5 Common Pitfalls in Software Architectural Decision-Making
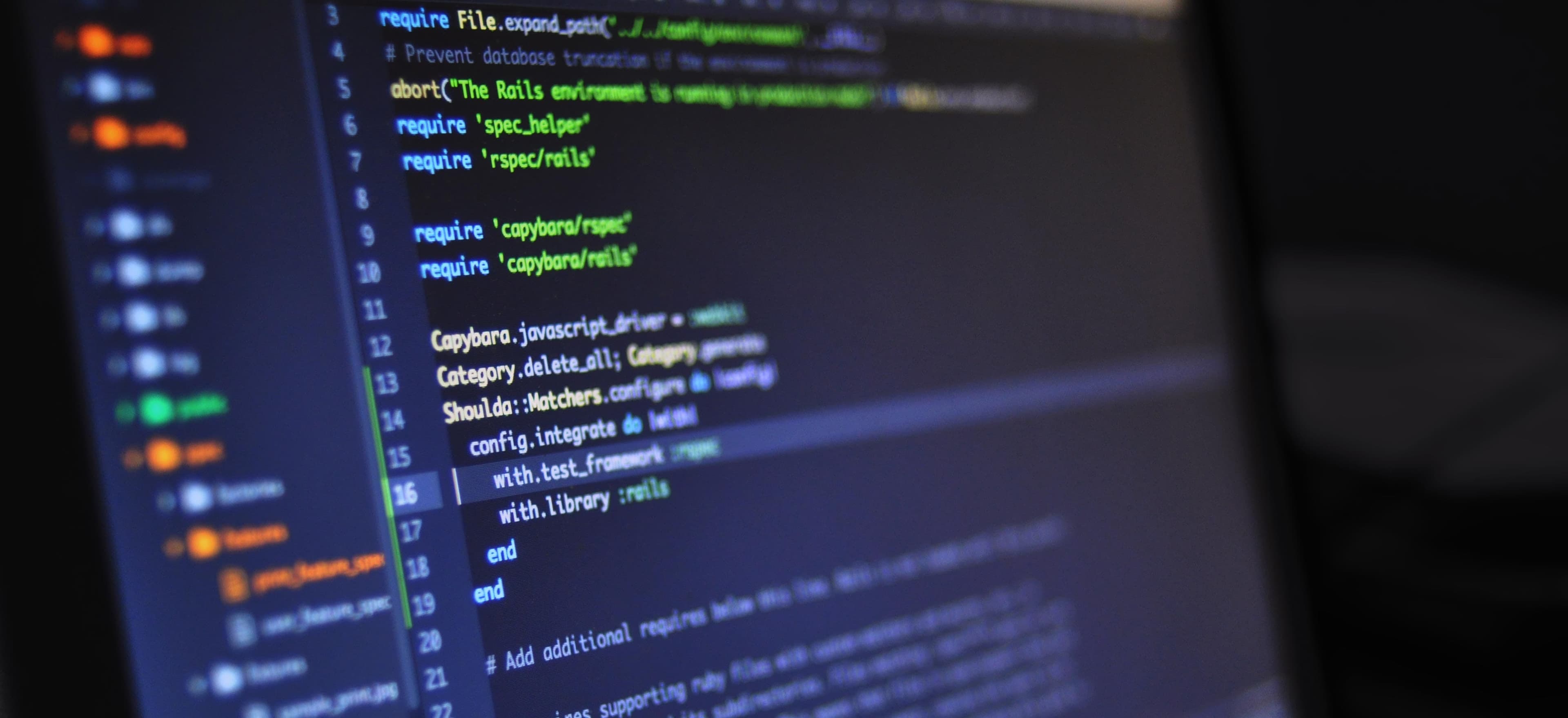
- Published on
5 Common Pitfalls in Software Architectural Decision-Making
As a Java developer, making sound architectural decisions is crucial for creating robust, scalable, and maintainable software. However, there are common pitfalls that can derail architectural decision-making, leading to suboptimal solutions. In this article, we'll explore five of these pitfalls and discuss strategies to avoid them.
1. Over-Engineering
One of the most prevalent pitfalls in software architectural decision-making is over-engineering. This occurs when developers introduce overly complex solutions to problems that could be adequately addressed with simpler designs. Over-engineering can lead to increased development time, unnecessary abstraction, and higher maintenance costs.
