Maximizing Efficiency: Overcoming Lean Learning Board Hurdles
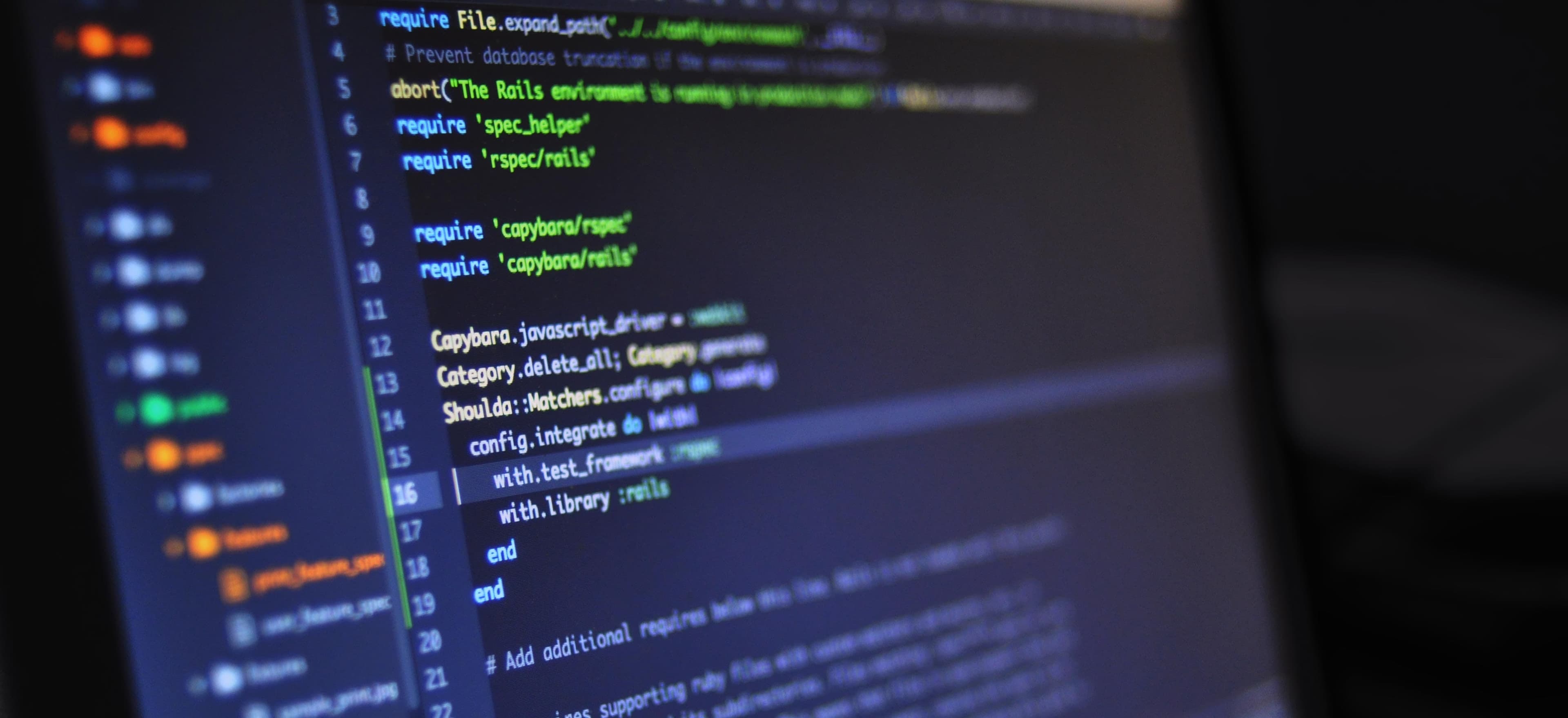
- Published on
Maximizing Efficiency: Overcoming Lean Learning Board Hurdles
In the world of software development, Java remains a widely-used and versatile programming language. Whether you're a seasoned developer or just starting out, optimizing your Java code is crucial for enhancing performance and maintaining clean, manageable code. Today, we'll delve into some valuable tips for maximizing efficiency and overcoming common hurdles when working with Java.
Embracing Object-Oriented Principles
Java, renowned for its object-oriented approach, empowers developers to create modular, maintainable code. By adhering to principles like encapsulation, inheritance, and polymorphism, you can build robust and extensible systems.
