Securing Atomic Operations on Volatile Fields: A Guide
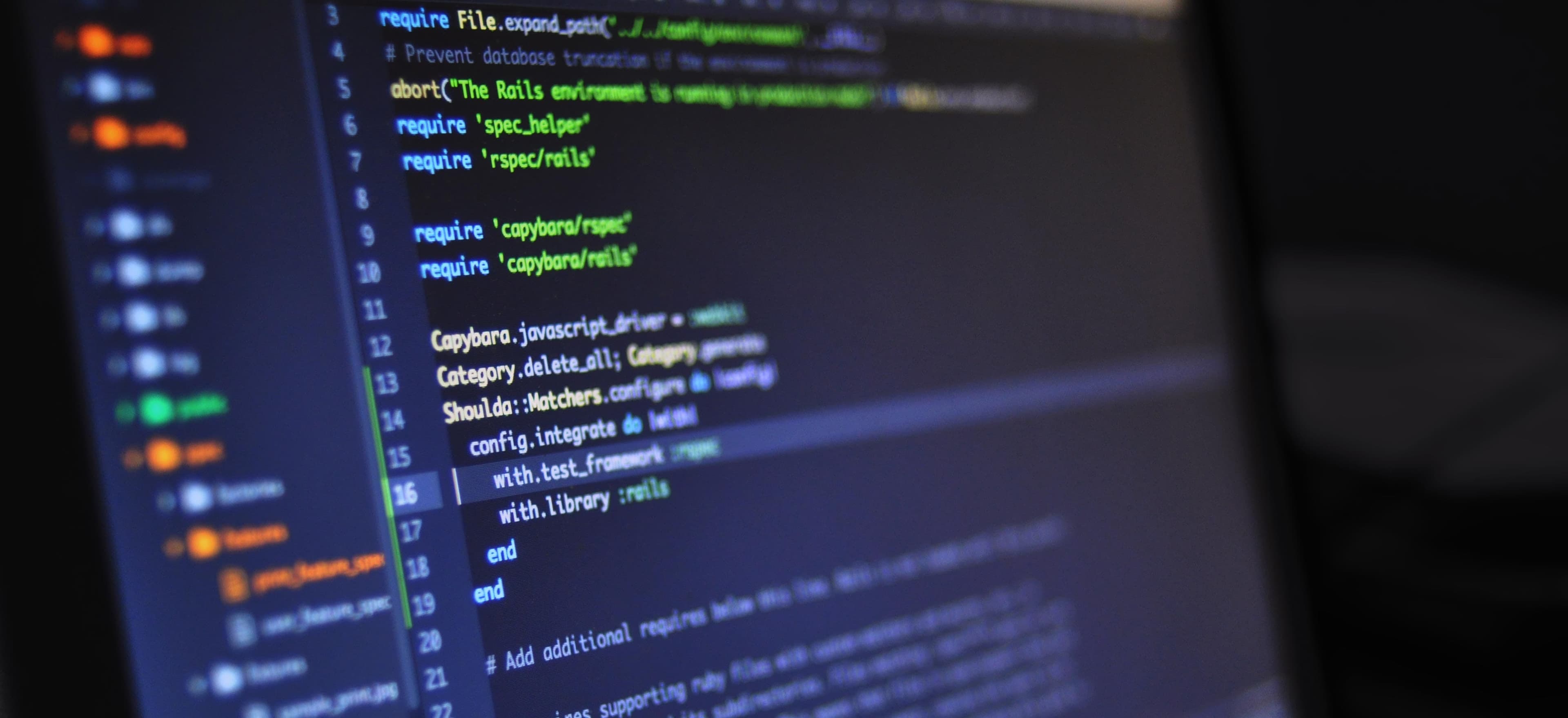
- Published on
Securing Atomic Operations on Volatile Fields: A Guide
In the world of multithreading in Java, atomic operations and volatile fields are key concepts. They help ensure that threads can safely read and write shared variables without encountering race conditions or inconsistent states. In this post, we'll delve into the use of volatile fields for securing atomic operations and explore best practices for ensuring thread safety in Java.
Understanding Atomic Operations and Volatile Fields
Before we dive into securing atomic operations on volatile fields, let's take a moment to understand what these concepts mean.
