Mastering Java: Unmodifiable Collections Made Easy!
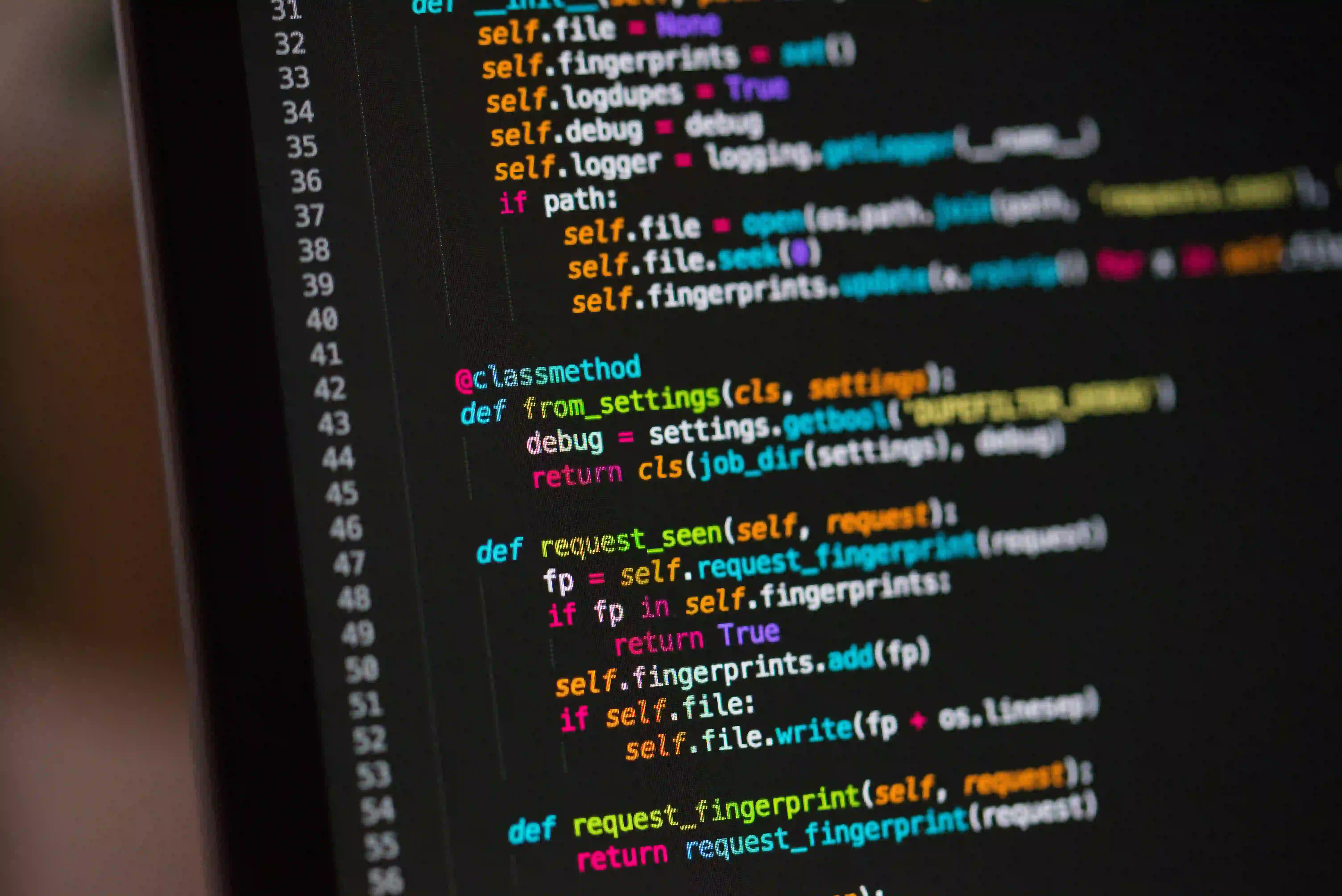
Mastering Java: Unmodifiable Collections Made Easy!
When working with Java, it's vital to ensure the integrity of your data structures. In many cases, you need to prevent the modification of collections, safeguarding them from accidental or intentional changes. This is where unmodifiable collections come into play. In this blog post, we'll explore the concept of unmodifiable collections in Java and how to use them effectively in your code.
What are Unmodifiable Collections?
In Java, unmodifiable collections are exactly what they sound like: collections that cannot be modified after they are created. This means that once you create an unmodifiable collection, any attempt to modify it will result in an UnsupportedOperationException
. Unmodifiable collections are backed by the original collection, so any changes to the original collection are reflected in the unmodifiable collection.
Why Use Unmodifiable Collections?
