Overcoming IP Bans in Distributed Crawling: Best Practices
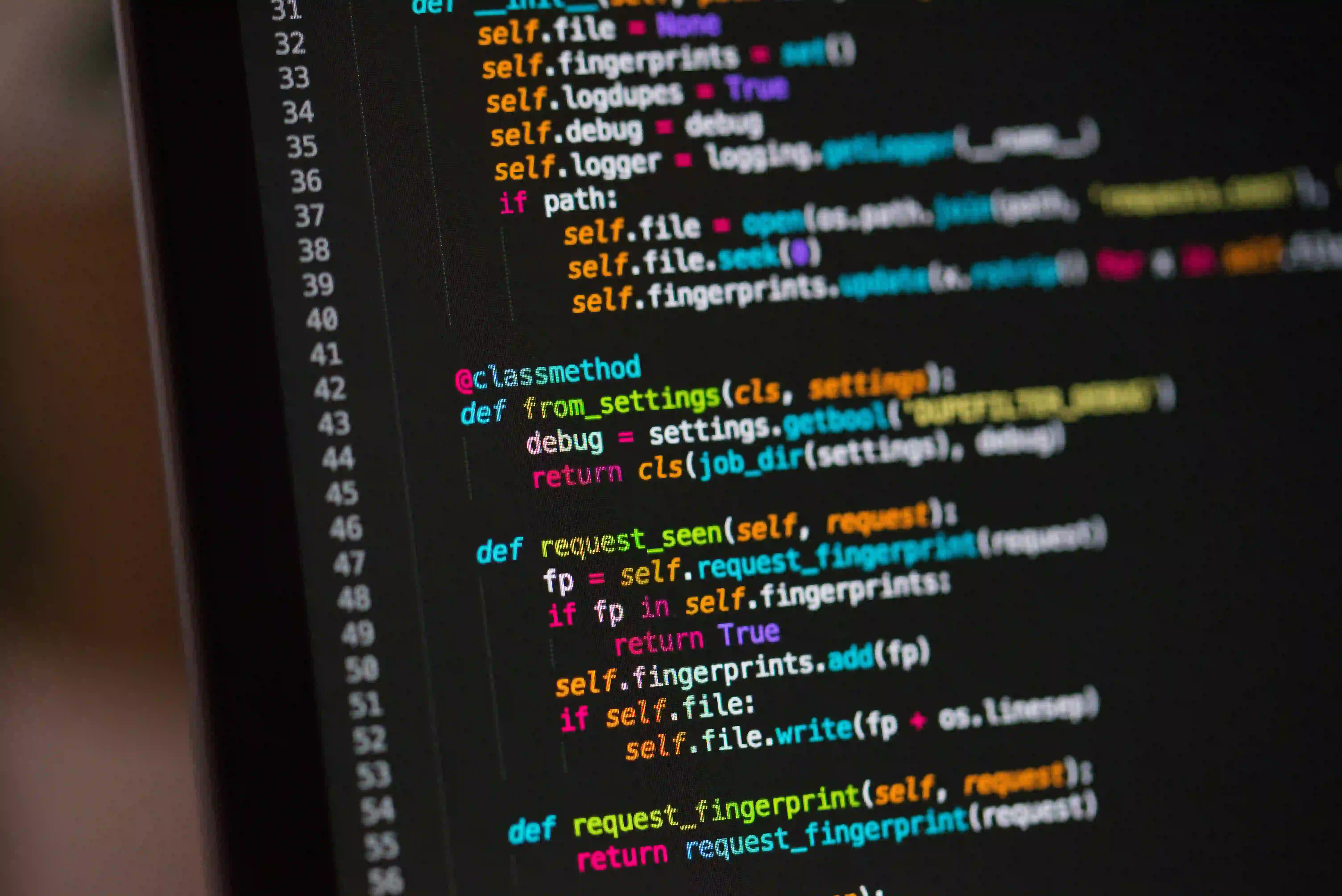
Overcoming IP Bans in Distributed Crawling: Best Practices
In the world of web crawling, dealing with IP bans is a common challenge. Many websites implement IP bans to prevent aggressive crawling, which can hinder the progress of web scraping and crawling tasks. In distributed crawling, where multiple agents are involved, the issue becomes even more complex. In this blog post, we will delve into the best practices for overcoming IP bans in distributed crawling using Java, focusing on strategies to optimize efficiency, avoid detection, and maintain a smooth operation.
