Maximizing Speed: Switching Between SPDY and HTTP in Netty
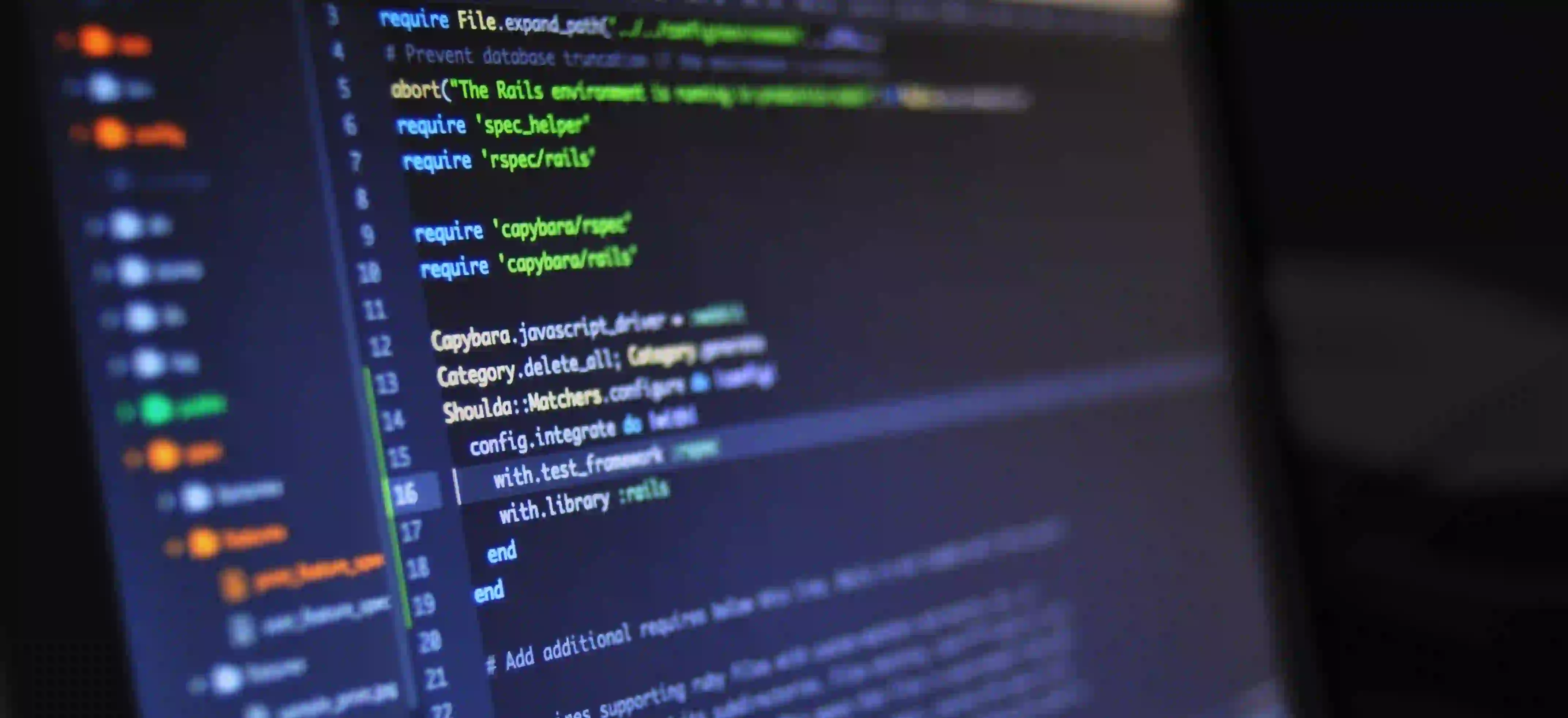
Maximizing Speed: Switching Between SPDY and HTTP in Netty
In today's digital landscape, speed is everything. For Java developers, ensuring speedy and efficient communication between clients and servers is paramount. This is where protocols like SPDY and HTTP come into play. In this article, we'll explore how to harness the power of both SPDY and HTTP in Netty, a highly extensible NIO (Non-blocking I/O) client-server framework.
Understanding SPDY and HTTP
SPDY
SPDY, pronounced "speedy," is a protocol developed by Google to address the performance limitations of HTTP. It aims to minimize latency through features such as multiplexing, header compression, and prioritization of requests. SPDY is the precursor to the HTTP/2 protocol, and many of its features have been incorporated into HTTP/2.
HTTP
Hypertext Transfer Protocol (HTTP) is the foundation of data communication on the World Wide Web. It is designed to enable communication between clients and servers, typically web browsers and web servers. While HTTP has undergone several iterations, its traditional version suffers from some performance limitations, which SPDY aims to overcome.
