Avoid These Common Pitfalls in Unit Testing Practices
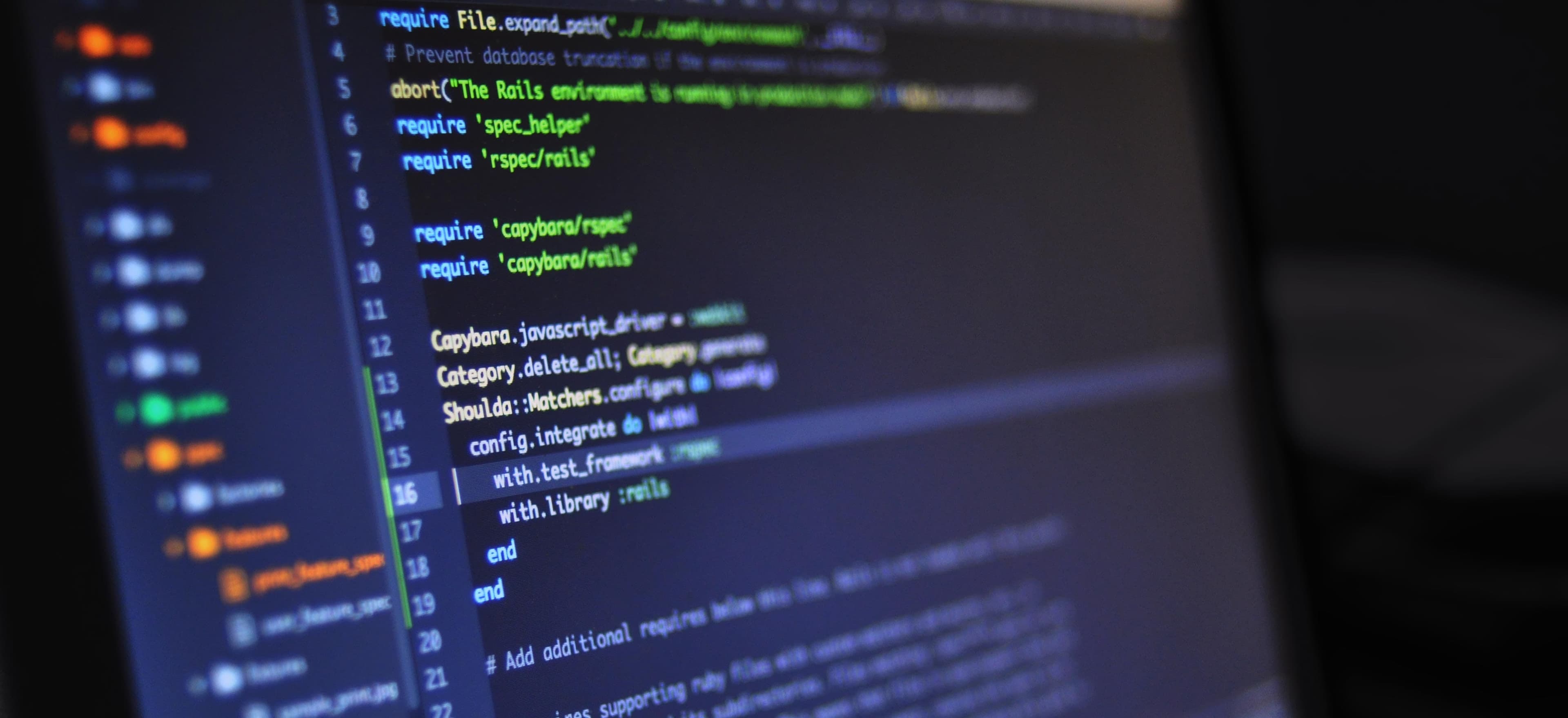
- Published on
Avoid These Common Pitfalls in Unit Testing Practices
Unit testing is a critical aspect of software development that helps ensure the reliability and functionality of code. However, many developers face challenges when it comes to creating effective unit tests. In this article, we'll discuss some common pitfalls in unit testing practices and how to avoid them.
