Clean Your Data: How to Remove Duplicate Rows in SQL
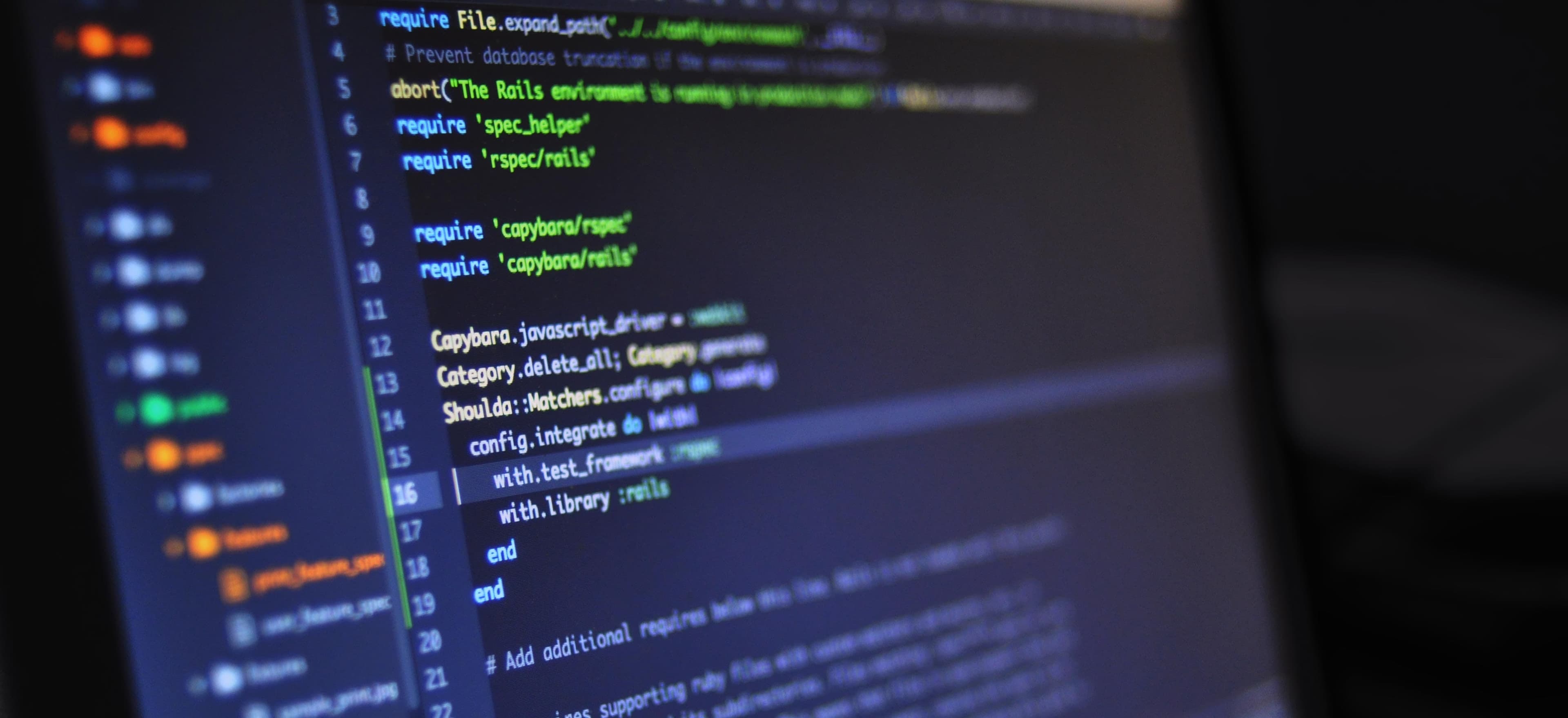
- Published on
Removing Duplicate Rows in SQL: A Step-By-Step Guide
In the world of programming, dealing with datasets is inevitable. Whether you're working with customer information, financial records, or any other type of data, duplicate rows can be a common nuisance. Not only do they clutter your data, but they can also lead to incorrect analysis and results. In this blog post, we'll explore how to remove duplicate rows in SQL, specifically focusing on Java and its integration with SQL databases. By the end of this guide, you'll have a clear understanding of how to efficiently clean your data and maintain the integrity of your database.
Why Removing Duplicate Rows Matters
Duplicate rows in a database can cause several issues. First and foremost, they present an inaccurate representation of the underlying data. When performing data analysis or generating reports, these duplicates can skew the results and potentially lead to incorrect business decisions. Moreover, duplicates consume unnecessary storage space, which, in turn, affects the overall performance of the database. Therefore, removing duplicate rows is essential for maintaining data quality, ensuring accurate analysis, and optimizing database performance.
