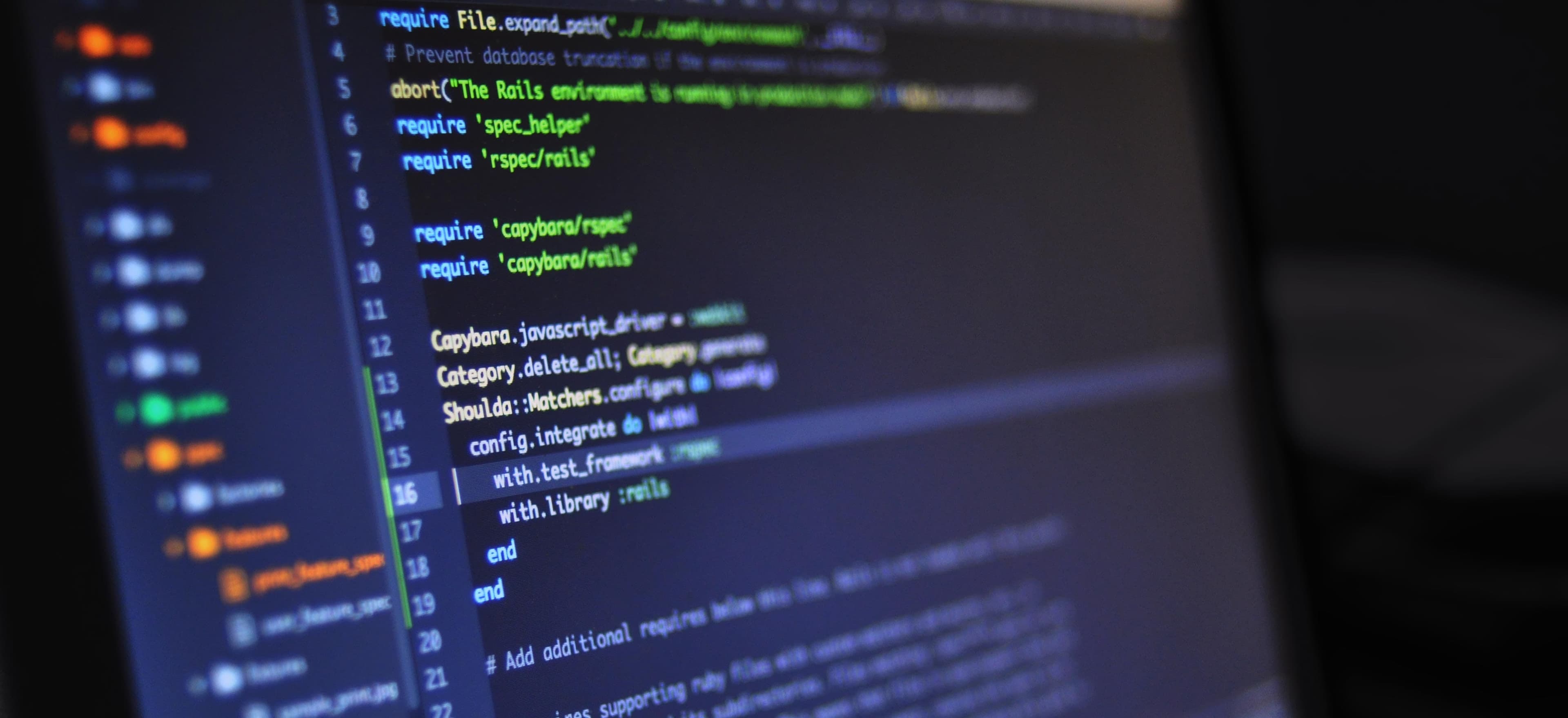
- Published on
Unveiling Hidden Gems: 10 Java 8 Features You Missed
Java 8, released in March 2014, introduced a plethora of new features that revolutionized the way developers write code in Java. While lambdas and streams often steal the spotlight, there are several other hidden gems in Java 8 that are equally powerful but often overlooked.
In this article, we will uncover 10 such hidden gems in Java 8 that you may have missed. These features not only enhance the expressiveness of your code but also improve efficiency and readability. Let's dive in!
1. Optional
The Optional
class, introduced in Java 8, is a container object that may or may not contain a non-null value. It helps in eliminating null pointer exceptions and writing more readable and expressive code by forcing the developer to explicitly handle the case when a value may be absent.
Optional<String> name = Optional.ofNullable(getName());
String defaultValue = name.orElse("default");
Using Optional
encourages developers to handle both cases (presence and absence of value) explicitly, leading to more robust and predictable code.
