Mastering API Tests: Curl Your Way to RESTful Success!
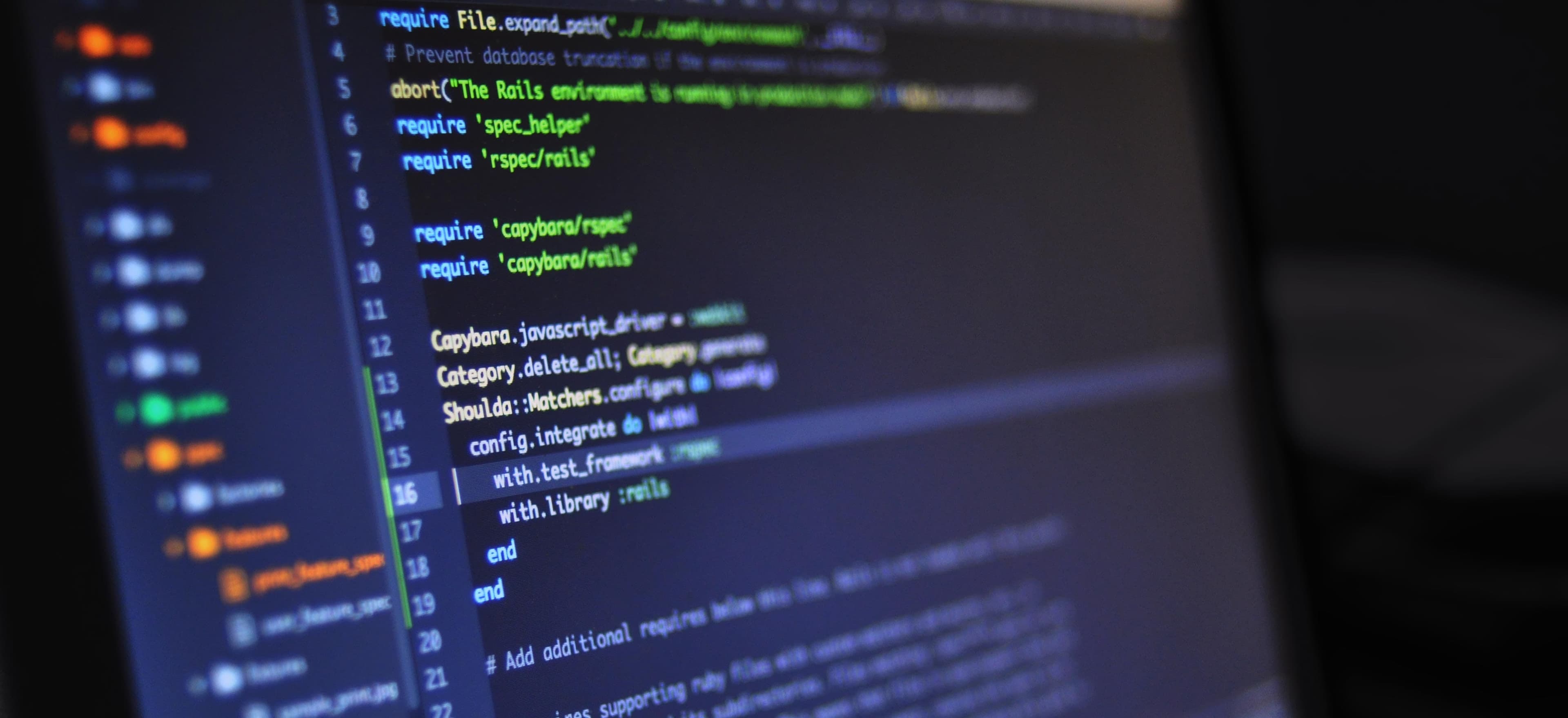
- Published on
Mastering API Tests: Curl Your Way to RESTful Success!
API testing has become an essential part of software development, ensuring that the core functionality of an application is reliable, secure, and performing as expected. With the rise of RESTful APIs, testing has become even more critical as APIs are the backbone of many modern applications. In this blog post, we will delve into the world of API testing, specifically focusing on using the powerful command-line tool, Curl, to master API tests.