Using Java to Remove Duplicate Rows in SQL:
Java, with its robust database connectivity capabilities, is commonly used to interact with SQL databases. The JDBC (Java Database Connectivity) API provides a platform-independent method for accessing any tabular data source, including relational databases. Combining the power of Java with SQL, let’s dive into the process of identifying and eliminating duplicate rows from a database table.
Step 1: Connect to the Database
Before diving into the process of removing duplicate rows, it's crucial to establish a connection to the SQL database using Java. The following code snippet demonstrates how to connect to a MySQL database using Java's JDBC API.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DatabaseConnector {
public static void main(String[] args) {
Connection connection = null;
try {
// Establish a connection to the database
String url = "jdbc:mysql://localhost:3306/database_name";
String username = "your_username";
String password = "your_password";
connection = DriverManager.getConnection(url, username, password);
if (connection != null) {
System.out.println("Connected to the database!");
}
} catch (SQLException e) {
// Handle any SQL errors
e.printStackTrace();
} finally {
try {
if (connection != null) {
connection.close();
}
} catch (SQLException ex) {
// Handle any SQL errors during connection closing
ex.printStackTrace();
}
}
}
}
In the above code, we use the DriverManager
class to connect to a MySQL database. You need to replace jdbc:mysql://localhost:3306/database_name
with the actual URL of your MySQL database, and provide the correct username
and password
for authentication.
Step 2: Identify Duplicate Rows
Once the connection is established, the next step is to identify the duplicate rows within a specific table. This can be achieved by executing a SQL query that uses the GROUP BY
and HAVING
clauses to filter out the duplicates.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
public class DuplicateRowIdentifier {
public static void main(String[] args) {
Connection connection = null;
try {
String url = "jdbc:mysql://localhost:3306/database_name";
String username = "your_username";
String password = "your_password";
connection = DriverManager.getConnection(url, username, password);
if (connection != null) {
System.out.println("Connected to the database!");
// Identify duplicate rows
Statement statement = connection.createStatement();
String sqlQuery = "SELECT column1, column2, COUNT(*), MIN(id) FROM your_table_name GROUP BY column1, column2 HAVING COUNT(*) > 1";
ResultSet resultSet = statement.executeQuery(sqlQuery);
while (resultSet.next()) {
System.out.println("Duplicate Found: " + resultSet.getString("column1") + ", " + resultSet.getString("column2"));
}
statement.close();
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (connection != null) {
connection.close();
}
} catch (SQLException ex) {
ex.printStackTrace();
}
}
}
}
Replace column1
, column2
, your_table_name
, and id
with the relevant column names and table name from your database. The SQL query groups the rows based on specific columns and filters out the duplicates by using the HAVING COUNT(*) > 1
condition.
Step 3: Remove Duplicate Rows
After identifying the duplicate rows, the final step is to remove them from the database table. This is accomplished by executing a delete operation using another SQL query.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import java.sql.Statement;
public class DuplicateRowRemover {
public static void main(String[] args) {
Connection connection = null;
try {
String url = "jdbc:mysql://localhost:3306/database_name";
String username = "your_username";
String password = "your_password";
connection = DriverManager.getConnection(url, username, password);
if (connection != null) {
System.out.println("Connected to the database!");
// Remove duplicate rows
Statement statement = connection.createStatement();
String sqlQuery = "DELETE FROM your_table_name WHERE id NOT IN (SELECT MIN(id) FROM your_table_name GROUP BY column1, column2)";
int rowsDeleted = statement.executeUpdate(sqlQuery);
System.out.println("Duplicate rows removed: " + rowsDeleted);
statement.close();
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (connection != null) {
connection.close();
}
} catch (SQLException ex) {
ex.printStackTrace();
}
}
}
}
In the above code, we use a DELETE
statement to remove the duplicate rows from the database table. The subquery SELECT MIN(id) FROM your_table_name GROUP BY column1, column2
is used to retain only the first occurrence of each duplicate group, while deleting the rest.
Final Considerations
In this guide, we explored the process of removing duplicate rows from a database table using Java and SQL. We started by establishing a connection to the database, then identified the duplicate rows based on specific criteria, and finally removed the duplicates to clean the dataset. It's important to note that before executing such operations in a production environment, it's advisable to make database backups and carefully review the delete operations to avoid unintended data loss.
By following the steps outlined in this guide, you can effectively clean your data and ensure the accuracy and integrity of your database. Removing duplicate rows not only improves data quality but also contributes to better and more reliable data analysis.
So, next time you encounter duplicate rows in your SQL database, remember these steps and use Java to efficiently clean your data, ultimately enhancing the robustness of your database.
In your journey of working with Java and SQL, there might be additional circumstances where you'll need to manage and manipulate data. Understanding Java's integration with SQL and various database operations will be beneficial. Feel free to explore the wealth of information on Java Code Geeks for further insights into Java development and SQL database management.
Checkout our other articles