Spring's Secret: Inject Beans into Unmanaged Objects!
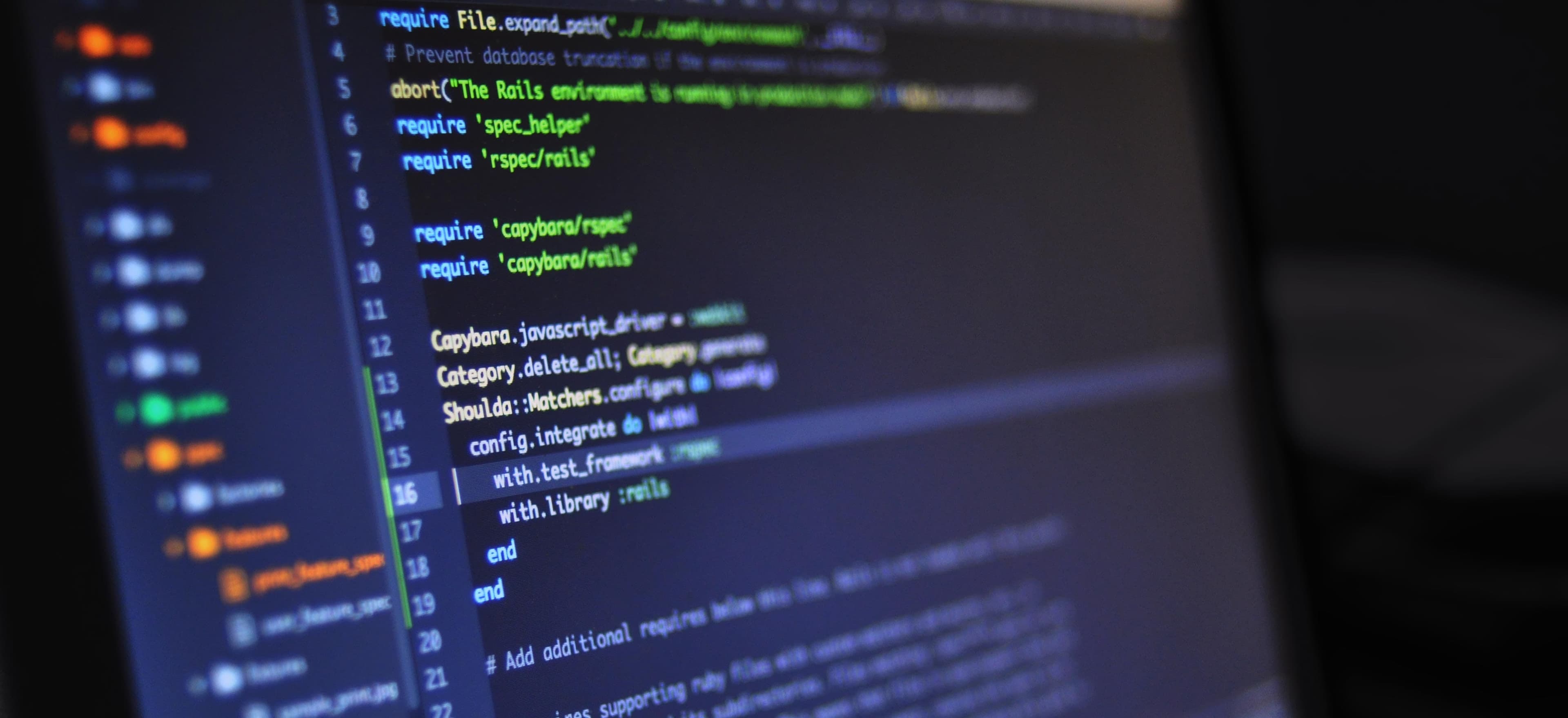
- Published on
Injecting Spring Beans into Unmanaged Objects
When it comes to building modern Java applications, the Spring framework has become a go-to choice for many developers. One of the powerful features of Spring is its ability to manage beans, which are Java objects that are created, managed, and wired together by the Spring IoC (Inversion of Control) container. However, there are times when you may want to inject Spring beans into objects that are not managed by the Spring container. In this blog post, we'll explore a lesser-known feature of Spring that allows us to do just that.
The @Configurable Annotation
Spring provides the @Configurable
annotation that enables dependency injection into non-Spring managed objects. This means that you can use Spring's powerful dependency injection capabilities even for objects that are created using the new
keyword, outside of the Spring container.
How It Works
To use the @Configurable
annotation, you need to perform the following steps:
-
Enable the
@EnableSpringConfigured
annotation in your Spring configuration. -
Use AspectJ to perform load-time weaving or compile-time weaving.
Let's dive into a practical example to see how it all comes together.
@EnableSpringConfigured
@Configuration
public class AppConfig {
// Your Spring configuration
}
In this example, we enable the @EnableSpringConfigured
annotation in a Spring configuration class. This tells Spring to enable the configuration needed for @Configurable
to work.
Next, let's look at how to use the @Configurable
annotation in a regular Java class.
@Configurable
public class NonSpringManagedObject {
@Autowired
private SomeSpringBean someSpringBean;
public void doSomething() {
someSpringBean.doSomething();
}
}
In this example, we've annotated the NonSpringManagedObject
class with @Configurable
, indicating that it should be treated as a Spring bean for the purpose of dependency injection. We also used the @Autowired
annotation to inject a Spring bean (SomeSpringBean
) into the NonSpringManagedObject
.
Important Considerations
It's important to note that using the @Configurable
annotation may come with some trade-offs and considerations:
-
AspectJ Weaving: As mentioned earlier, to make
@Configurable
work, you need to use AspectJ for load-time weaving or compile-time weaving. This introduces a level of complexity and might not be suitable for all projects. -
Performance Overhead: The use of AspectJ weaving may introduce some performance overhead, so it's essential to weigh the benefits against the potential drawbacks for your specific use case.
-
Complexity: While
@Configurable
provides flexibility, it may also add complexity to your codebase, especially for developers who are not familiar with AspectJ weaving.
Use Cases
The ability to inject Spring beans into non-Spring managed objects opens up a range of possibilities. Here are a few use cases where this feature can be particularly useful:
Legacy Code Integration
If you're working on a project that involves integrating new Spring-based components with existing legacy code, the @Configurable
annotation can be a lifesaver. You can use it to inject modern Spring beans into legacy objects, allowing for a gradual migration to a more modern architecture.
Third-Party Libraries
There are situations where you may need to work with third-party libraries or frameworks that are not designed for use within a Spring container. By using @Configurable
, you can still leverage the power of Spring's dependency injection in such scenarios.
Testing
When writing unit tests, you may need to create instances of non-Spring managed objects and inject mock dependencies into them. The @Configurable
annotation can be instrumental in such testing scenarios, allowing you to seamlessly inject mock dependencies without refactoring the existing codebase.
To Wrap Things Up
While the @Configurable
annotation provides a powerful mechanism for injecting Spring beans into non-Spring managed objects, it's essential to use it judiciously. Understanding the trade-offs and implications of using AspectJ weaving is crucial for making informed decisions about its adoption in your projects.
By being aware of this lesser-known feature of Spring, you can expand your toolkit for integrating modern Spring-based components with legacy code, working with third-party libraries, and streamlining your testing processes.
In conclusion, the @Configurable
annotation unlocks a hidden capability within Spring and demonstrates the framework's flexibility in accommodating diverse application architectures and scenarios.
To explore more about Spring's advanced features, check out the official Spring documentation.
Ready to enhance your Java development with Spring's secret powers? Give @Configurable
a try and harness the full potential of Spring's dependency injection capabilities!
Checkout our other articles