Avoiding Downtime: Crafting High-Availability Systems
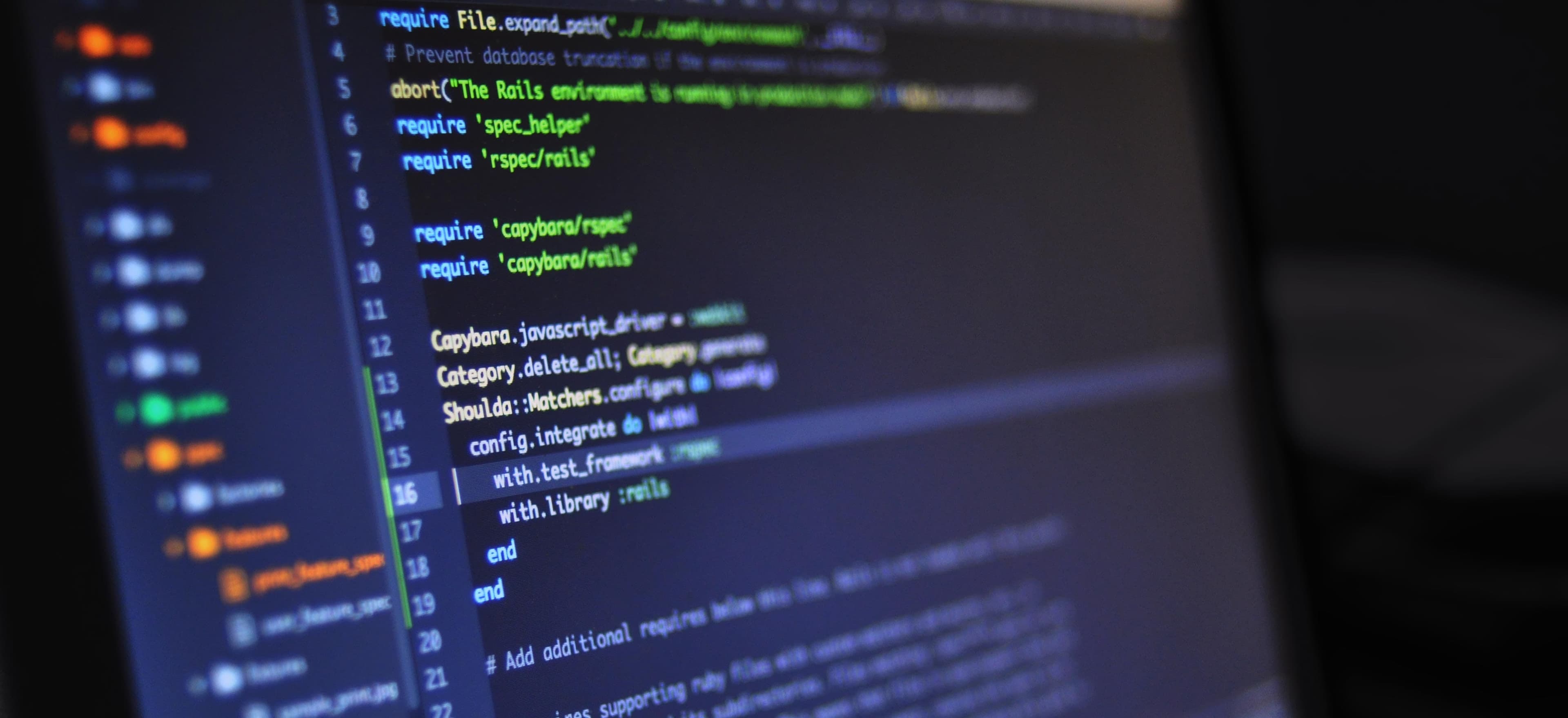
- Published on
The Art of Crafting High-Availability Systems in Java
In today's fast-paced digital world, system downtime is simply not an option. Whether it's a banking application handling thousands of transactions per second or a social media platform with millions of users, ensuring high availability is crucial. In this article, we'll explore how to build high-availability systems in Java, leveraging its robust features and rich ecosystem.
Understanding High-Availability Systems
High availability refers to a system's ability to remain operational and accessible for a high percentage of time. Achieving high availability involves building redundancy, fault tolerance, and automatic failover mechanisms into the system architecture.