Using unmodifiable collections is beneficial for several reasons:
- Data Integrity: By creating unmodifiable collections, you can ensure that the data remains unchanged throughout the program.
- Thread Safety: Unmodifiable collections provide a level of thread safety, as they cannot be modified once created.
- API Design: They are useful in API design to expose read-only views of collections, preventing clients from modifying the underlying data.
Now that we understand the importance of unmodifiable collections, let's dive into how to create and use them in Java.
Creating Unmodifiable Collections
Java provides a convenient way to create unmodifiable collections through the Collections
utility class. You can use the Collections.unmodifiableXXX
methods (where XXX
represents the type of collection) to obtain an unmodifiable view of a collection. Let's take a look at some examples:
Creating an Unmodifiable List
import java.util.Collections;
import java.util.List;
import java.util.ArrayList;
List<String> mutableList = new ArrayList<>();
mutableList.add("Java");
mutableList.add("is");
mutableList.add("awesome");
List<String> unmodifiableList = Collections.unmodifiableList(mutableList);
In this example, unmodifiableList
is an unmodifiable view of mutableList
. Any attempt to modify unmodifiableList
will result in an UnsupportedOperationException
.
Creating an Unmodifiable Map
import java.util.Collections;
import java.util.Map;
import java.util.HashMap;
Map<Integer, String> mutableMap = new HashMap<>();
mutableMap.put(1, "Java");
mutableMap.put(2, "is");
mutableMap.put(3, "awesome");
Map<Integer, String> unmodifiableMap = Collections.unmodifiableMap(mutableMap);
Similarly, unmodifiableMap
is an unmodifiable view of mutableMap
.
Creating an Unmodifiable Set
import java.util.Collections;
import java.util.Set;
import java.util.HashSet;
Set<String> mutableSet = new HashSet<>();
mutableSet.add("Java");
mutableSet.add("is");
mutableSet.add("awesome");
Set<String> unmodifiableSet = Collections.unmodifiableSet(mutableSet);
Here, unmodifiableSet
is an unmodifiable view of mutableSet
.
It's important to note that while the unmodifiable collection cannot be modified, the underlying original collection (mutableList
, mutableMap
, mutableSet
) can still be changed. Any changes to the original collection are reflected in the unmodifiable view.
Best Practices for Using Unmodifiable Collections
When using unmodifiable collections, there are a few best practices to keep in mind:
- Immutability: Consider making the elements within the collection immutable as well, especially if they are mutable objects. This ensures that the objects within the collection cannot be changed.
- Documentation: Clearly document in your code that a method or class returns an unmodifiable collection to avoid confusion for other developers.
- Defensive Copying: If you need to prevent the original collection from being modified, consider making a defensive copy of the collection before creating the unmodifiable view.
Working with Unmodifiable Collections
Now that we have created unmodifiable collections, let's explore how to work with them in our code.
Iterating Over an Unmodifiable Collection
List<String> unmodifiableList = Collections.unmodifiableList(new ArrayList<>(List.of("Java", "is", "awesome")));
for (String element : unmodifiableList) {
System.out.println(element);
}
In this example, we iterate over the unmodifiable list in the same way we would iterate over a regular list. The unmodifiable nature of the collection does not affect iteration.
Using Unmodifiable Collections in Method Signatures
public void processElements(List<String> elements) {
// ...
}
Using unmodifiable collections in method signatures clearly communicates to other developers that the method does not intend to modify the collection. It's a good practice to use unmodifiable collections when the method only requires read-only access to the data.
Stream Operations on Unmodifiable Collections
List<String> unmodifiableList = Collections.unmodifiableList(new ArrayList<>(List.of("Java", "is", "awesome")));
long count = unmodifiableList.stream().filter(s -> s.startsWith("a")).count();
You can perform stream operations on unmodifiable collections just like you would on mutable collections. The immutability of the collection does not hinder the use of stream operations.
The Closing Argument
In this post, we've delved into the world of unmodifiable collections in Java. We've learned what unmodifiable collections are, why they are important, how to create them, and best practices for using them. By using unmodifiable collections, you can ensure the integrity and immutability of your data structures, providing thread safety and clear API design.
Java's Collections
utility class makes it straightforward to create unmodifiable collections, empowering you to leverage their benefits in your code. As you continue to master Java, integrating unmodifiable collections into your development practices will enhance the robustness and security of your applications.
Unmodifiable collections are a valuable tool in the Java developer's arsenal, allowing for the creation of predictable and tamper-proof data structures. By mastering the use of unmodifiable collections, you can elevate the quality and reliability of your Java code. Start leveraging unmodifiable collections in your projects today for a more robust and secure codebase!