Stream Pitfalls: Why Your Java Count Might Be Off
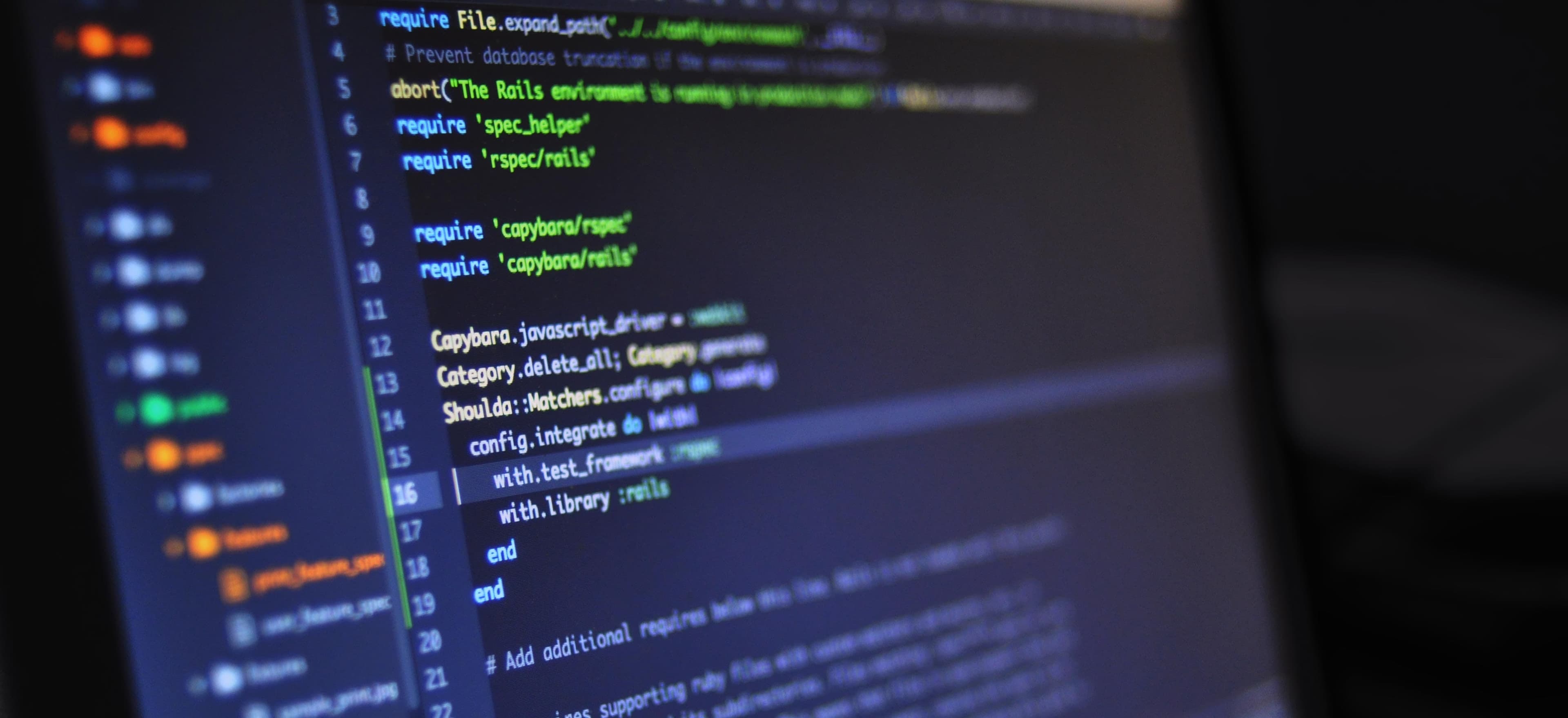
- Published on
Stream Pitfalls: Why Your Java Count Might Be Off
When working with Java streams, it's easy to fall into traps that can lead to unexpected results. One common issue that developers encounter is an incorrect count when using the count()
method. In this blog post, we'll explore some common pitfalls that can cause your Java count to be off and how to avoid them.
Understanding the count()
Method
In Java, the count()
method is used to count the number of elements in a stream. It returns the count as a long
value. This method is often used in combination with other stream operations to perform calculations or to determine the size of a stream.
Let's start with an example:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
long count = names.stream().count();
System.out.println(count); // Output: 4
In this example, we have a list of names, and we create a stream from the list using the stream()
method. We then call the count()
method to count the number of elements in the stream, which is 4 in this case.
Pitfall 1: Forgetting Stream Terminal Operations
One common pitfall when using the count()
method is forgetting to call a terminal operation on the stream before invoking count()
. Streams in Java are lazy, meaning that intermediate operations are only executed when a terminal operation is invoked. If you forget to call a terminal operation, the intermediate operations will not be executed, and the count will be zero.
Let's take a look at an example:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
long count = names.stream().filter(name -> name.startsWith("A")).count();
System.out.println(count); // Output: 0
In this example, we have a list of names, and we create a stream from the list. We then apply a filter operation to the stream to retain only the names that start with the letter "A". However, we forgot to call a terminal operation after the filter, so the count()
method is called on a stream with no elements, resulting in a count of 0.
To avoid this pitfall, always remember to include a terminal operation, such as forEach
, collect
, or count
, to ensure that the stream pipeline is executed.
Pitfall 2: Reusing Streams
Another common pitfall is attempting to reuse a stream after a terminal operation has been invoked. Once a terminal operation has been called on a stream, the stream is considered consumed and cannot be reused. If you attempt to reuse a consumed stream, it will result in an IllegalStateException
.
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
Stream<String> nameStream = names.stream().filter(name -> name.startsWith("A"));
long count = nameStream.count(); // Terminal operation
long otherCount = nameStream.count(); // IllegalStateException
In this example, we create a stream from the list of names and apply a filter operation to retain only the names that start with the letter "A". After calling the count()
method as a terminal operation, the stream nameStream
is consumed. If we attempt to call count()
again on the same stream, it will result in an IllegalStateException
.
To avoid this pitfall, create a new stream from the source data or recompute the stream pipeline if you need to perform additional operations.
Pitfall 3: Infinite Streams
In Java, it's possible to create infinite streams using methods like Stream.generate()
or Stream.iterate()
. When working with infinite streams, calling count()
can lead to unexpected results or even an infinite loop.
Stream<Integer> infiniteStream = Stream.iterate(0, i -> i + 1);
long count = infiniteStream.count(); // Infinite loop
In this example, we create an infinite stream of integers starting from 0 and incrementing by 1. When we attempt to call count()
on the infinite stream, it will lead to an infinite loop because the count()
method tries to traverse the entire stream to count the elements.
When working with infinite streams, consider using alternative methods such as limit()
to reduce the size of the stream before calling count()
.
My Closing Thoughts on the Matter
When working with Java streams, it's essential to be mindful of potential pitfalls that can lead to incorrect counts. Always ensure that you invoke a terminal operation on the stream, avoid reusing consumed streams, and be cautious when working with infinite streams. By understanding and addressing these pitfalls, you can confidently use the count()
method and avoid unexpected results in your Java code.
In conclusion, by paying close attention to the behavior of Java streams, you can ensure that your code produces accurate results, particularly when using the count()
method. As always, staying aware of best practices and potential issues will help you to write cleaner, more dependable Java code.
For further reading on the topic of Java streams and how to avoid common pitfalls, please refer to Oracle's official documentation for a comprehensive guide.
Remember, always keep in mind the intricacies of Java streams, and ensure that your code not only performs well but also remains easy to maintain.