Understanding the Challenge
When a crawler is detected and subsequently banned by a website, it can significantly disrupt the crawling process. Without effective measures in place, the entire operation may come to a grinding halt. Moreover, in a distributed crawling setup, where multiple agents are simultaneously accessing the target website, the likelihood of triggering IP bans is amplified.
Proxies and Rotating IP Addresses
One effective strategy for overcoming IP bans in distributed crawling is to utilize a pool of proxies and rotate IP addresses. By routing requests through a diverse set of IP addresses, crawlers can avoid being flagged for excessive traffic from a single source.
Here’s an example of how to integrate a proxy rotation mechanism in Java using the HttpClient
:
CloseableHttpClient httpClient = HttpClients.custom()
.setRoutePlanner(new SystemDefaultRoutePlanner(
new ProxySelector() {
@Override
public List<Proxy> select(URI uri) {
List<Proxy> proxies = new ArrayList<>();
// Populate the list with rotating proxies
return proxies;
}
@Override
public void connectFailed(URI uri, SocketAddress sa, IOException ioe) {
// Handle connection failures
}
}))
.build();
HttpGet httpGet = new HttpGet("https://target-website.com");
CloseableHttpResponse response = httpClient.execute(httpGet);
In this example, the HttpClient
is configured to utilize a custom route planner that selects proxies for each request. By populating the list of rotating proxies, the crawler can effectively mitigate the risk of IP bans.
Rate Limiting and Randomized Delays
Another critical aspect of evading IP bans is implementing rate limiting and randomized delays between requests. By simulating more human-like behavior, crawlers can avoid triggering anti-crawling mechanisms that are designed to detect and block automated activity.
Random random = new Random();
int delay = 1000 + random.nextInt(2000); // Random delay between 1 to 3 seconds
Thread.sleep(delay);
In the above code snippet, a randomized delay is introduced between requests to mimic natural human behavior. This simple yet effective approach can drastically reduce the chances of IP bans resulting from a high frequency of requests.
User-Agent Rotation
Websites often scrutinize the User-Agent header to identify crawlers. By rotating User-Agent strings for each request, crawlers can avoid detection and subsequent IP bans.
HttpUriRequest request = RequestBuilder.get()
.setUri("https://target-website.com")
.setHeader(HttpHeaders.USER_AGENT, UserAgentUtil.getRandomUserAgent())
.build();
In the code above, the User-Agent header is set with a random User-Agent string, which helps the crawler blend in with legitimate web traffic.
Distributed Proxy Management
In a distributed crawling setup, effective proxy management becomes crucial. Coordinating the allocation and rotation of proxy resources among the distributed agents is essential for seamless operations and maintaining a low ban rate.
Handling CAPTCHAs
In some cases, websites may present CAPTCHAs as a means to deter crawlers. Integrating CAPTCHA solving mechanisms, such as CAPTCHA solving services or custom solvers, can be necessary for dealing with this obstacle in a distributed crawling environment.
Monitoring and Adaptive Strategies
Continuous monitoring of the crawling operation, including response codes, request frequencies, and IP ban incidents, is essential. By leveraging this data, adaptive strategies can be employed to dynamically adjust crawling behaviors and mitigate the risk of IP bans.
The Bottom Line
Overcoming IP bans in distributed crawling is a multifaceted challenge that demands a systematic and comprehensive approach. By incorporating proxy rotation, rate limiting, user-agent rotation, distributed proxy management, and adaptive strategies, Java-based crawlers can effectively navigate the complexities of distributed crawling while minimizing the impact of IP bans.
Reference
In conclusion, the key to overcoming IP bans in distributed crawling lies in employing a combination of effective strategies such as proxy rotation, rate limiting, user-agent rotation, distributed proxy management, and adaptive measures. By integrating these practices, Java-based crawlers can maintain a smooth and efficient crawling operation while evading IP bans in a distributed environment.