Unraveling the Mystery: Effective Post-Hoc Tracing
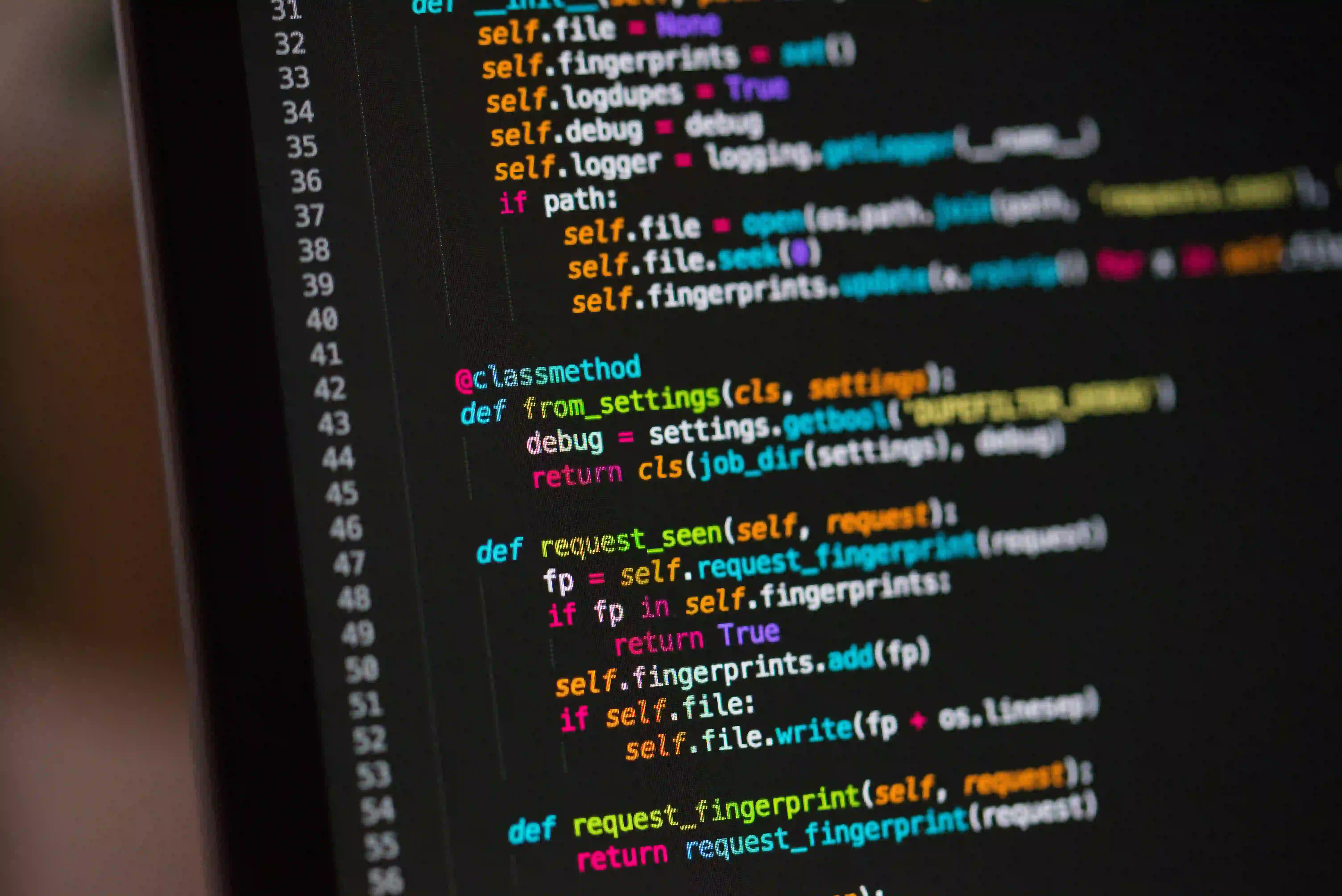
Unraveling the Mystery: Effective Post-Hoc Tracing in Java
Debugging and tracing Java applications can be an arduous and complex task. Developers often find themselves facing challenges in identifying and diagnosing issues that occur during the execution of their applications. Traditional debugging methods focus on real-time monitoring and often fall short when it comes to understanding the intricate details of an application’s behavior in a production environment. This is where post-hoc tracing comes into play, providing a powerful approach to diagnosing issues, identifying performance bottlenecks, and understanding system behavior after the application has been executed.
