Overcoming Challenges with Android's Background Services
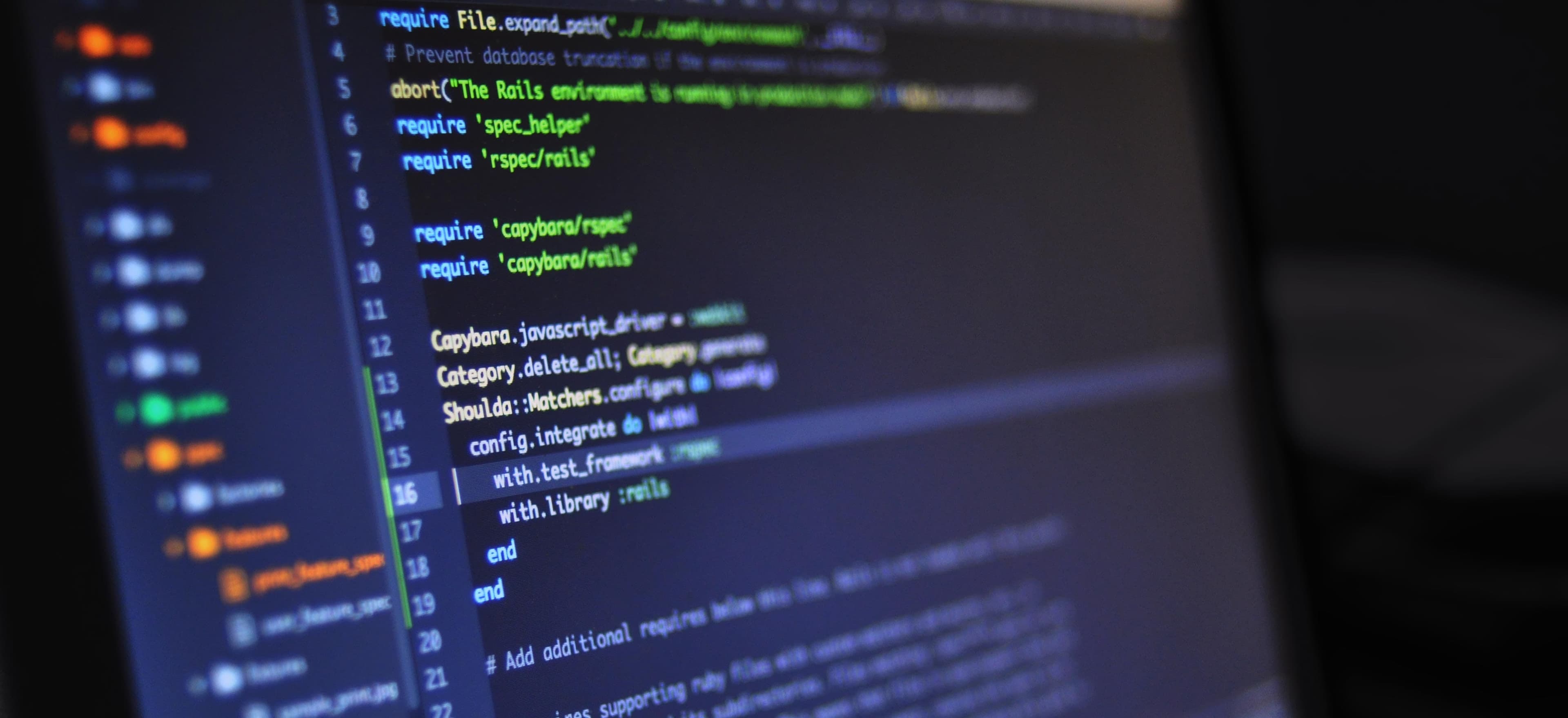
- Published on
Overcoming Challenges with Android's Background Services
When developing an Android application, implementing background services is often essential for performing tasks that should continue even when the app is not in the foreground. However, working with background services can be challenging due to the need to manage resources efficiently, handle different Android versions, and ensure the service runs reliably. In this article, we'll explore the common challenges faced when working with Android's background services and discuss the best practices to overcome them.
