Tackling Scroll Issues in SWT ScrolledComposite
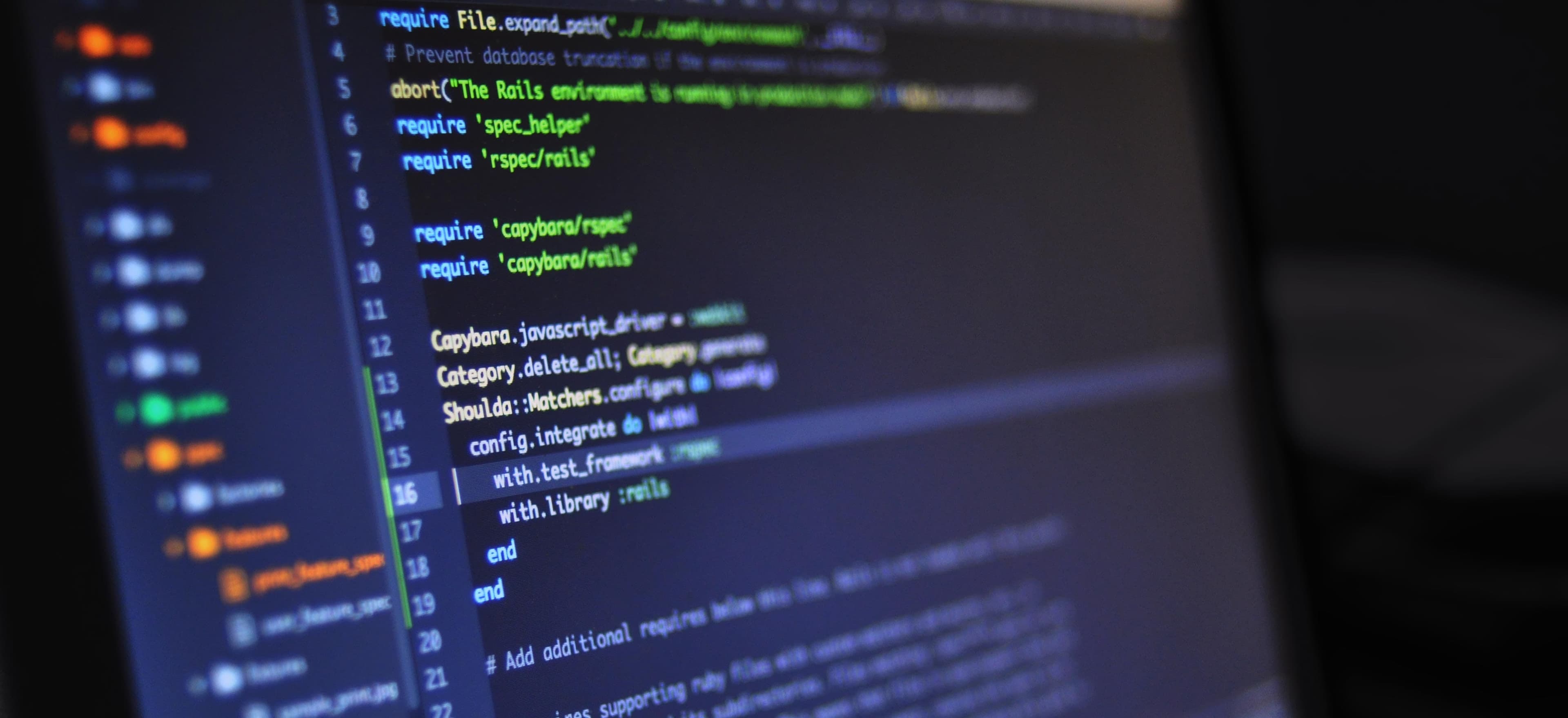
- Published on
Tackling Scroll Issues in SWT ScrolledComposite
Scrolling is a common requirement in user interfaces to handle content that overflows beyond the available visible area. In Java applications, the Standard Widget Toolkit (SWT) provides the ScrolledComposite
class to facilitate scrolling functionality. However, developers often encounter various issues when implementing and customizing scrolling behavior in SWT applications.