Understanding Post-Hoc Tracing
What is Post-Hoc Tracing?
Post-hoc tracing is a method that allows developers to analyze and trace the behavior of an application after its execution. Unlike real-time monitoring tools that track an application's behavior as it runs, post-hoc tracing captures detailed information about the application's execution and performance, enabling developers to gain insights into the system's behavior and performance in production.
Post-hoc tracing involves collecting data related to the application's execution, such as method invocations, resource usage, and network calls, and then analyzing this data to understand the application's behavior. This approach is particularly valuable in diagnosing complex bugs, identifying performance bottlenecks, and understanding the system's behavior under real-world conditions.
Benefits of Post-Hoc Tracing
Post-hoc tracing offers several advantages over real-time monitoring and traditional debugging methods.
Minimal Performance Overhead
Real-time monitoring tools often introduce a significant performance overhead, impacting the application's performance. In contrast, post-hoc tracing minimizes the impact on the running application by collecting data after the execution has completed.
Analysis of Production Data
Post-hoc tracing allows developers to analyze production data, providing insights into how the application behaves under real-world conditions. This can be particularly valuable in identifying issues that only manifest in production environments.
Detailed Execution Context
By capturing detailed information about the application's execution, post-hoc tracing provides a comprehensive view of the system's behavior. This level of insight can be crucial in diagnosing complex issues and understanding the application's behavior in different scenarios.
Implementing Post-Hoc Tracing in Java
Tools and Libraries
Several Java tools and libraries are available for implementing post-hoc tracing in applications. Some popular choices include Zipkin, Jaeger, and custom implementations using Application Performance Monitoring (APM) tools such as New Relic and Datadog.
-
Zipkin: This open-source distributed tracing system allows developers to gather timing data for requests across distributed systems. It provides features for performance troubleshooting and monitoring.
-
Jaeger: Another open-source tracing system that is compatible with Zipkin. Jaeger offers features for distributed context propagation, distributed transaction monitoring, and root cause analysis.
-
Custom Implementations with APM Tools: Many APM tools provide custom implementations for post-hoc tracing, allowing developers to integrate tracing capabilities seamlessly into their applications and leverage existing monitoring infrastructure.
Step-by-Step Guide to Setting Up
Implementing post-hoc tracing in a Java application involves several key steps:
-
Choose the Right Tool: Select a post-hoc tracing tool that best fits the requirements of the application. Consider factors such as compatibility, features, and ease of integration.
-
Integrate the Tracing Library: Integrate the chosen tracing library into the Java application. This usually involves adding the library as a dependency and configuring it to capture the required tracing data.
-
Configure Data Collection: Configure the tracing library to collect relevant data points, such as method invocations, database queries, and network requests. This ensures that the tracing data provides a comprehensive view of the application's behavior.
Example Code Snippet
// Example code snippet demonstrating the setup of a basic tracer using Zipkin
import brave.Tracing;
import brave.okhttp3.TracingInterceptor;
import okhttp3.OkHttpClient;
import zipkin2.reporter.AsyncReporter;
import zipkin2.reporter.okhttp3.OkHttpSender;
public class TracingSetup {
public static void main(String[] args) {
// Create and configure a Zipkin sender
OkHttpSender sender = OkHttpSender.create("http://localhost:9411/api/v2/spans");
// Create and configure a Zipkin reporter
AsyncReporter<zipkin2.Span> reporter = AsyncReporter.create(sender);
// Create and configure a Zipkin tracer
Tracing tracing = Tracing.newBuilder()
.localServiceName("my-service")
.spanReporter(reporter)
.build();
// Create an OkHttp client with tracing interceptor
OkHttpClient client = new OkHttpClient.Builder()
.addInterceptor(TracingInterceptor.create(tracing))
.build();
// Use the OkHttpClient for making requests
// ...