Introducing Netty
Netty is a high-performance network application framework that provides an event-driven architecture for rapid development of maintainable high-performance protocol servers and clients. It supports various transport protocols such as TCP, UDP, and SCTP, and integrates easily with third-party libraries.
Netty's extensibility and support for both SPDY and HTTP make it an ideal choice for building high-speed communication systems.
Adapting Netty for SPDY and HTTP
Setting Up Netty
To get started, we'll need to set up a basic Netty server that can handle both SPDY and HTTP requests. First, let's create a new Maven project and add the Netty dependency to the pom.xml
file:
<dependency>
<groupId>io.netty</groupId>
<artifactId>netty-all</artifactId>
<version>4.1.63.Final</version>
</dependency>
Creating a Server
Next, we'll implement a simple Netty server that can handle both SPDY and HTTP requests. Here's a basic example of how this can be achieved:
public class NettyServer {
public static void main(String[] args) {
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) {
ChannelPipeline p = ch.pipeline();
// Add handlers for SPDY and HTTP here
}
})
.option(ChannelOption.SO_BACKLOG, 128)
.childOption(ChannelOption.SO_KEEPALIVE, true);
ChannelFuture f = b.bind(8080).sync();
f.channel().closeFuture().sync();
} finally {
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
}
}
}
In the initChannel
method, we can add handlers for both SPDY and HTTP to the ChannelPipeline
. This allows the server to handle requests from both protocols simultaneously.
Handling SPDY Requests
When handling SPDY requests, we can use the spdy
package provided by Netty, which offers a range of classes and interfaces for working with the SPDY protocol.
For example, the following code demonstrates how to handle a SPDY request and send a response:
public class SpdyRequestHandler extends SimpleChannelInboundHandler<SpdySynStreamFrame> {
@Override
protected void channelRead0(ChannelHandlerContext ctx, SpdySynStreamFrame msg) {
// Handle the SPDY request
SpdySynStreamFrame response = createSpdyResponse(); // Create the response frame
ctx.writeAndFlush(response);
}
private SpdySynStreamFrame createSpdyResponse() {
// Create and configure the SPDY response frame
// Add headers, data, etc.
return responseFrame;
}
}
In this example, when the server receives a SPDY request, the channelRead0
method of the SpdyRequestHandler
class is invoked. Here, we can create a SPDY response frame and send it back to the client.
Handling HTTP Requests
Similarly, handling HTTP requests in Netty is straightforward. Netty provides the http
package, which includes classes and interfaces for working with HTTP.
Below is an example of how to handle an HTTP request and send a response:
public class HttpRequestHandler extends SimpleChannelInboundHandler<FullHttpRequest> {
@Override
protected void channelRead0(ChannelHandlerContext ctx, FullHttpRequest msg) {
// Handle the HTTP request
FullHttpResponse response = createHttpResponse(); // Create the HTTP response
ctx.writeAndFlush(response);
}
private FullHttpResponse createHttpResponse() {
// Create and configure the HTTP response
// Set status code, headers, content, etc.
return response;
}
}
In this example, the channelRead0
method of the HttpRequestHandler
class is invoked when the server receives an HTTP request. Here, we can create an HTTP response and send it back to the client.
Switching Between SPDY and HTTP
Now that we have handlers for both SPDY and HTTP requests, we can easily switch between the two protocols based on the incoming requests. For example, we can inspect the request headers to determine which protocol the client is using and route the request to the appropriate handler.
public class ProtocolSwitchHandler extends ChannelInboundHandlerAdapter {
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) {
if (msg instanceof HttpRequest) {
// Determine if the request is HTTP or SPDY
if (isSpdyRequest((HttpRequest) msg)) {
ctx.pipeline().addLast(new SpdyRequestHandler());
} else {
ctx.pipeline().addLast(new HttpRequestHandler());
}
ctx.pipeline().remove(this); // Remove the protocol switch handler
}
ctx.fireChannelRead(msg);
}
private boolean isSpdyRequest(HttpRequest request) {
// Logic to determine if the request is using SPDY
return isSpdy;
}
}
In this ProtocolSwitchHandler
class, we inspect the incoming request to determine whether it is a SPDY or HTTP request. Based on this determination, we add the appropriate request handler to the channel pipeline and remove the protocol switch handler.
A Final Look
In conclusion, Netty provides a robust framework for building high-performance servers that can handle both SPDY and HTTP requests. By leveraging Netty's extensibility and support for various protocols, Java developers can maximize the speed and efficiency of client-server communication.
Incorporating the examples discussed in this article, you can adapt your Netty server to seamlessly switch between SPDY and HTTP, catering to diverse client requirements. With speed being the essence of modern web communication, the ability to handle multiple protocols efficiently is a valuable asset for any network application.
To delve deeper into Netty and its capabilities, you can explore the official Netty documentation and experiment with various features to further enhance the performance and versatility of your applications.