Atomic Operations
In the context of multithreading, an operation is considered atomic if it appears to occur instantaneously to the rest of the system. In other words, there is no chance of interference from other threads when an atomic operation is being performed. In Java, the java.util.concurrent.atomic
package provides classes such as AtomicInteger
, AtomicLong
, and AtomicReference
that facilitate atomic operations on primitive types and references.
Volatile Fields
In Java, the volatile
keyword is used to mark a variable as volatile. When a field is declared as volatile, it ensures that any read or write operation on that variable is atomic and all threads see the most up-to-date value of the variable.
Securing Atomic Operations on Volatile Fields
Now, let's discuss how we can use volatile fields to secure atomic operations. Imagine a scenario where multiple threads are accessing and updating a shared variable. We want to ensure that these operations are atomic and that the latest value of the variable is always visible to all threads.
Declaring a Volatile Field
To secure atomic operations, we can declare the shared variable as volatile. Here's an example of how to declare a volatile field in Java:
public class SharedResource {
private volatile int counter;
// Other methods and fields
}
In this example, the counter
field is declared as volatile, ensuring that all read and write operations on it are atomic.
Ensuring Atomic Updates
Let's consider a scenario where we need to increment the volatile counter in a thread-safe manner. We can achieve this by using the AtomicInteger
class, which provides atomic operations for incrementing and updating integers.
import java.util.concurrent.atomic.AtomicInteger;
public class SharedResource {
private AtomicInteger counter = new AtomicInteger(0);
public void incrementCounter() {
counter.incrementAndGet();
}
// Other methods
}
By using AtomicInteger
instead of a volatile int, we ensure that the increment operation is atomic and thread-safe without the need for explicit synchronization.
Benefits of Using Volatile Fields for Atomic Operations
Using volatile fields for atomic operations offers several benefits:
- Simplified Code: By using volatile fields, we can achieve atomicity without the need for explicit locking or synchronization.
- Improved Performance: Volatile fields have lower overhead compared to using synchronized blocks, leading to better performance in certain scenarios.
Best Practices for Securing Atomic Operations
While using volatile fields for atomic operations can be powerful, it's essential to follow best practices to ensure thread safety and avoid common pitfalls.
Choose the Right Data Structure
When dealing with complex data structures or composite operations, consider using classes from the java.util.concurrent.atomic
package, such as AtomicInteger
or AtomicReference
, to ensure atomicity without the need for explicit synchronization.
Mind the Visibility Guarantees
While volatile fields ensure atomic reads and writes, they do not provide atomicity for compound actions involving multiple fields. It's crucial to understand the visibility guarantees of volatile fields and use them appropriately in the context of your multithreaded application.
Consider Using Lock-Free Algorithms
In scenarios where fine-grained control over synchronization is required, consider using lock-free algorithms and data structures offered by the java.util.concurrent
package. These provide high concurrency and can be more efficient than traditional locking mechanisms in certain use cases.
Avoid Misusing Volatile
It's important to use volatile fields judiciously and avoid overreliance on them for complex synchronization requirements. In some cases, using explicit locking mechanisms such as synchronized blocks or ReentrantLock may be more appropriate for ensuring thread safety.
Wrapping Up
Securing atomic operations on volatile fields is essential for ensuring thread safety and preventing race conditions in multithreaded Java applications. By understanding the principles of atomic operations, leveraging volatile fields, and following best practices for securing atomic operations, developers can build robust and efficient multithreaded applications.
In this post, we've explored the use of volatile fields for securing atomic operations, discussed best practices for ensuring thread safety, and highlighted the benefits of using atomic classes for managing shared state in a multithreaded environment.
By incorporating these concepts and best practices into your Java development workflow, you can write concurrent code that is both efficient and reliable.
Remember, understanding the nuances of multithreading in Java and applying best practices for securing atomic operations is essential for building high-performance, scalable, and thread-safe applications.
Start incorporating these practices into your code today to ensure a smoother and more reliable multithreading experience in Java.
For further reading on the topic of atomic operations and volatile fields, consider checking out Java Concurrency in Practice, a comprehensive guide to writing concurrent programs in Java.
Happy coding!