Challenges of Designing a JVM-Compatible Language
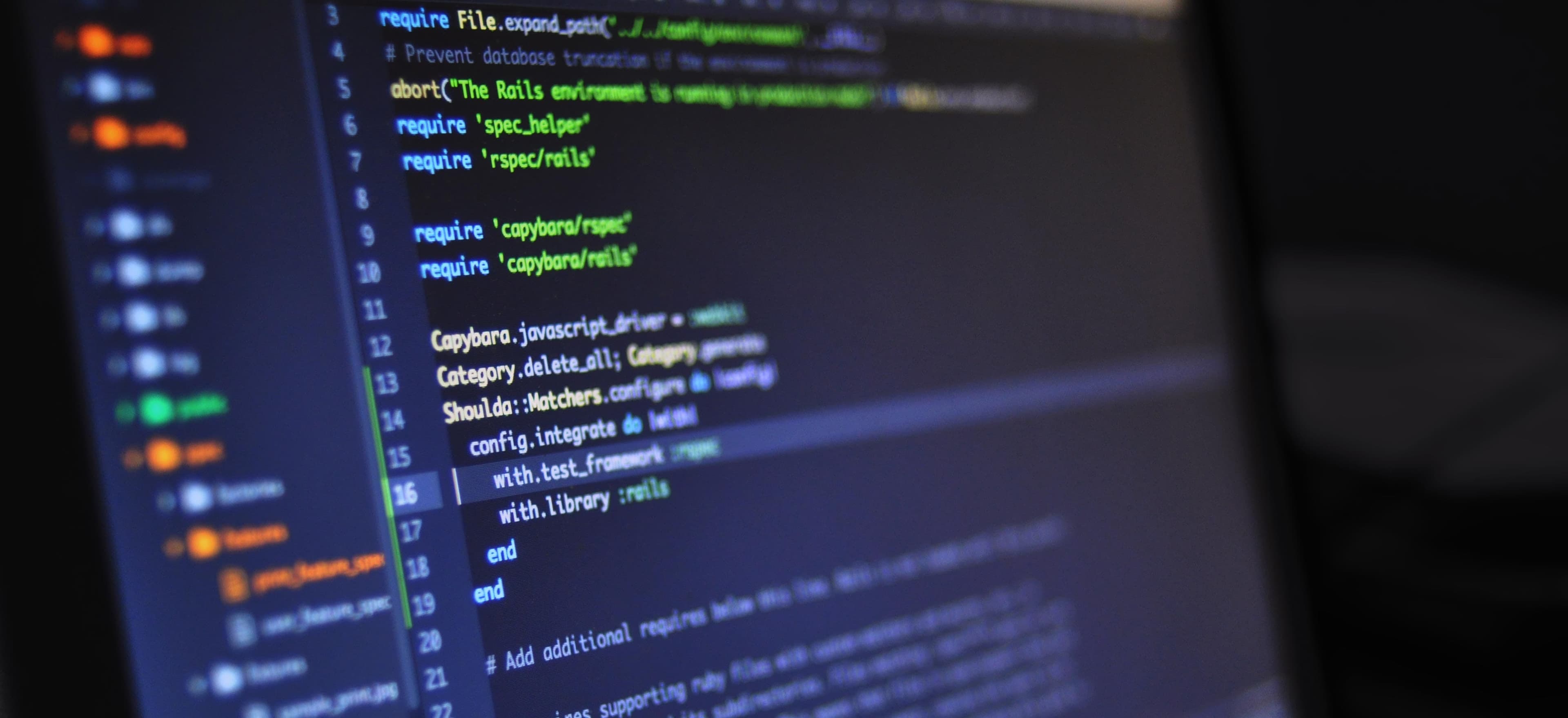
- Published on
Challenges of Designing a JVM-Compatible Language
When it comes to developing a new programming language, targeted for use on the Java Virtual Machine (JVM), various challenges and considerations must be addressed. The JVM ecosystem offers a plethora of benefits, making it an attractive platform for language developers and users alike. However, leveraging the JVM for a new language involves a myriad of challenges that need to be carefully navigated to ensure a successful, efficient, and compatible integration.
In this article, we’ll explore the complexities and obstacles involved in designing a language that seamlessly integrates with the JVM, including considerations such as interoperability, performance, and tooling support.
Interoperability with Java
One of the most significant advantages of developing a language for the JVM is the ability to seamlessly integrate with existing Java code and libraries. Java's extensive ecosystem, including frameworks, libraries, and tools, is a valuable resource for any language targeting the JVM. Therefore, ensuring smooth interoperability with Java is crucial for the success of a new JVM-compatible language.
