Breaking the Chain: Overcoming Common Pitfalls in Chain of Responsibility
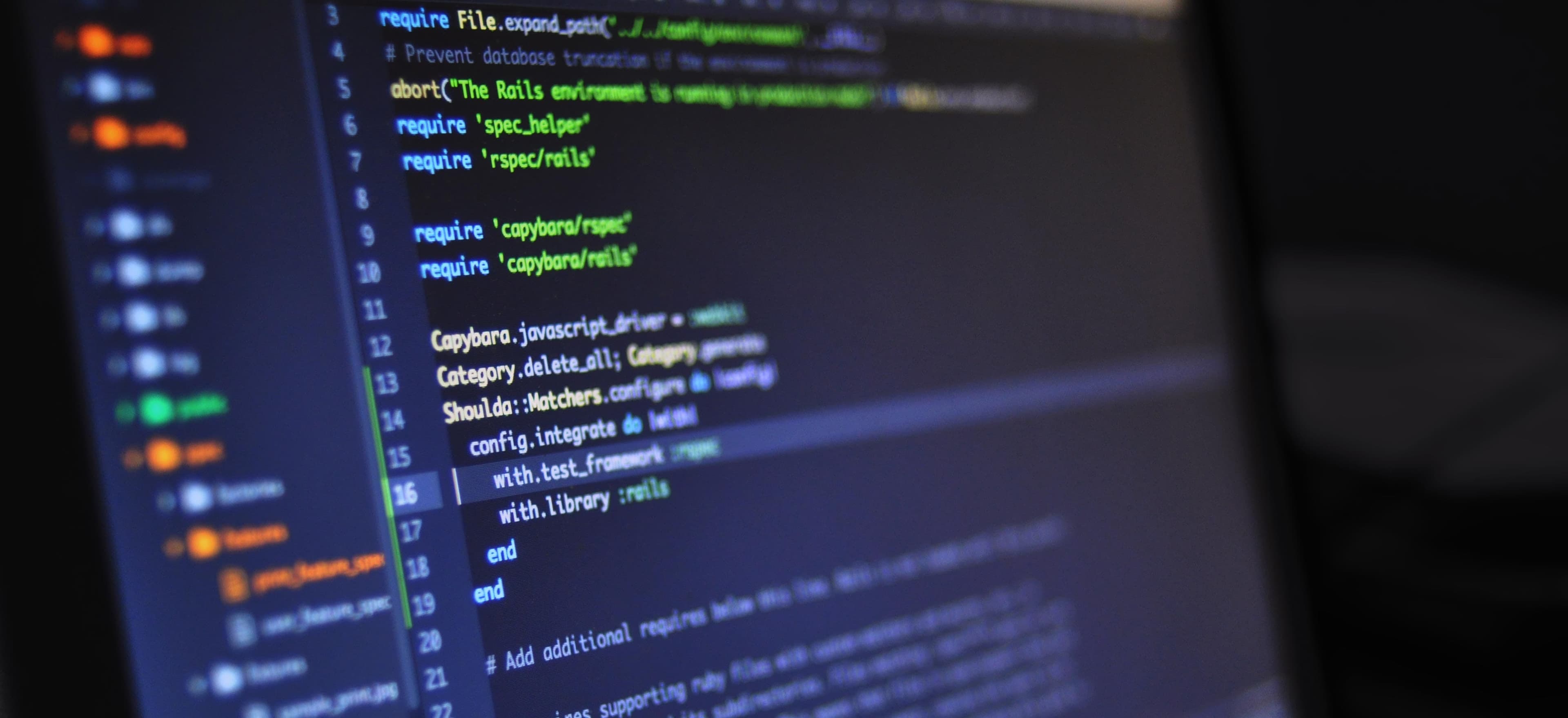
- Published on
Breaking the Chain: Overcoming Common Pitfalls in Chain of Responsibility
In Java development, the Chain of Responsibility pattern provides a way to pass a request along a chain of handlers. Each handler decides either to process the request or pass it to the next handler in the chain. While this pattern offers flexibility and decoupling, it can also lead to common pitfalls if not implemented carefully.
Understanding the Chain of Responsibility Pattern
The Chain of Responsibility pattern is a behavioral design pattern where a request is passed along a chain of handlers, and each handler has the opportunity to process the request or pass it along to the next handler in the chain. This pattern promotes loose coupling between the sender of a request and its receivers.