Leveraging Encapsulation for Code Modularity
Encapsulation involves bundling data and methods that operate on the data within a single unit, thus restricting access and preventing unintended interference. Let's consider an example of encapsulation in action:
public class Car {
private String model;
public String getModel() {
return model;
}
public void setModel(String model) {
this.model = model;
}
}
In this snippet, the model
attribute is encapsulated within the Car
class, and its access is restricted to only relevant methods. This fosters modularity and prevents external code from directly manipulating the model
attribute.
Harnessing Inheritance for Code Reusability
Inheritance facilitates the creation of new classes that inherit properties and behaviors from existing classes. Here's a succinct example:
public class Vehicle {
protected String brand;
}
public class Car extends Vehicle {
private int numberOfSeats;
}
In this scenario, the Car
class inherits the brand
attribute from the Vehicle
class, promoting code reusability and fostering a hierarchical structure.
Unleashing Polymorphism for Flexibility
Polymorphism permits objects to be treated as instances of their parent class, enabling flexibility and extensibility. Consider a simple demonstration:
public class Shape {
public void draw() {
System.out.println("Drawing a shape");
}
}
public class Circle extends Shape {
public void draw() {
System.out.println("Drawing a circle");
}
}
public class Square extends Shape {
public void draw() {
System.out.println("Drawing a square");
}
}
In this context, invoking the draw
method on a Shape
object could yield varying behaviors, based on the actual instance it represents.
Embracing Efficient Data Structures
Efficient data structures are pivotal for optimized Java code. Utilizing the appropriate data structures, such as ArrayList
, HashMap
, and LinkedList
, can significantly enhance performance.
Opting for the Right Data Structure
When choosing a data structure, it's essential to consider factors like access patterns, insertion and deletion requirements, and memory constraints. For instance, if rapid access to elements is crucial, HashMap
can be a preferred choice due to its constant-time complexity for retrieval operations.
Let's illustrate this with a concise example:
Map<String, Integer> studentScores = new HashMap<>();
studentScores.put("Alice", 95);
studentScores.put("Bob", 87);
int aliceScore = studentScores.get("Alice");
Here, the HashMap
efficiently facilitates retrieving scores with stellar performance.
Unearthing Java Stream API Elegance
Java Stream API, introduced in Java 8, furnishes a powerful and expressive mechanism for processing collections of objects. Leveraging streams and their associated operations can lead to concise and efficient code.
List<String> fruits = Arrays.asList("Apple", "Banana", "Orange", "Mango");
long count = fruits.stream()
.filter(fruit -> fruit.startsWith("A"))
.count();
System.out.println("Fruits starting with 'A': " + count);
In this snippet, the stream facilitates seamless filtering and counting of fruits starting with the letter 'A', showcasing the elegance and succinctness of the Stream API.
Tackling Thread Safety with Synchronization
In a multi-threaded environment, ensuring thread safety is paramount to prevent race conditions and data inconsistencies. Employing synchronization where necessary can mitigate such issues.
Employing Synchronized Methods
Synchronized methods can safeguard critical sections of code by allowing only one thread to execute them at a time. Consider the following:
public class Counter {
private int count;
public synchronized void increment() {
count++;
}
}
By synchronizing the increment
method, concurrent invocations are effectively serialized, averting potential data corruption.
Employing Effective Exception Handling
A robust error-handling strategy is indispensable in Java development to bolster code reliability. Effective exception handling ensures graceful failure and facilitates debugging.
Utilizing Exception Propagation
Throwing and propagating custom exceptions can convey meaningful information and streamline error handling. Let's glance at an instance:
public class AgeValidator {
public void validateAge(int age) throws InvalidAgeException {
if (age < 0) {
throw new InvalidAgeException("Age cannot be negative");
}
}
}
In the above example, the validateAge
method propagates an InvalidAgeException
if an invalid age is encountered, permitting the calling code to handle the exception appropriately.
Closing the Chapter
In conclusion, Java's penchant for object-oriented design, efficient data structures, and powerful APIs empowers developers to craft high-performance, maintainable code. Embracing encapsulation, inheritance, polymorphism, stream API, synchronization, and effective exception handling constitutes a significant stride toward maximizing efficiency and overcoming common hurdles in Java development.
By adhering to these best practices and continually refining your skills, you can surmount obstacles and pave the way for a more streamlined, lucrative Java development journey.