Pitfall 1: Testing Too Much or Too Little
Writing unit tests that cover every single line of code can be time-consuming and impractical. On the other hand, writing too few tests can leave gaps in test coverage, leading to undetected bugs. The key is to find the right balance.
Solution:
Focus on testing critical functionality: Prioritize unit testing for critical and complex business logic. Writing tests for every getter and setter may not be necessary, while testing complex validation or calculation logic is crucial.
Use code coverage tools: Tools such as JaCoCo and Cobertura can help identify areas of code that lack test coverage, allowing you to focus your testing efforts more effectively.
Pitfall 2: Ignoring Test Independence
Unit tests should be independent of each other to ensure that a failure in one test does not impact the execution of other tests. This can be challenging when tests share common setup or state.
Solution:
Avoid test dependencies: Refrain from relying on the state or output of one test to drive another test. Each test should be able to run on its own without any external dependencies.
Use setup and teardown methods: Utilize setup and teardown methods provided by testing frameworks like JUnit to initialize and clean up test fixtures, ensuring test isolation.
@Before
public void setUp() {
// Initialize test fixtures
}
@After
public void tearDown() {
// Clean up test fixtures
}
Pitfall 3: Overlooking Test Readability and Maintainability
Complex and unreadable tests can be difficult to maintain and understand. This can lead to a reluctance to update or add new tests, ultimately decreasing overall test effectiveness.
Solution:
Write clear and descriptive test names: Use descriptive names that convey the purpose of the test. This makes it easier to understand the test's intent without delving into the implementation details.
Refactor repetitive code into helper methods: If certain setup or assertion logic is repeated across multiple tests, refactor it into helper methods to improve readability and maintainability.
private void assertOrderTotal(double expectedTotal, Order order) {
assertEquals(expectedTotal, order.calculateTotal(), 0.01);
}
Pitfall 4: Neglecting Edge Cases and Error Handling
Failing to consider edge cases and error scenarios can result in incomplete test coverage. Unit tests should not only validate expected behaviors but also handle unexpected conditions.
Solution:
Identify edge cases: Think about boundary values, null inputs, and exceptional scenarios that the code might encounter. Writing tests specifically for these cases ensures robustness.
Use parameterized tests: Parameterized tests, available in frameworks like JUnit, allow you to run the same test with different inputs, making it easier to cover a range of scenarios.
@Parameters
public static Collection<Object[]> data() {
return Arrays.asList(new Object[][] {
{ 0, "zero" },
{ 1, "one" },
{ 2, "two" },
{ 3, "three" }
});
}
@Test
public void testNumberToWordConversion(int input, String expected) {
assertEquals(expected, NumberConverter.convertToWord(input));
}
Pitfall 5: Relying Solely on Mocks or Stubs
While mocks and stubs are valuable in isolating code for testing, relying too heavily on them can lead to incomplete testing of real interactions between components.
Solution:
Strive for a balance: Use mocks and stubs strategically to isolate dependencies, but also incorporate integration tests to verify the interactions between real components.
Leverage tools for mock verification: Tools like Mockito provide methods to verify interactions with mocks, ensuring that the expected interactions indeed occur during the test.
verify(mockedList, times(2)).add("example");
Pitfall 6: Disregarding Test Performance
As the codebase grows, the number of unit tests can also increase significantly, potentially impacting build and test execution times.
Solution:
Run tests in parallel: Leverage testing frameworks or build tools that support parallel test execution, reducing the overall test execution time.
Identify slow tests: Use profiling tools to identify and optimize slow tests, ensuring that they do not become a bottleneck in the test suite.
The Bottom Line
Unit testing, when done effectively, can significantly improve the quality and maintainability of code. By avoiding these common pitfalls and following best practices, developers can create robust and maintainable unit test suites that contribute to the overall success of the software development lifecycle.
Remember, the goal of unit testing is to provide fast feedback on the correctness of individual code units, and by understanding and addressing these pitfalls, developers can achieve just that.
Now it’s your turn - what others pitfalls do you beileve that devs should avoid?