Maximizing UI Design: Taming JavaFX BorderPane Issues
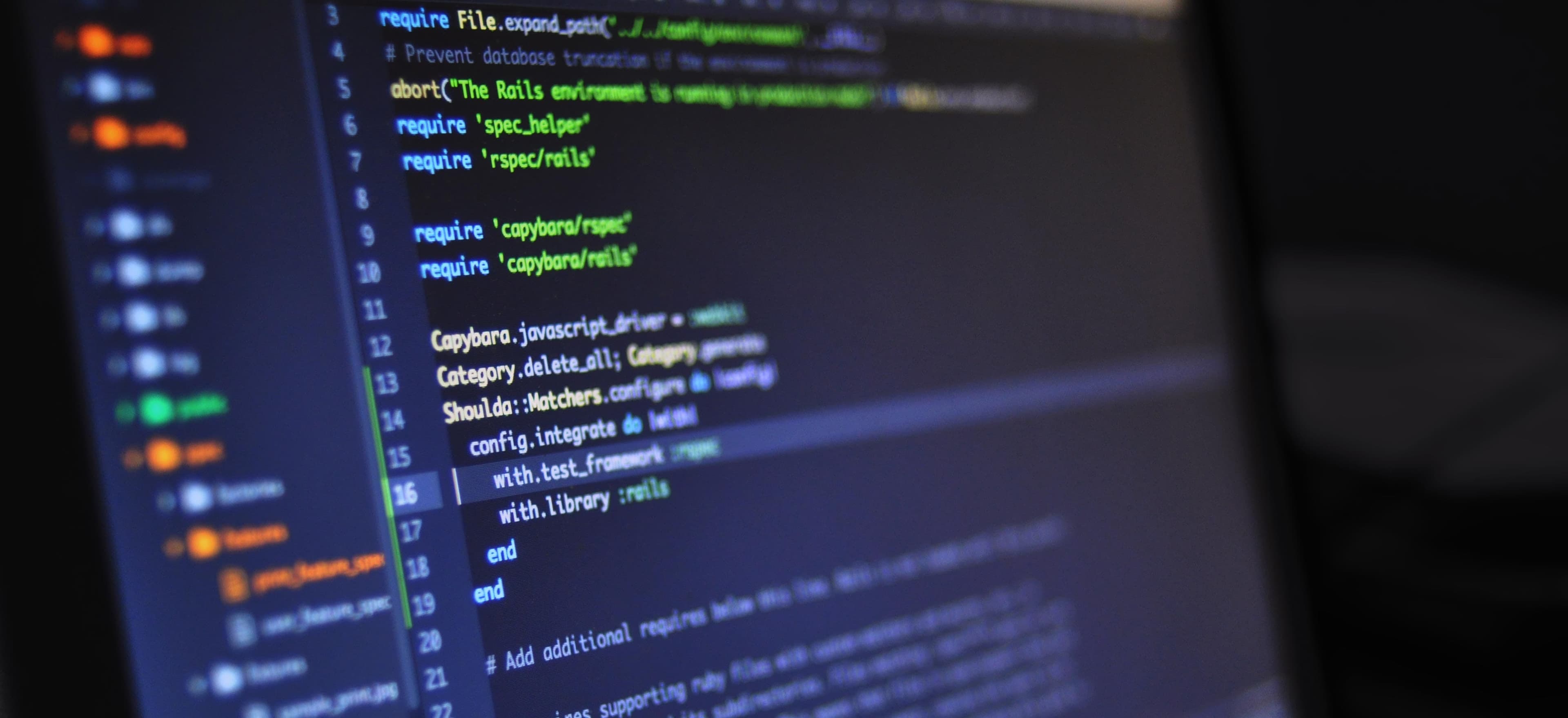
- Published on
Maximizing UI Design: Taming JavaFX BorderPane Issues
When it comes to building modern and visually appealing user interfaces in Java applications, JavaFX is a go-to choice for many developers. JavaFX provides a rich set of libraries and components for creating interactive and responsive UIs. However, like any UI framework, JavaFX has its own set of challenges, especially when it comes to laying out UI components effectively.
In this article, we'll delve into one of the core layout panes in JavaFX - the BorderPane. While the BorderPane layout can be a powerful tool for organizing UI elements, it also comes with its own set of quirks and challenges. We'll explore some common issues that developers encounter when working with BorderPane and discuss strategies for overcoming these challenges to maximize UI design.
Understanding the BorderPane
The BorderPane layout is a versatile container in JavaFX that divides the space into five regions: top, bottom, left, right, and center. This allows developers to easily position UI components in relation to these regions, making it suitable for a wide range of UI designs.
Common Issues with BorderPane
1. Component Overlapping
One of the most common issues with BorderPane is the overlapping of UI components, especially when components are added to the same region. This can lead to a cluttered and unattractive UI, making it crucial to address this issue effectively.
2. Lack of Responsiveness
Another challenge with BorderPane is ensuring that UI components respond effectively to changes in the window size. Without careful handling, components may not resize or reposition as intended, leading to a lack of responsiveness in the UI layout.
3. Complex Nesting
As UI designs become more intricate, the nesting of multiple BorderPanes within each other can lead to complex and convoluted layouts. This makes it difficult to manage the placement and sizing of components effectively.
Strategies for Taming BorderPane Issues
1. Proper Use of Constraints
When adding components to a BorderPane, it's essential to utilize proper constraints to define the placement of the components within the layout. This involves specifying the region (top, bottom, left, right, center) to which the component should be added.
BorderPane borderPane = new BorderPane();
Button topButton = new Button("Top");
borderPane.setTop(topButton);
2. Utilizing HBox and VBox for Complex Layouts
In cases where a single region of the BorderPane requires multiple components to be laid out horizontally or vertically, using an HBox or VBox as the child of the respective region can provide a more flexible and responsive layout.
BorderPane borderPane = new BorderPane();
HBox topBox = new HBox();
Button button1 = new Button("Button 1");
Button button2 = new Button("Button 2");
topBox.getChildren().addAll(button1, button2);
borderPane.setTop(topBox);
3. Handling Resizability
To address the responsiveness challenge, it's important to manage the responsiveness of components within the BorderPane. This can be achieved by utilizing layout constraints and specifying how components should resize when the window dimensions change.
BorderPane borderPane = new BorderPane();
Button centerButton = new Button("Center");
BorderPane.setMargin(centerButton, new Insets(10));
BorderPane.setAlignment(centerButton, Pos.CENTER);
borderPane.setCenter(centerButton);
4. Simplifying Complex Nesting
To avoid overly complex nested BorderPane layouts, consider alternative layout strategies such as using AnchorPane or creating custom composite components to encapsulate specific UI designs and functionalities.
public class CustomControl extends Region {
public CustomControl() {
getChildren().add(new Button("Custom Button"));
setStyle("-fx-background-color: lightgray;");
}
}
Closing Remarks
By understanding the nuances of JavaFX's BorderPane and implementing the strategies discussed in this article, developers can effectively address common UI layout challenges. Proper use of constraints, leveraging HBox and VBox for complex layouts, handling resizability, and simplifying complex nesting are key tactics for taming BorderPane issues and maximizing UI design in Java applications. With these insights and best practices, developers can craft more intuitive and visually appealing user interfaces that enhance the overall user experience of their Java applications.
For more in-depth insights into JavaFX and UI design best practices, check out JavaFX Documentation and Oracle's official documentation.