Developing RESTful APIs with Spring MVC: Best Practices
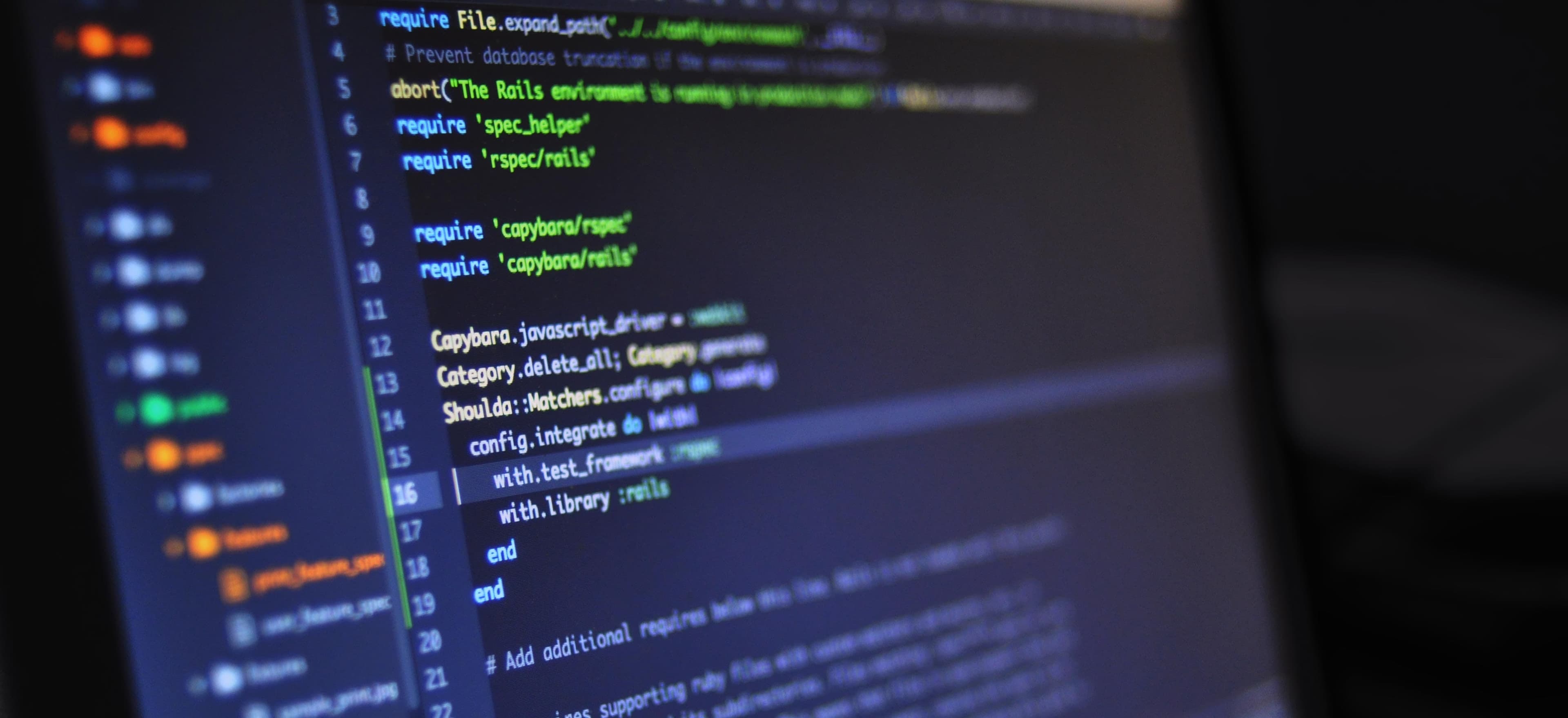
- Published on
Developing RESTful APIs with Spring MVC: Best Practices
When it comes to building robust and scalable APIs, Spring MVC has become a popular choice among Java developers. With its powerful features and flexibility, Spring MVC provides an ideal framework for creating RESTful APIs. In this article, we will explore best practices for developing RESTful APIs with Spring MVC, covering everything from setting up the project to implementing best practices in code.
Setting Up the Project
Before diving into the implementation details, it's essential to set up the project structure and dependencies correctly. To begin, create a new Spring Boot application using either Spring Initializr or your preferred IDE. Here's a simple example of how to set up a basic Spring Boot application for building RESTful APIs:
@SpringBootApplication
public class RestfulApiApplication {
public static void main(String[] args) {
SpringApplication.run(RestfulApiApplication.class, args);
}
}
Ensure that you include the spring-boot-starter-web
dependency in your pom.xml
or build.gradle
file to enable Spring MVC for handling web requests.
Implementing RESTful Endpoints
Once the project is set up, the next step is to implement RESTful endpoints using Spring MVC. When defining endpoints, it's important to adhere to RESTful principles and best practices for a clear and consistent API design. Here's an example of creating a simple RESTful endpoint for retrieving a list of items:
@RestController
@RequestMapping("/items")
public class ItemController {
@Autowired
private ItemService itemService;
@GetMapping
public ResponseEntity<List<Item>> getAllItems() {
List<Item> items = itemService.getAllItems();
return ResponseEntity.ok(items);
}
// Other CRUD endpoints (POST, PUT, DELETE) can be added here
}
In this example, the @RestController
annotation marks the ItemController
as a controller that handles RESTful requests. The @RequestMapping
annotation specifies the base path for all endpoints in the controller. The @GetMapping
annotation defines a GET endpoint for retrieving all items, returning a list of items wrapped in a ResponseEntity
.