In Java, the Chain of Responsibility pattern is often used in scenarios where the processing of a request can be handled by multiple objects, and the exact processing object is determined dynamically at runtime.
Example Use Case
Consider a scenario where a user's request needs to be handled by multiple layers of authentication and authorization. The request is passed from one layer to another until it is successfully authenticated and authorized. Each layer represents a handler in the chain, and the request is passed along until it is effectively handled.
Common Pitfalls in Implementing the Chain of Responsibility Pattern
While the Chain of Responsibility pattern offers advantages in terms of flexibility and extensibility, it can also lead to common pitfalls if not implemented carefully. Let's explore some of these pitfalls and how they can be overcome.
1. Unclear Handler Responsibilities
One common pitfall is the ambiguity or overlap of handler responsibilities within the chain. It's crucial to define clear and specific responsibilities for each handler to avoid confusion and potential conflicts in handling requests.
2. Lack of Termination Condition
Another pitfall is the lack of a termination condition in the chain, leading to the possibility of an endless loop as the request keeps being passed along without being effectively handled. Implementing a clear termination condition is essential to prevent infinite loops in the chain.
3. Tight Coupling Between Handlers
If the handlers in the chain are tightly coupled, it can lead to difficulties in extending or modifying the chain. Loose coupling between handlers is essential to allow for easy modifications and additions to the chain.
Overcoming Common Pitfalls
Now that we've identified some common pitfalls in implementing the Chain of Responsibility pattern, let's delve into strategies for overcoming these challenges effectively.
1. Clear Handler Responsibilities
To address the issue of unclear handler responsibilities, it's important to define a specific and unambiguous set of responsibilities for each handler in the chain. Each handler should have a single responsibility and a clear understanding of when to process a request or pass it along.
public abstract class Handler {
protected Handler successor;
public void setSuccessor(Handler successor) {
this.successor = successor;
}
public abstract void handleRequest(Request request);
}
In the above example, the Handler
class defines the common structure for all handlers in the chain. Each concrete handler class will implement the handleRequest
method to fulfill its specific responsibility, ensuring a clear division of responsibilities within the chain.
2. Implementing a Termination Condition
To prevent the chain from entering an endless loop, it's crucial to implement a termination condition that dictates when the request should stop propagating through the chain. This termination condition can be based on specific criteria or the state of the request itself.
public class ConcreteHandler1 extends Handler {
public void handleRequest(Request request) {
if (/* check if request should be handled by this handler */) {
// Process the request
} else if (successor != null) {
successor.handleRequest(request);
}
}
}
In the ConcreteHandler1
class, the termination condition is checked before passing the request to the next handler. If the condition is not met, the request will not propagate further, effectively terminating the chain.
3. Promoting Loose Coupling Between Handlers
To ensure loose coupling between handlers, it's beneficial to employ a mechanism that allows for dynamic changes to the chain without directly modifying the existing handlers. This can be achieved through the use of a dynamic mechanism for adding, removing, or reordering handlers in the chain.
public class Chain {
private Handler firstHandler;
public void addHandler(Handler handler) {
if (firstHandler == null) {
firstHandler = handler;
} else {
Handler current = firstHandler;
while (current.successor != null) {
current = current.successor;
}
current.successor = handler;
}
}
public void handleRequest(Request request) {
if (firstHandler != null) {
firstHandler.handleRequest(request);
}
}
}
In the Chain
class, a dynamic mechanism for adding handlers to the chain is implemented, promoting loose coupling and allowing for easy modifications and extensions to the chain without directly altering the existing handlers.
Wrapping Up
The Chain of Responsibility pattern is a powerful tool for decoupling the sender of a request from its receivers and promoting flexibility in handling requests. However, it's essential to be mindful of common pitfalls that can arise during its implementation.
By acknowledging and addressing the potential pitfalls such as unclear handler responsibilities, lack of termination condition, and tight coupling between handlers, developers can harness the full potential of the Chain of Responsibility pattern while mitigating its associated challenges.
In conclusion, a well-implemented Chain of Responsibility pattern can contribute to a more flexible, maintainable, and extensible codebase, empowering developers to effectively handle complex request processing scenarios in Java applications.
Remember to stay informed about the best practices and latest developments in Java design patterns to enhance your software development skills. If you're keen on diving deeper into Java design patterns, you can explore Bryan Hansen's article for further insights.
Keep coding and breaking those chains of responsibility!