}
}
Tips and Best Practices
When implementing post-hoc tracing in Java applications, it's essential to consider the following tips and best practices:
-
Selecting Relevant Data Points: Choose data points that are essential for understanding the application's behavior and diagnosing potential issues. Collecting too much data can lead to information overload, while collecting too little might result in missing crucial insights.
-
Ensuring Data Accuracy: Verify that the tracing data accurately represents the application's behavior. Inaccurate or incomplete data can lead to incorrect conclusions when diagnosing issues.
-
Managing Overhead: Monitor and manage the overhead introduced by post-hoc tracing. While it minimizes impact during application execution, tracing still incurs some overhead, especially when collecting detailed data.
Use Cases and Real-World Examples
Post-hoc tracing has proven particularly useful in various use cases, demonstrating its value in diagnosing complex issues and understanding an application's behavior under real-world conditions.
Diagnosing Unreplicable Bugs
In scenarios where bugs cannot be replicated in a test environment, post-hoc tracing can provide invaluable insights into the application's behavior and state at the time of the issue. This can significantly expedite the debugging process and aid in identifying the root cause of the problem.
Performance Optimization
For applications experiencing performance issues in production, post-hoc tracing allows developers to analyze the actual execution path and identify performance bottlenecks. This can lead to targeted optimizations and improvements without impacting the running application.
Security Vulnerability Tracking
In the event of a security breach or vulnerability, post-hoc tracing can help identify the sequence of events leading to the issue, providing crucial information for forensic analysis and remediation.
Challenges and Limitations
While post-hoc tracing offers valuable insights, it also presents certain challenges and limitations that developers should consider:
-
Data Privacy Concerns: Tracing data may contain sensitive information, raising concerns about data privacy and compliance with regulations such as GDPR and HIPAA. It's essential to handle tracing data responsibly and securely.
-
Managing Tracing Data Volume: Collecting and storing tracing data from production environments can result in large volumes of data. Managing and analyzing this data effectively can be a significant challenge, especially in distributed systems.
-
Impact on Application Performance: Although post-hoc tracing minimizes performance overhead during execution, the collection and analysis of tracing data can still impact application performance, particularly in high-throughput environments.
Closing the Chapter
Effective post-hoc tracing in Java applications provides developers with a powerful tool for diagnosing issues, understanding system behavior, and optimizing performance. By capturing detailed information about an application's execution and performance, post-hoc tracing enables developers to gain insights that are crucial for maintaining and optimizing Java applications in production environments. As the complexity of modern applications continues to grow, post-hoc tracing emerges as an essential component of the developer's toolkit, offering a deeper understanding of application behavior under real-world conditions.
Implementing post-hoc tracing in Java applications requires careful consideration of tools, data collection strategies, and best practices to ensure effective and efficient tracing. By embracing post-hoc tracing, developers can uncover valuable insights into their applications' behavior and drive improvements that enhance performance, reliability, and security.
Further Reading and Resources
For further information on post-hoc tracing and its implementation in Java, consider exploring the following resources:
- Zipkin - Official documentation and tutorials for implementing tracing with Zipkin in Java applications.
- Jaeger - Resources and community forums for implementing distributed tracing using Jaeger in Java applications.
- Brave - GitHub repository for the Brave library, offering extensive documentation and examples for integrating tracing in Java applications.
By delving deeper into post-hoc tracing and leveraging its capabilities in Java development, developers can enhance their ability to diagnose, optimize, and maintain complex applications in production environments.