Handling Request and Response Bodies
When dealing with complex objects or data, it's crucial to handle request and response bodies properly. Spring MVC provides convenient ways to handle request and response bodies using Jackson for JSON serialization and deserialization. Here's how to handle request and response bodies in a RESTful endpoint:
@RestController
@RequestMapping("/items")
public class ItemController {
// ...
@PostMapping
public ResponseEntity<Item> createItem(@RequestBody Item newItem) {
Item createdItem = itemService.createItem(newItem);
return ResponseEntity.status(HttpStatus.CREATED).body(createdItem);
}
}
In this example, the @PostMapping
annotation defines a POST endpoint for creating a new item. The @RequestBody
annotation binds the request body to the newItem
parameter, allowing for seamless deserialization of the incoming JSON data into a Java object. The method then returns a ResponseEntity
with the created item and a 201 Created
status code.
Error Handling and Exception Management
Effective error handling is essential for any RESTful API to provide meaningful feedback to clients. Spring MVC offers various ways to handle errors and exceptions, ensuring a consistent approach across the API. One of the best practices is to use @ControllerAdvice
to centralize exception handling. Here's how to create a global exception handler using @ControllerAdvice
:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ItemNotFoundException.class)
public ResponseEntity<String> handleItemNotFoundException(ItemNotFoundException ex) {
return ResponseEntity.status(HttpStatus.NOT_FOUND).body(ex.getMessage());
}
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleGenericException(Exception ex) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body("An unexpected error occurred");
}
}
In this example, the @ControllerAdvice
annotation marks the GlobalExceptionHandler
as a global exception handler. The @ExceptionHandler
annotation is used to define specific exception handlers, such as ItemNotFoundException
, with custom error responses. Additionally, a generic exception handler is implemented to catch any unexpected errors and return a generic error message.
Versioning and Media Types
As APIs evolve, maintaining backward compatibility while introducing new features is crucial. Versioning RESTful APIs allows for changes without breaking existing clients. One common approach to API versioning is using URI versioning or custom request headers. Here's an example of versioning a RESTful endpoint using custom media types:
@RestController
@RequestMapping(value = "/items", headers = "Accept=application/vnd.company.v1+json")
public class ItemControllerV1 {
// Endpoint logic for version 1
}
In this example, the @RequestMapping
annotation specifies the custom media type application/vnd.company.v1+json
as the version identifier for the ItemControllerV1
. By using custom media types, API versioning can be achieved without altering the URI structure, providing a clear and flexible versioning approach.
Testing RESTful Endpoints
Testing is an integral part of developing RESTful APIs to ensure that endpoints behave as expected. Spring MVC provides robust support for testing RESTful APIs using tools like JUnit and MockMvc. Here's a simple example of testing a RESTful endpoint with JUnit and MockMvc:
@RunWith(SpringRunner.class)
@WebMvcTest(ItemController.class)
public class ItemControllerTests {
@Autowired
private MockMvc mockMvc;
@MockBean
private ItemService itemService;
@Test
public void testGetAllItems() throws Exception {
List<Item> items = Arrays.asList(new Item("Item 1"), new Item("Item 2"));
when(itemService.getAllItems()).thenReturn(items);
mockMvc.perform(get("/items"))
.andExpect(status().isOk())
.andExpect(content().json("[{'name':'Item 1'},{'name':'Item 2'}]"));
}
// Additional tests for other endpoints can be added here
}
In this example, the @WebMvcTest
annotation is used to focus testing on the ItemController
and its related components. The MockMvc
instance is used to perform HTTP requests and assert the expected behavior of the RESTful endpoints using MockMvcResultMatchers
.
Closing the Chapter
Developing RESTful APIs with Spring MVC involves adhering to best practices for creating a robust and maintainable API. By following the guidelines outlined in this article, Java developers can build RESTful APIs that are efficient, well-designed, and easy to maintain. From setting up the project to handling request and response bodies, error handling, versioning, and testing, Spring MVC offers a comprehensive toolkit for building high-quality RESTful APIs in Java.
In conclusion, leveraging the powerful features of Spring MVC combined with best practices leads to the creation of RESTful APIs that meet the demands of modern web applications while delivering an exceptional developer and user experience.
By employing these best practices, developers can ensure that their Spring MVC-based RESTful APIs are not only functional and reliable but also scalable and easily maintainable for future enhancements and iterations. With a solid foundation in place, the potential for growth and expansion is substantially increased, which is a vital consideration in today's ever-evolving technological landscape.
Development of RESTful APIs with Spring MVC is an investment in the future, as it contributes to the creation of a flexible and resilient ecosystem where the seamless integration of new features and technologies becomes a natural and straightforward process.
And this is the beauty of Spring MVC—a framework that empowers developers to build RESTful APIs that not only meet but exceed the demands of modern web development, ensuring a smooth and efficient interaction between the backend and frontend components, and ultimately delivering unparalleled user experiences. So, why not give Spring MVC a try for your next RESTful API project?
Remember, embracing best practices in RESTful API development with Spring MVC today sets the stage for a future-ready, scalable, and adaptable system that stands the test of time.
In this article, we explored the best practices for developing RESTful APIs with Spring MVC, covering essential aspects like project setup, endpoint implementation, request and response handling, error management, versioning, and testing. By following these best practices, Java developers can create high-quality, scalable, and maintainable RESTful APIs with Spring MVC. If you're interested in learning more about Spring MVC, be sure to check out the official Spring documentation for in-depth insights and guidance on building web applications.
Checkout our other articles