Redundancy
Redundancy involves duplicating critical system components to ensure that if one fails, another can seamlessly take over. In Java, this can be achieved through clustering and load balancing. Apache ZooKeeper is a popular choice for coordinating and managing distributed systems, providing a high-availability output.
Fault Tolerance
Fault tolerance is the system's ability to continue operating properly in the event of the failure of some of its components. In Java, this can be achieved through frameworks like Hystrix, which provides latency and fault tolerance.
Automatic Failover
Automatic failover allows a standby server to take over when the primary server fails. Implementing this in Java can be done using tools like Java Database Connectivity (JDBC) and Java Messaging Service (JMS).
Now that we understand the key concepts, let's dive into the implementation details.
Implementing High Availability in Java
1. Use of Load Balancing
In a high-availability system, distributing incoming network traffic across multiple servers is crucial. This not only enhances performance but also ensures that if one server fails, the others can handle the load.
In Java, this can be achieved using a robust framework such as Spring Cloud Netflix that provides built-in support for client-side load balancing. Here's a snippet demonstrating the use of Ribbon, a client-side load balancer integrated with Spring Cloud:
@FeignClient(name = "service-name", configuration = FooConfiguration.class)
@RibbonClient(name = "service-name")
public interface MyFeignClient {
// REST endpoints
}
In this example, @FeignClient
creates a load-balanced client, and @RibbonClient
specifies the ribbon configuration for the targeted service.
2. Implementing Circuit Breaker Pattern
The Circuit Breaker pattern is essential for preventing a network or service failure from cascading to other services. In Java, the renowned library Resilience4j provides a fault tolerance library, integrating seamlessly with Spring Boot.
Here's an example of using Resilience4j to create a circuit breaker:
CircuitBreaker circuitBreaker = CircuitBreaker.ofDefaults("backendService");
Supplier<String> decoratedSupplier = CircuitBreaker
.decorateSupplier(circuitBreaker, backendService::doSomething);
String result = Try.ofSupplier(decoratedSupplier)
.recover(throwable -> "Hello from Recovery").get();
This circuit breaker protects the backendService
invocation, and the system can failover gracefully without affecting the overall stability.
3. Database Replication and Failover
Database availability is critical for any high-availability system. Implementing database replication and failover strategies is essential.
In Java, using Spring Data with tools like MongoDB or MySQL makes achieving database replication and failover seamless. This ensures that even if one database instance goes down, the system can seamlessly switch to another available instance.
4. Implementing Queues for Asynchronous Processing
Another critical aspect of building high-availability systems is handling asynchronous processing. By using a message queue system like RabbitMQ or Apache Kafka, Java applications can offload time-consuming tasks to be processed asynchronously, ensuring smooth system operation.
Utilizing a library such as Spring AMQP for RabbitMQ integration simplifies the implementation and ensures reliability.
Testing and Monitoring
Building high-availability systems is only half the battle; it's crucial to continuously test and monitor the system's performance and resilience.
1. Unit Testing
Performing unit tests using frameworks like JUnit ensures that individual components behave as expected, even under failure scenarios.
@Test
public void testHighAvailabilityBehavior() {
// Test high-availability scenarios
// Assert expected behavior
}
2. Integration Testing
Integration tests, particularly in a distributed environment, are crucial to validate the system's behavior under various failure scenarios.
3. Monitoring with Prometheus and Grafana
Implementing a robust monitoring system using Prometheus for metrics collection and Grafana for visualization provides insights into the system's behavior. Integrating these tools with Java applications ensures proactive detection and resolution of potential issues.
In Conclusion, Here is What Matters
Crafting high-availability systems in Java demands a multi-faceted approach, consisting of redundancy, fault tolerance, automatic failover mechanisms, and robust testing and monitoring strategies. By leveraging Java's rich ecosystem and integrating with renowned frameworks and libraries, developers can build and maintain resilient systems capable of withstanding the challenges of the digital landscape.
Regardless of the scale or complexity of the system, prioritizing high availability is a non-negotiable aspect of modern software engineering. Through strategic architecture and the right tools, Java empowers developers to architect systems that not only remain highly available but also deliver exceptional performance and reliability.