Boost Your API Game: Top Pitfalls in Automation Testing!
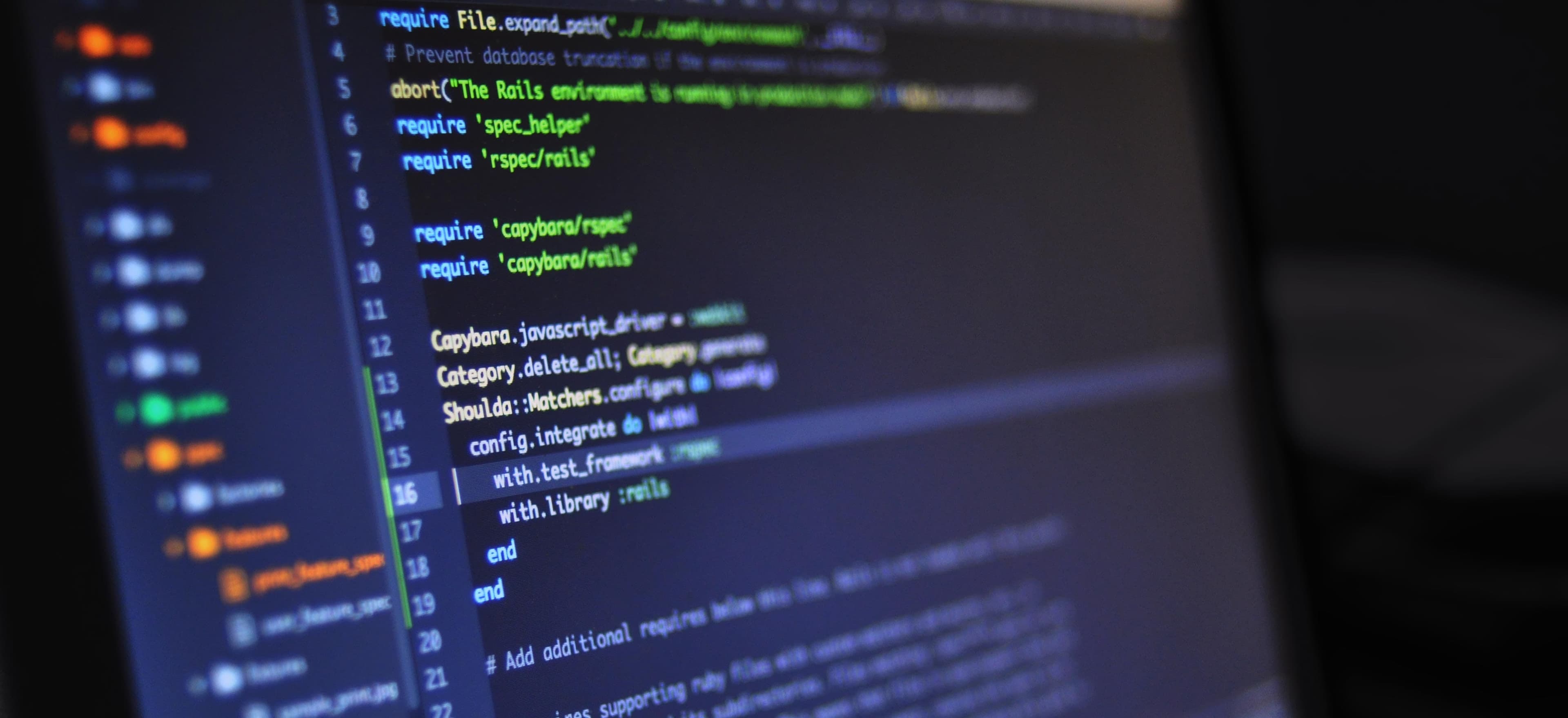
- Published on
Boost Your API Game: Top Pitfalls in Automation Testing
APIs have become the backbone of modern software development, enabling seamless integration and communication between different systems. With the increasing complexity of APIs, the need for robust automation testing has become more critical than ever. In this blog post, we'll explore some of the top pitfalls in API automation testing and how to overcome them using Java.
Pitfall 1: Inadequate Test Coverage
One of the common pitfalls in API automation testing is inadequate test coverage. It's essential to ensure that your test suite covers a wide range of scenarios, including various input combinations, error handling, and boundary conditions. In Java, you can address this by leveraging libraries like RestAssured, which provides a rich set of features for crafting expressive and comprehensive API tests.
@Test
public void testCreateUserAPI() {
given()
.contentType(ContentType.JSON)
.body(user)
.when()
.post("/users")
.then()
.statusCode(201);
// Additional assertions for response body
}
In the above example, we're using RestAssured to perform a POST request to the /users
endpoint and asserting the response status code. This allows for thorough testing of the API's functionality, ensuring adequate test coverage.
Pitfall 2: Brittle Tests
Brittle tests are another common issue in API automation testing, where tests are overly sensitive to changes in the API implementation. To mitigate this, it's crucial to write stable and maintainable tests that focus on the expected behavior rather than the internal implementation details. In Java, you can achieve this by utilizing libraries like JUnit and Hamcrest for fluent and resilient assertions.
@Test
public void testUpdateUserAPI() {
User updatedUser = new User("John Doe", "johndoe@example.com");
given()
.contentType(ContentType.JSON)
.body(updatedUser)
.when()
.put("/users/{id}", userId)
.then()
.statusCode(200)
.body("name", equalTo("John Doe"))
.body("email", equalTo("johndoe@example.com"));
}
In this example, we're using Hamcrest matchers within JUnit assertions to validate the response body's specific attributes. This creates more resilient tests that are less prone to breaking due to minor API changes.
Pitfall 3: Lack of Data Management
Effective API testing often requires the management of test data, including the creation of test-specific data and the cleanup of resources after test execution. In Java, you can address this by integrating tools like TestNG or JUnit with frameworks like Mockito to simulate complex API responses and manage test data effectively.
@Test
public void testGetUserAPI() {
// Setup
User expectedUser = new User("Jane Smith", "janesmith@example.com");
given(userService.getUser(userId)).willReturn(expectedUser);
// Test
given()
.pathParam("id", userId)
.when()
.get("/users/{id}")
.then()
.statusCode(200)
.body("name", equalTo("Jane Smith"))
.body("email", equalTo("janesmith@example.com"));
// Verification
verify(userService).getUser(userId);
}
Here, we're using Mockito to mock the behavior of a userService
and simulate the expected API response. By managing test data and interactions with external dependencies, you can ensure more robust and reliable API tests.
Pitfall 4: Ignoring Security Testing
Security vulnerabilities in APIs can pose significant risks to the overall system's integrity. However, it's common for teams to overlook security testing in their API automation strategy. In Java, you can incorporate security testing into your automation suite by using libraries like OWASP ZAP or Burp Suite in conjunction with tools such as JUnit and Cucumber to perform targeted security tests.
@SecurityTest
public void testSecureEndpoint() {
given()
.auth().oauth2(accessToken)
.when()
.get("/secure-endpoint")
.then()
.statusCode(200);
}
In this example, we're using RestAssured to perform an authenticated request to a secure endpoint, ensuring that the API's security measures are functioning as expected.
In Conclusion, Here is What Matters
API automation testing is a crucial aspect of ensuring the reliability and stability of your applications. By addressing these common pitfalls and leveraging the power of Java and its associated libraries, you can elevate your API testing game and build a more resilient and robust automation suite.
To delve deeper into the world of API testing and Java, consider exploring the official documentation for RestAssured and JUnit. These valuable resources provide further insights and best practices for achieving excellence in API automation testing using Java.