How to Prioritize and Manage Stakeholder Requests
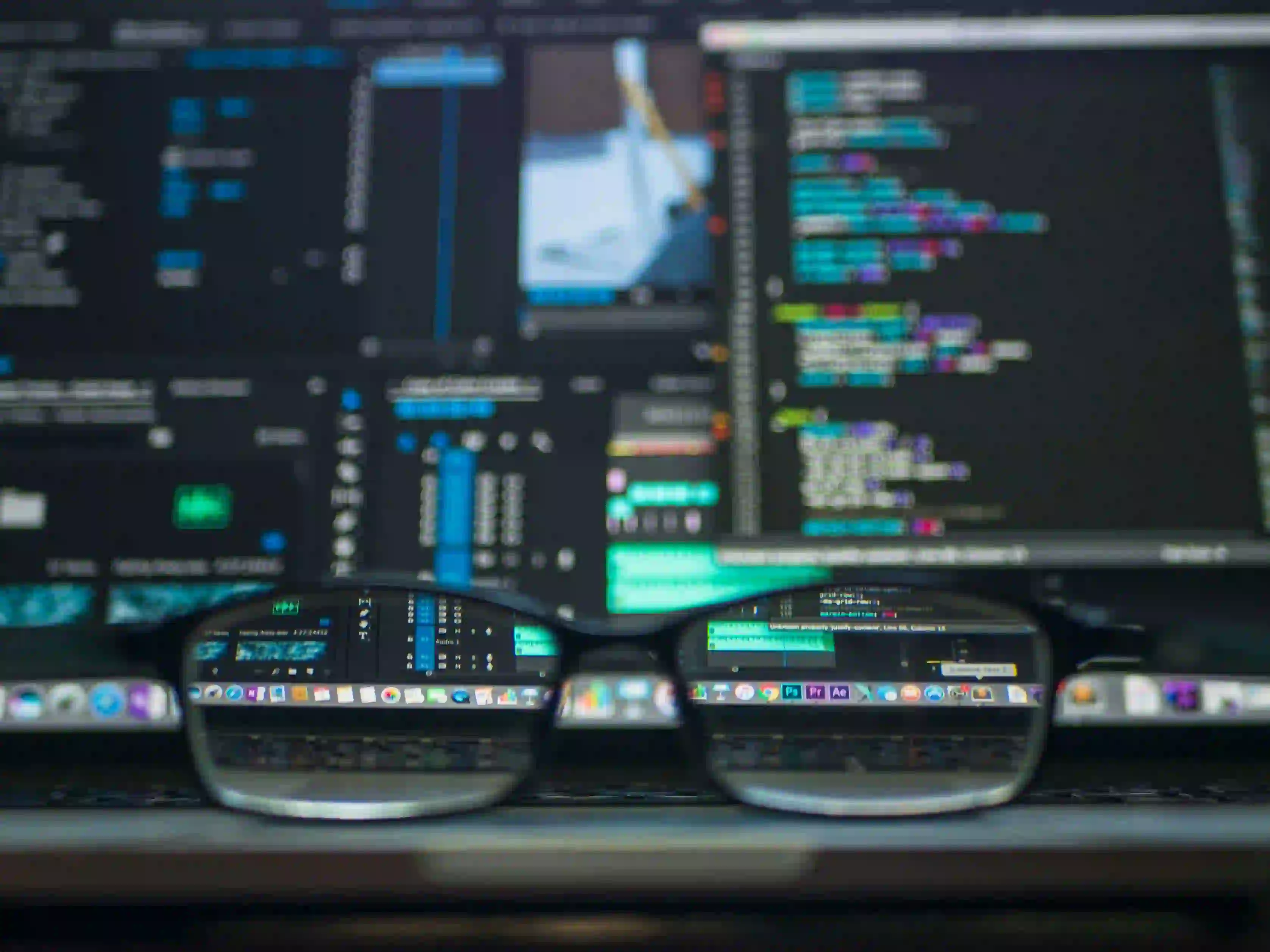
Understanding Stakeholder Management in Java Projects
Stakeholder management is a crucial aspect of Java development projects. Balancing the needs and requests of various stakeholders can be challenging, and it's essential to prioritize and manage these requests effectively to ensure the successful delivery of the project. In this article, we'll discuss some strategies for prioritizing and managing stakeholder requests in Java projects.