Understanding Android Background Services
Before delving into the challenges and their solutions, let's ensure a clear understanding of what background services are in the context of Android development. Background services are components that run in the background to perform long-running operations without a user interface. These operations could include playing music, fetching data from the internet, or performing periodic syncs.
Challenge 1: Battery Consumption
One of the primary concerns when working with background services is the impact on device battery life. Inefficient background services can drain the battery quickly, leading to a poor user experience and negative app reviews.
Solution:
To mitigate excessive battery consumption, it's crucial to optimize the execution of background services. This can be achieved by using the JobScheduler
for deferred execution of tasks, which allows the system to batch and optimize work to preserve battery life. Additionally, utilizing WorkManager
can help in managing deferrable, asynchronous tasks, ensuring that they execute reliably when the app is in the background.
// Example of scheduling work with WorkManager
OneTimeWorkRequest workRequest = new OneTimeWorkRequest.Builder(MyWorker.class)
.setInitialDelay(10, TimeUnit.MINUTES)
.build();
WorkManager.getInstance(context).enqueue(workRequest);
By utilizing these APIs, developers can effectively minimize battery consumption by efficiently scheduling and managing background tasks.
Challenge 2: Compatibility Across Android Versions
Another challenge arises from the fragmentation of Android devices across different OS versions. Background service behavior and constraints can vary between versions, requiring developers to handle these differences strategically.
Solution:
To address compatibility issues, leveraging the JobIntentService
is a recommended approach. JobIntentService
is a compatibility library that provides the same functionality as IntentService
but uses the JobScheduler
on Android 5.0 (API level 21) and higher, ensuring compatibility and consistent behavior across various Android versions.
// Example of creating a JobIntentService
public class MyJobIntentService extends JobIntentService {
// ... Service implementation
}
By utilizing JobIntentService
, developers can maintain consistent behavior of background services across different Android versions, simplifying the development process.
Challenge 3: Reliability and Lifecycle Management
Ensuring the reliability of background services, along with proper lifecycle management, is crucial for maintaining a seamless user experience. Background services should gracefully handle different lifecycle events and continue running efficiently without being interrupted.
Solution:
To address reliability and lifecycle management, implementing foreground services for high-priority tasks is recommended. Foreground services display a notification to the user, signifying that the app is performing a task in the background, thereby reducing the likelihood of the system terminating the service.
Additionally, utilizing the JobScheduler
or WorkManager
for periodic and deferred tasks ensures that the tasks are executed reliably and efficiently, even when the app is not actively in use.
// Example of starting a foreground service
Intent serviceIntent = new Intent(context, MyForegroundService.class);
ContextCompat.startForegroundService(context, serviceIntent);
By prioritizing tasks as foreground services and utilizing modern APIs for background task scheduling, developers can ensure the reliability and efficient lifecycle management of background services.
In Conclusion, Here is What Matters
In conclusion, conquering the challenges of Android's background services involves optimizing resource usage to minimize battery consumption, ensuring compatibility across diverse Android versions, and prioritizing reliability and lifecycle management. By employing modern APIs such as JobScheduler
, WorkManager
, and JobIntentService
, developers can overcome these challenges and deliver a robust and efficient background service experience in their Android applications.
Developers must strive to keep abreast of best practices in background service management and leverage the evolving Android APIs to ensure optimal performance and user satisfaction.
By incorporating these best practices, developers can build Android applications with background services that not only adhere to standards but also provide an exceptional user experience.
Considering the significance of optimizing battery consumption, understanding compatibility across Android versions, and streamlining lifecycle management, addressing these facets is essential. Therefore, diligently employing appropriate tools and techniques while developing Android background services is indispensable. Leveraging modern APIs and adhering to best practices ensures that these background services function seamlessly, ultimately enriching the user experience.
Should you be interested in diving deeper into Android's background services, you can refer to the Android Developers documentation on Managing Jobs and Creating Services. These resources offer valuable insights and actionable guidance for efficient management of background services in Android applications.