Master Your Data: Tips for Perfect Rolling Averages
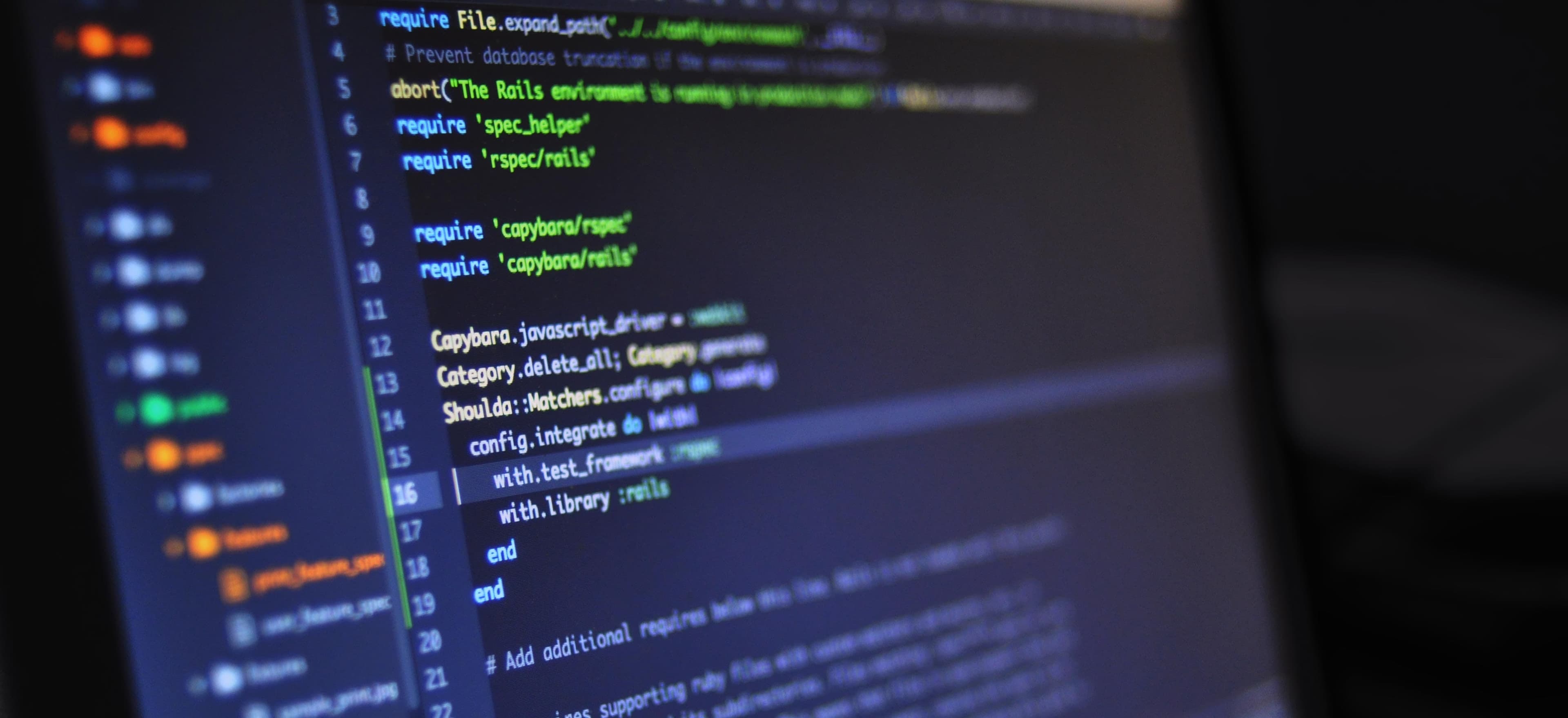
- Published on
Master Your Data: Tips for Perfect Rolling Averages
As a Java developer, you must often deal with large sets of data and perform various calculations on them. One common operation is calculating rolling averages, which are essential in smoothing out data and identifying trends over time. In this post, we will explore the concept of rolling averages and learn how to compute them efficiently in Java.
Understanding Rolling Averages
A rolling average, also known as a moving average, is a statistical calculation used to analyze data points by creating a series of averages of different subsets of the full data set. This technique is particularly useful for time series data, where it helps to identify trends and patterns by minimizing the impact of short-term fluctuations.
One of the key advantages of using rolling averages is their ability to smooth out irregularities and highlight long-term trends, making them a valuable tool in financial analysis, signal processing, and various other fields.
Computing Rolling Averages in Java
Let's dive into the Java code and explore how we can efficiently compute rolling averages using a simple and effective approach.
Using a Circular Buffer
One efficient way to compute rolling averages in Java is by using a circular buffer. A circular buffer is a data structure that uses a fixed-size array and maintains a pointer to the current position in the array. As new data points come in, they overwrite the oldest points in a cyclic manner.
Here's a simple implementation of a circular buffer in Java:
public class CircularBuffer {
private double[] buffer;
private int size;
private int index = 0;
private double sum = 0;
public CircularBuffer(int size) {
this.size = size;
buffer = new double[size];
}
public void add(double value) {
sum -= buffer[index];
buffer[index] = value;
sum += value;
index = (index + 1) % size;
}
public double getAverage() {
return sum / size;
}
}
In this implementation, we define a CircularBuffer
class that maintains an array to store the data points, along with a sum
variable to keep track of the sum of the elements in the buffer. The add
method adds a new value to the buffer while updating the sum and overwriting the oldest value. The getAverage
method then returns the average of the values in the buffer.
Computing Rolling Averages
With the circular buffer in place, we can now compute rolling averages using the data points from our input. Let's assume we have an array of data points represented by the data
array. We can then compute the rolling averages as follows:
public class RollingAverage {
public static void main(String[] args) {
double[] data = {1.0, 2.0, 3.0, 4.0, 5.0, 6.0, 7.0, 8.0, 9.0, 10.0};
int windowSize = 3;
CircularBuffer buffer = new CircularBuffer(windowSize);
for (double value : data) {
buffer.add(value);
System.out.println("Rolling Average: " + buffer.getAverage());
}
}
}
In this example, we initialize a CircularBuffer
with a window size of 3 and then iterate through our data points, adding each value to the buffer and printing the rolling average at each step.
Benefits of Using Circular Buffer for Rolling Averages
Using a circular buffer for computing rolling averages offers several advantages:
-
Constant Time Complexity: The circular buffer approach allows us to compute rolling averages in constant time, regardless of the window size. This is particularly beneficial when dealing with large datasets, as it ensures efficient performance.
-
Minimal Memory Footprint: By maintaining a fixed-size array, the circular buffer approach minimizes the memory footprint, making it suitable for memory-constrained environments.
-
Easily Adaptable: The implementation can be easily extended to accommodate additional functionalities, such as calculating other statistical metrics or handling different data types.
Key Takeaways
In this post, we've explored the concept of rolling averages and learned how to efficiently compute them in Java using a circular buffer approach. By understanding the principles behind rolling averages and leveraging the power of Java's data structures and iteration, we can effectively analyze and interpret large sets of data.
As you continue to work with data in your Java projects, consider the insights gained from this post to master the art of computing rolling averages and enhance your data analysis capabilities.
By mastering rolling averages in Java, you can gain a competitive edge in developing data-driven applications and performing insightful analytics.
Start applying these techniques in your projects and elevate your data computation and analysis prowess with the power of rolling averages in Java.