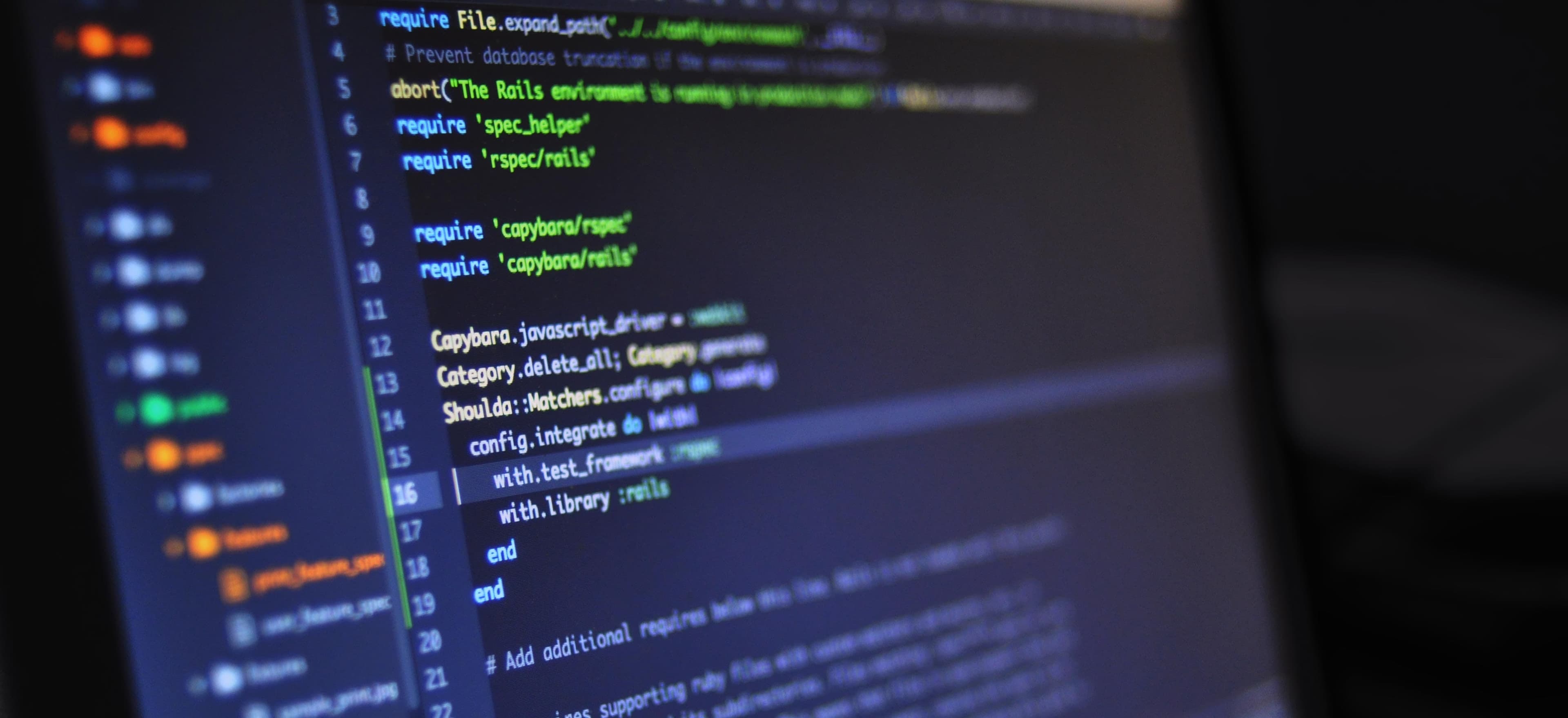
- Published on
Mastering Checkbox Woes in Spring MVC: A Quick Guide
Checkboxes in forms are a common source of frustration for developers working with Spring MVC. Handling checkbox data and binding it to the model correctly can be tricky. In this guide, we'll delve into the nuances of dealing with checkboxes in Spring MVC forms and provide practical solutions to common issues.
The Checkbox Conundrum
When working with a form that includes checkboxes, you might encounter challenges such as:
- Data Binding: Ensuring that the selected checkbox values are properly bound to the model.
- Handling Multiple Selections: Dealing with scenarios where multiple checkboxes can be selected.
- Processing Checkbox Values: Extracting and processing checkbox values on form submission.
In the context of Spring MVC, these challenges can be addressed effectively with the right strategies and best practices.
The Model
Let's start with the model. Consider a scenario where you have a User
class with a field that represents user roles, and you want the user to be able to select multiple roles using checkboxes.
public class User {
private List<String> roles;
// Getter and setter for roles
}
In the User
class, the roles
field is a list of strings representing the selected roles. This is a typical scenario where checkboxes are used for multi-selection.
The Form
Next, let's look at how the form is structured in the JSP file.
<form:form method="post" modelAttribute="user">
<form:checkboxes path="roles" items="${allRoles}" />
<input type="submit" value="Save" />
</form:form>
In the form, the form:checkboxes
tag is used to generate checkboxes for the roles
field. The allRoles
attribute contains the list of all available roles from which the user can choose.
Data Binding
One common issue with checkboxes in Spring MVC is ensuring that the selected checkbox values are correctly bound to the model when the form is submitted. This is where data binding comes into play.
Spring MVC uses the @ModelAttribute
annotation to bind form data to the model. In our controller, we can use the following approach to handle checkbox data binding:
@Controller
public class UserController {
@PostMapping("/saveUser")
public String saveUser(@ModelAttribute("user") User user) {
// Process the user object with selected roles
// ...
return "success";
}
}
In the saveUser
method, the @ModelAttribute
annotation automatically binds the form data to the User
object, including the selected roles from the checkboxes.
Handling Multiple Selections
Another challenge is handling scenarios where multiple checkboxes can be selected. Spring MVC simplifies this by automatically binding the selected values to a collection in the model, such as a list.
By defining the roles
field as a List<String>
in the User
class, Spring MVC will automatically populate this list with the selected role values from the checkboxes.
Processing Checkbox Values
Once the form is submitted, you may need to process the selected checkbox values. This often involves iterating through the collection of selected values and performing the necessary operations.
In the controller method that handles the form submission, you can access the selected roles from the User
object and process them accordingly.
@Controller
public class UserController {
@PostMapping("/saveUser")
public String saveUser(@ModelAttribute("user") User user) {
List<String> selectedRoles = user.getRoles();
// Process the selected roles
// ...
return "success";
}
}
Here, the getRoles
method retrieves the list of selected roles from the User
object, allowing you to perform any required processing based on the selected values.
Closing the Chapter
Dealing with checkboxes in Spring MVC forms doesn't have to be a headache. By understanding the intricacies of data binding, handling multiple selections, and processing checkbox values, you can master the checkbox woes that often confound developers.
With the right approach and best practices, you can effectively incorporate checkboxes into your Spring MVC forms and streamline the management of multi-select data.
By following the guidelines presented in this guide, you'll be well-equipped to tackle checkbox challenges with confidence and precision in your Spring MVC applications.
Now that you've learned how to handle checkboxes in Spring MVC forms, you may also be interested in exploring Spring MVC Form Validation to further enhance your form handling capabilities.
Remember, mastering checkboxes is just one piece of the larger puzzle in building robust, user-friendly web applications with Spring MVC.
Checkout our other articles