In this article, we will discuss common scroll issues that developers may face when working with ScrolledComposite
in SWT and explore effective strategies to address them.
Understanding ScrolledComposite
The ScrolledComposite
class in SWT provides a scrollable area that can contain a single child control. It automatically adds scroll bars when the child control's size exceeds the client area's available space. The ScrolledComposite
widget simplifies the implementation of scrolling functionality for developers working with SWT-based user interfaces.
Common Scroll Issues and Solutions
1. Incorrect Child Control Sizing
Issue: The child control within the ScrolledComposite
may not exhibit the expected behavior in terms of sizing, resulting in improper rendering and layout.
Solution: Set ScrolledComposite
content's minimum size using ScrolledComposite#setMinSize(int, int)
to ensure accurate sizing of the child control. This allows the ScrolledComposite
to determine when to display scroll bars based on the specified minimum size.
ScrolledComposite scrolledComposite = new ScrolledComposite(parent, SWT.V_SCROLL | SWT.H_SCROLL);
Composite content = new Composite(scrolledComposite, SWT.NONE);
content.setLayout(new GridLayout(1, true));
// Add child controls to the content composite
scrolledComposite.setContent(content);
scrolledComposite.setMinSize(content.computeSize(SWT.DEFAULT, SWT.DEFAULT));
By setting the minimum size, the ScrolledComposite
adjusts the scrolling behavior based on the specified dimensions, ensuring proper sizing of the child control.
2. Failure to Update Scroll Bars
Issue: Changes to the content within the ScrolledComposite
do not trigger an update of the scroll bars, leading to a mismatch between the actual content size and the displayed scroll bars.
Solution: Call ScrolledComposite#setMinSize(int, int)
or ScrolledComposite#setContent(Control)
after making changes to the content to prompt the ScrolledComposite
to update the scroll bars based on the modified content size.
// Update content within the ScrolledComposite
// ...
// Trigger a refresh of the scroll bars
scrolledComposite.setMinSize(content.computeSize(SWT.DEFAULT, SWT.DEFAULT));
By updating the minimum size or resetting the content control, the ScrolledComposite
refreshes its scroll bars to accurately reflect the modified content dimensions.
3. Inconsistent Scroll Behavior
Issue: The scrolling behavior in the ScrolledComposite
may exhibit inconsistencies, such as lagging or choppy scrolling, impacting the user experience.
Solution: Optimize the performance of the ScrolledComposite
by reducing unnecessary layout calculations and minimizing the complexity of the child control's content. Additionally, consider utilizing virtual controls for large datasets to enhance the scrolling performance.
scrolledComposite.setExpandHorizontal(true);
scrolledComposite.setExpandVertical(true);
Enabling horizontal and vertical expansion for the ScrolledComposite
allows it to efficiently handle varying content sizes and ensures a smoother scrolling experience for the users.
4. Scroll Bar Customization
Issue: Customizing the appearance and behavior of scroll bars within the ScrolledComposite
to align with the application's design requirements can be challenging.
Solution: Utilize ScrollBar
listeners and SWT's advanced drawing capabilities to customize the scroll bars as per the desired specifications. This includes handling scroll events and utilizing custom-drawn scroll bars to achieve a consistent look and feel across the application.
scrolledComposite.getVerticalBar().addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
int selection = scrolledComposite.getVerticalBar().getSelection();
// Perform custom actions based on the scroll bar selection
}
});
By leveraging SelectionListener
and PaintListener
interfaces, developers can customize the scroll bars' behavior and appearance, ensuring seamless integration with the application's visual style.
Closing the Chapter
In this article, we have explored common scroll issues encountered when working with ScrolledComposite
in SWT-based Java applications. By understanding these challenges and implementing the recommended solutions, developers can effectively address scroll-related issues and deliver a seamless scrolling experience to users.
Remember, when working with ScrolledComposite
, it's crucial to manage the content's sizing, update scroll bars appropriately, optimize performance, and customize scroll bars to align with the application's design. By mastering these strategies, developers can overcome scroll-related challenges and enhance the overall usability of their SWT-based Java applications.