2. CompletableFuture
CompletableFuture
is a powerful class for asynchronous programming in Java. It enables developers to write non-blocking, asynchronous code in a more intuitive and readable manner compared to traditional approaches using callbacks or raw threads.
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> fetchData());
future.thenApply(data -> processData(data))
.thenAccept(result -> handleResult(result));
It simplifies the handling of asynchronous tasks, making the code cleaner and more maintainable.
3. Date and Time API
Prior to Java 8, working with dates and times in Java was often cumbersome and error-prone. The Date and Time API introduced in Java 8 provides a comprehensive, immutable, and thread-safe API for handling date and time-related operations.
LocalDate today = LocalDate.now();
LocalDate nextWeek = today.plusWeeks(1);
The new API addresses the shortcomings of the legacy java.util.Date
and java.util.Calendar
classes, offering a more intuitive and less error-prone approach to date and time manipulation.
4. Method References
Method references provide a concise way to refer to methods or constructors using a special syntax. They enhance the readability of your code and can be particularly useful when working with lambda expressions.
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(System.out::println);
Using method references not only reduces boilerplate code but also makes the code more expressive and maintainable.
5. Stream API Enhancements
In addition to introducing the Stream API, Java 8 also enhanced it with a variety of useful methods such as takeWhile
, dropWhile
, and ofNullable
. These methods further augment the flexibility and power of stream operations.
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8);
List<Integer> result = numbers.stream()
.takeWhile(n -> n < 5)
.collect(Collectors.toList());
These enhancements make stream operations more versatile and enable developers to write more expressive and concise code.
6. Default Methods in Interfaces
Java 8 introduced the concept of default methods in interfaces, allowing developers to add new methods to interfaces without breaking existing implementations. This feature enables the evolution of interfaces without forcing all implementers to provide an implementation immediately.
public interface Logger {
default void log(String message) {
System.out.println(message);
}
}
Default methods promote backward compatibility and provide a mechanism for extending interfaces without disrupting the classes that implement them.
7. Nashorn JavaScript Engine
Java 8 includes Nashorn, a high-performance JavaScript engine that allows developers to embed JavaScript code within Java applications. This feature facilitates seamless interoperation between Java and JavaScript, opening doors to new possibilities for polyglot programming.
ScriptEngine engine = new ScriptEngineManager().getEngineByName("nashorn");
engine.eval("print('Hello, World!')");
Nashorn provides a lightweight and efficient way to incorporate JavaScript into Java applications, enabling a broader range of programming techniques.
8. Improved Annotations
Java 8 brought enhancements to annotations, including the introduction of repeating annotations and type annotations. Repeating annotations enable the use of multiple annotations of the same type, while type annotations allow annotations to be applied to a wider range of program elements.
@Author(name="Alice")
@Author(name="Bob")
public class MyClass {
@NonNull String data;
}
These improvements provide greater flexibility and expressiveness when using annotations in Java code.
9. Parallel Array Sorting
Java 8 introduced new methods for parallel sorting of arrays using the Arrays
class. These methods leverage the multi-core processors available in modern systems, resulting in faster sorting of large arrays.
int[] numbers = {5, 3, 9, 1, 7};
Arrays.parallelSort(numbers);
Parallel array sorting improves the performance of sorting operations, especially for large datasets.
10. Compact Profiles
Java 8 introduced the concept of compact profiles, which are predefined subsets of the Java platform that are tailored to specific deployment scenarios. These profiles enable the creation of smaller, more efficient Java runtimes for applications with different resource constraints.
java -profile compact1 MyProgram.java
Compact profiles provide a way to optimize the Java runtime for different environments, such as embedded systems or cloud-based applications, without sacrificing essential functionality.
Closing the Chapter
Java 8 brought about a significant evolution in the Java programming language, introducing several features that not only modernized the language but also enhanced developer productivity. While lambdas and streams rightfully garnered attention, the hidden gems discussed in this article offer additional capabilities that can greatly benefit developers.
By leveraging the features such as Optional
, CompletableFuture
, method references, and others, developers can write more expressive, efficient, and maintainable code. Understanding and harnessing these hidden gems can elevate your Java programming skills to the next level, unlocking the full potential of the language.
Don't let these hidden gems remain hidden any longer. Explore, experiment, and integrate them into your Java code to take full advantage of everything Java 8 has to offer. Happy coding!
For more in-depth insights into Java 8 and other Java features, check out Java Magazine and Baeldung.
Checkout our other articles