Example of Over-Engineering in Java
Consider the following code snippet:
public class OverEngineeredService {
private SomeComplexDependency dependency;
public OverEngineeredService() {
this.dependency = new SomeComplexDependency(new ConfigParams(), true, "extra");
}
// ... (other methods)
}
In this example, the OverEngineeredService
unnecessarily creates a complex dependency using a constructor with multiple parameters. This can lead to rigid coupling and make the code harder to maintain.
How to Avoid Over-Engineering
To avoid over-engineering, developers should prioritize simplicity and incremental design. By starting with minimalistic solutions and gradually evolving the architecture as requirements become clearer, teams can avoid the trap of over-engineering.
2. Lack of Modularization
Another common pitfall is the lack of modularization in architectural decision-making. When architecture lacks proper modularization, it becomes challenging to comprehend, maintain, and extend. This often leads to monolithic, tightly coupled systems that are difficult to scale and test.
Example of Lack of Modularization in Java
public class MonolithicApplication {
// ... (many lines of code)
public void monolithicMethod() {
// ... (hundreds of lines of code)
}
// ... (more methods)
}
In this example, the MonolithicApplication
class contains a single, monolithic method, making it hard to understand and maintain.
How to Avoid Lack of Modularization
To address this pitfall, developers should embrace modular design principles, such as separation of concerns, single responsibility, and loose coupling. By breaking down complex systems into cohesive, independent modules, teams can improve maintainability, reusability, and scalability.
3. Ignoring Non-Functional Requirements
Ignoring non-functional requirements, such as performance, scalability, security, and maintainability, is a critical pitfall in architectural decision-making. Focusing solely on functional aspects while neglecting non-functional considerations can result in systems that fail to meet crucial performance and security standards.
Example of Ignoring Non-Functional Requirements in Java
public class IgnoringNonFunctionalService {
// ... (implementation without considering performance or security)
}
In this example, the IgnoringNonFunctionalService
class implements a functionality without considering critical non-functional requirements.
How to Avoid Ignoring Non-Functional Requirements
To avoid this pitfall, architectural decision-making should include thorough analysis of non-functional requirements from the outset. Incorporating performance testing, security audits, and scalability assessments into the architectural process ensures that non-functional aspects are given the attention they deserve.
4. Tight Coupling
Tight coupling between components or modules is a prevalent pitfall that can hinder flexibility, reusability, and testability. When components are tightly coupled, making changes to one part of the system often necessitates modifications across multiple modules, leading to cascading effects and fragility.
Example of Tight Coupling in Java
public class TightCouplingClass {
private AnotherTightCouplingClass dependency;
public TightCouplingClass() {
this.dependency = new AnotherTightCouplingClass();
}
// ... (usage of dependency)
}
In this example, the TightCouplingClass
directly instantiates and uses AnotherTightCouplingClass
, creating tight coupling between the two components.
How to Avoid Tight Coupling
To mitigate tight coupling, developers should leverage principles such as dependency injection, inversion of control, and interfaces. By decoupling components and favoring abstractions over concrete implementations, architectural designs become more flexible and easier to maintain.
5. Lack of Documentation and Communication
Finally, a lack of documentation and communication around architectural decisions can lead to misunderstandings, inconsistency, and reduced maintainability. When architectural rationale and design choices are not well-documented or communicated, it becomes challenging for team members to understand the system's intent and make informed modifications.
Example of Lack of Documentation and Communication in Java
// No comments or documentation explaining the rationale behind the architectural choices
public class UndocumentedService {
// ... (implementation without clear explanations)
}
In this example, the UndocumentedService
lacks sufficient documentation to explain the architectural decisions.
How to Avoid Lack of Documentation and Communication
To address this pitfall, teams should prioritize clear, concise documentation of architectural decisions, including rationale, trade-offs, and known limitations. Additionally, fostering open communication channels and conducting regular architecture reviews can help ensure that everyone involved understands and agrees on the architectural direction.
Final Thoughts
In conclusion, navigating the landscape of software architectural decision-making requires vigilance to avoid common pitfalls such as over-engineering, lack of modularization, ignoring non-functional requirements, tight coupling, and lack of documentation and communication. By recognizing these pitfalls and implementing strategies to mitigate them, Java developers can craft architectural solutions that are resilient, maintainable, and effective over the long term.
Remember, the art of architectural decision-making is an ongoing journey, and by learning from these common pitfalls, developers can pave the way for more robust and successful software systems.
So, have you encountered any of these pitfalls in your architectural decision-making? How did you address them? Share your experiences in the comments below.
To dive deeper into the world of software architecture and design patterns, check out this comprehensive guide from Toptal.
For in-depth insights into Java best practices and architectural patterns, give this article on "Effective Java" a read.
Happy coding!