Java Interoperability Considerations
When designing a JVM-compatible language, it's essential to consider how the language will interact with Java classes and objects. This includes addressing challenges such as:
- Type System Compatibility: Ensuring that the language's type system is interoperable with Java's type system is crucial for seamless interaction between the two languages.
- Exception Handling: Providing mechanisms for handling Java exceptions within the new language and vice versa.
- Integration with Java Libraries: Enabling the use of existing Java libraries seamlessly from the new language, leveraging the rich Java ecosystem.
Example: Interoperability with Java
Let's consider an example of interoperability with Java using the popular JVM language, Kotlin. Kotlin's seamless interoperability with Java is one of its key strengths. Below is a simple Kotlin class that interacts with a Java class:
// Java class
public class JavaClass {
public String getData() {
return "Data from Java";
}
}
// Kotlin class
class KotlinClass {
fun processData(javaClass: JavaClass): String {
return "Kotlin received: ${javaClass.data}"
}
}
In this example, Kotlin seamlessly interacts with a Java class, demonstrating the importance of interoperability when designing a JVM-compatible language.
Performance and Optimization
Efficient performance and optimization are crucial considerations when designing a language for the JVM. The JVM provides a robust runtime environment, complete with automatic memory management, dynamic compilation, and optimization features. However, leveraging these features effectively and optimizing the language's performance on the JVM presents a significant challenge.
Performance Considerations
When developing a JVM-compatible language, performance considerations include:
- Garbage Collection: Designing memory-efficient data structures and managing object lifecycles to minimize the impact on garbage collection.
- Just-In-Time (JIT) Compilation: Utilizing JIT compilation effectively to translate the language's bytecode into efficient native machine code.
- Optimizing for JVM Features: Leveraging JVM-specific features such as virtual method dispatch and dynamic class loading to enhance performance.
Example: Performance Optimization in Scala
Scala, a popular language for the JVM, demonstrates effective performance optimization techniques. By utilizing functional programming constructs and leveraging JVM features such as tail recursion optimization, Scala demonstrates how a language can achieve high performance on the JVM.
// Scala code demonstrating tail recursion optimization
def factorial(n: Int): Int = {
@annotation.tailrec
def loop(acc: Int, n: Int): Int = {
if (n <= 0) acc
else loop(acc * n, n - 1)
}
loop(1, n)
}
In this example, Scala's tail recursion optimization showcases how the language embraces performance considerations specific to the JVM.
Tooling Support and Ecosystem Integration
Another critical aspect of designing a JVM-compatible language is ensuring comprehensive tooling support and seamless integration with the JVM ecosystem. Robust tooling, including compilers, build systems, IDE support, and debugging tools, plays a vital role in the adoption and success of a new language on the JVM.
Tooling and Ecosystem Integration Considerations
Key considerations for tooling support and ecosystem integration include:
- Compiler Integration: Developing a robust compiler that translates the language's source code into JVM bytecode efficiently.
- IDE Support: Providing plugins and extensions for popular IDEs such as IntelliJ IDEA and Eclipse to enable a smooth development experience.
- Build System Integration: Supporting integration with popular build systems like Maven and Gradle to facilitate dependency management and project builds.
Example: Kotlin's Tooling Support
Kotlin's exemplary tooling support, including seamless integration with IntelliJ IDEA and Android Studio, demonstrates the significance of robust tooling for the success of a JVM-compatible language. With first-class IDE support and a dedicated build system (Kotlin Gradle Plugin), Kotlin has successfully integrated into the JVM ecosystem.
Lessons Learned
Designing a language for the JVM presents a unique set of challenges and considerations, including interoperability with Java, performance optimization, and comprehensive tooling support. By carefully addressing these challenges, language designers can develop JVM-compatible languages that seamlessly integrate into the robust JVM ecosystem, ultimately offering developers the benefits of both the language and the JVM platform.
In conclusion, the successful design of a JVM-compatible language requires a deep understanding of JVM internals, Java interoperability, and performance optimization, coupled with extensive tooling support to create a compelling and efficient language for the JVM.
As language designers continue to innovate and address these challenges, the JVM ecosystem will continue to flourish with a diverse range of languages offering unique capabilities while harnessing the power of the JVM.
Whether you're designing a new language for the JVM or exploring the capabilities of existing JVM languages, addressing these challenges is pivotal to ensure a seamless and efficient integration with the Java Virtual Machine.
So, which JVM-compatible language are you most excited about? How do you think it addresses the challenges discussed in this article? Let's continue the conversation!
Checkout our other articles