Importance of Stakeholder Management
Stakeholders, including clients, end users, project sponsors, and other team members, have a significant impact on the success of a project. Managing their requests and expectations is key to ensuring that the final product meets their needs and aligns with the project goals. Failing to manage stakeholder requests effectively can lead to scope creep, missed deadlines, and dissatisfaction among the project's key players.
Prioritizing Stakeholder Requests
Prioritizing stakeholder requests is essential to ensure that the most critical needs are addressed first. Here are some strategies for prioritizing stakeholder requests in Java projects:
1. Impact and Effort Analysis
Evaluate each stakeholder request based on its potential impact on the project and the effort required to implement it. High-impact, low-effort requests should be prioritized, as they can deliver significant value with minimal investment of resources.
2. Business Value
Consider the business value of each stakeholder request. Requests that align closely with the project's objectives and contribute to the overall success of the product should be given higher priority.
3. Risk Assessment
Assess the risks associated with each stakeholder request. Requests that mitigate potential risks or address critical issues should be prioritized to ensure the stability and security of the project.
4. Time Sensitivity
Take into account the time sensitivity of stakeholder requests. Urgent requests that require immediate attention should be handled promptly to prevent any project delays or negative impacts on the project timeline.
5. Stakeholder Collaboration
Engage in open communication with stakeholders to gain a better understanding of their needs and expectations. Collaborative discussions can help in identifying the most critical requests and aligning them with the project's goals.
Managing Stakeholder Requests in Java Projects
Once stakeholder requests are prioritized, it's crucial to manage them effectively throughout the project lifecycle. Here are some tips for managing stakeholder requests in Java projects:
1. Use Case Management Tools
Utilize dedicated use case management tools and issue tracking systems, such as JIRA, to capture and track stakeholder requests. These tools provide a centralized platform for managing and prioritizing requests based on their impact and urgency.
2. Agile Methodologies
Adopt agile methodologies, such as Scrum or Kanban, to iteratively address stakeholder requests. Agile frameworks allow for flexibility and responsiveness to changing stakeholder needs, enabling you to deliver value quickly and efficiently.
3. Continuous Integration and Deployment
Implement continuous integration and deployment (CI/CD) pipelines to swiftly incorporate stakeholder-requested features and enhancements into the project. CI/CD practices streamline the process of delivering frequent updates and responding to stakeholder feedback in a timely manner.
Example: Prioritizing and Managing Stakeholder Requests in Java
Let's consider a practical example of how to prioritize and manage stakeholder requests in a Java project using the Spring Boot framework.
// Example of prioritizing and managing stakeholder requests in Java
@RestController
public class FeatureController {
@Autowired
private FeatureService featureService;
// Endpoint to handle stakeholder requests for a specific feature
@GetMapping("/features/{id}")
public ResponseEntity<Feature> getFeature(@PathVariable Long id) {
// Retrieve the requested feature from the database
Feature feature = featureService.getFeatureById(id);
if (feature != null) {
return ResponseEntity.ok(feature);
} else {
return ResponseEntity.notFound().build();
}
}
// Endpoint to submit new stakeholder requests for features
@PostMapping("/features")
public ResponseEntity<Feature> submitFeatureRequest(@RequestBody FeatureRequest request) {
// Process the stakeholder request and add it to the feature backlog
Feature newFeature = featureService.processFeatureRequest(request);
return ResponseEntity.status(HttpStatus.CREATED).body(newFeature);
}
}
In this example, the FeatureController
manages stakeholder requests for features in a Java application. By employing Java's Spring Boot framework, we can easily handle incoming requests and process stakeholder inputs. The FeatureService
collaborates with the controller to prioritize and manage stakeholder requests, ensuring that high-impact, business-critical requests are addressed promptly.
Final Thoughts
Prioritizing and managing stakeholder requests is essential for the success of Java projects. By evaluating the impact, business value, risks, and time sensitivity of stakeholder requests, and using dedicated tools and agile methodologies, Java developers can effectively address stakeholder needs while maintaining project momentum. Incorporating stakeholder collaboration and employing CI/CD practices further enhances the management of stakeholder requests, contributing to a successful project delivery.
Effective stakeholder management in Java projects is not only about writing code, but also about understanding the needs of the project’s key players. With the right approach and tools, Java developers can navigate stakeholder requests and deliver exceptional outcomes.