Why API Testing is Crucial
Before we dive into the nitty-gritty of API testing with Curl, let's briefly discuss why API testing is crucial. In the era of interconnected systems and microservices, APIs serve as the communication channels between different software components. Any flaws or unpredictability in APIs can spell disaster for the entire application. API testing ensures that the endpoints, request-response mechanisms, data formats, and authentication methods are all functioning correctly.
Introducing Curl - The Command-Line Swiss Army Knife
Curl is a command-line tool for transferring data with URL syntax, supporting various protocols including HTTP and HTTPS. It is a versatile tool that facilitates making requests to servers, making it an excellent choice for testing and debugging APIs. Curl is available on most operating systems and is highly scriptable, enabling automation of API tests.
Setting the Stage: An Example REST API
To demonstrate the power of Curl in API testing, let's consider a simple RESTful API for managing a library. The API provides endpoints for adding a new book, retrieving a book by its ID, and listing all books in the library. We will use Curl to interact with these endpoints and perform various tests.
class LibraryApiTest {
private final String baseUrl = "http://example.com/api/library";
@Test
public void testAddNewBook() {
// Curl command to add a new book
// curl -X POST -d '{"title":"The Great Gatsby","author":"F. Scott Fitzgerald"}' -H "Content-Type: application/json" http://example.com/api/library/books
}
@Test
public void testGetBookById() {
// Curl command to retrieve a book by ID
// curl http://example.com/api/library/books/123
}
@Test
public void testListAllBooks() {
// Curl command to list all books
// curl http://example.com/api/library/books
}
}
In the above code snippet, we have a simple JUnit test class that interacts with the library API using Curl commands as comments. We will now explore each of these tests in detail, showcasing how Curl can be used for various aspects of API testing.
Testing Adding a New Book
The testAddNewBook()
method simulates adding a new book to the library using the POST method. The corresponding Curl command sends a POST request with the book's details in JSON format to the /books
endpoint. This test ensures that the API can accept new book entries and responds appropriately.
Testing Retrieving a Book by ID
In the testGetBookById()
method, we retrieve a book by its ID using a GET request. The Curl command makes a simple GET request to the /books/{id}
endpoint to fetch the details of a specific book. This test verifies that the API can successfully retrieve book details based on the provided ID.
Testing Listing All Books
The testListAllBooks()
method focuses on listing all the books in the library. The corresponding Curl command sends a GET request to the /books
endpoint to retrieve a list of all books. This test ensures that the API can return a collection of books without any errors.
Advanced API Testing with Curl
So far, we have seen how Curl can be used for basic API testing scenarios. However, Curl's capabilities extend beyond simple requests. Here are some advanced techniques for API testing with Curl:
Sending Authentication Headers
@Test
public void testAuthenticatedRequest() {
// Curl command with basic authentication
// curl -u username:password http://example.com/api/secure-endpoint
}
The testAuthenticatedRequest()
method demonstrates how to include authentication credentials in a Curl request. This is crucial for testing secure endpoints that require authentication.
Emulating Different HTTP Methods
@Test
public void testUpdateBook() {
// Curl command to update a book using the PUT method
// curl -X PUT -d '{"title":"Updated Title"}' -H "Content-Type: application/json" http://example.com/api/library/books/123
}
@Test
public void testDeleteBook() {
// Curl command to delete a book using the DELETE method
// curl -X DELETE http://example.com/api/library/books/123
}
The testUpdateBook()
and testDeleteBook()
methods showcase using Curl to emulate PUT and DELETE requests, demonstrating how to update and delete resources through API testing.
Handling Response Data
@Test
public void testHandleResponse() {
// Curl command to save the response to a file
// curl -o response.json http://example.com/api/library/books
}
The testHandleResponse()
method illustrates saving the API response to a file using Curl, enabling further analysis and validation of the returned data.
Lessons Learned
In this post, we have explored the significance of API testing and how Curl can be leveraged to conduct comprehensive API tests. By using Curl for API testing, developers gain a powerful tool for ensuring the reliability and functionality of their RESTful APIs. With its simplicity and versatility, Curl proves to be an indispensable companion in the quest for mastering API tests.
With the demonstrated examples and advanced techniques, developers can harness the full potential of Curl in API testing, ultimately contributing to the delivery of robust and high-quality software applications.
Now it's your turn to Curl your way to RESTful success in API testing!
Remember to practice and experiment with Curl commands for various API testing scenarios to become a proficient API testing maestro.
Happy testing!