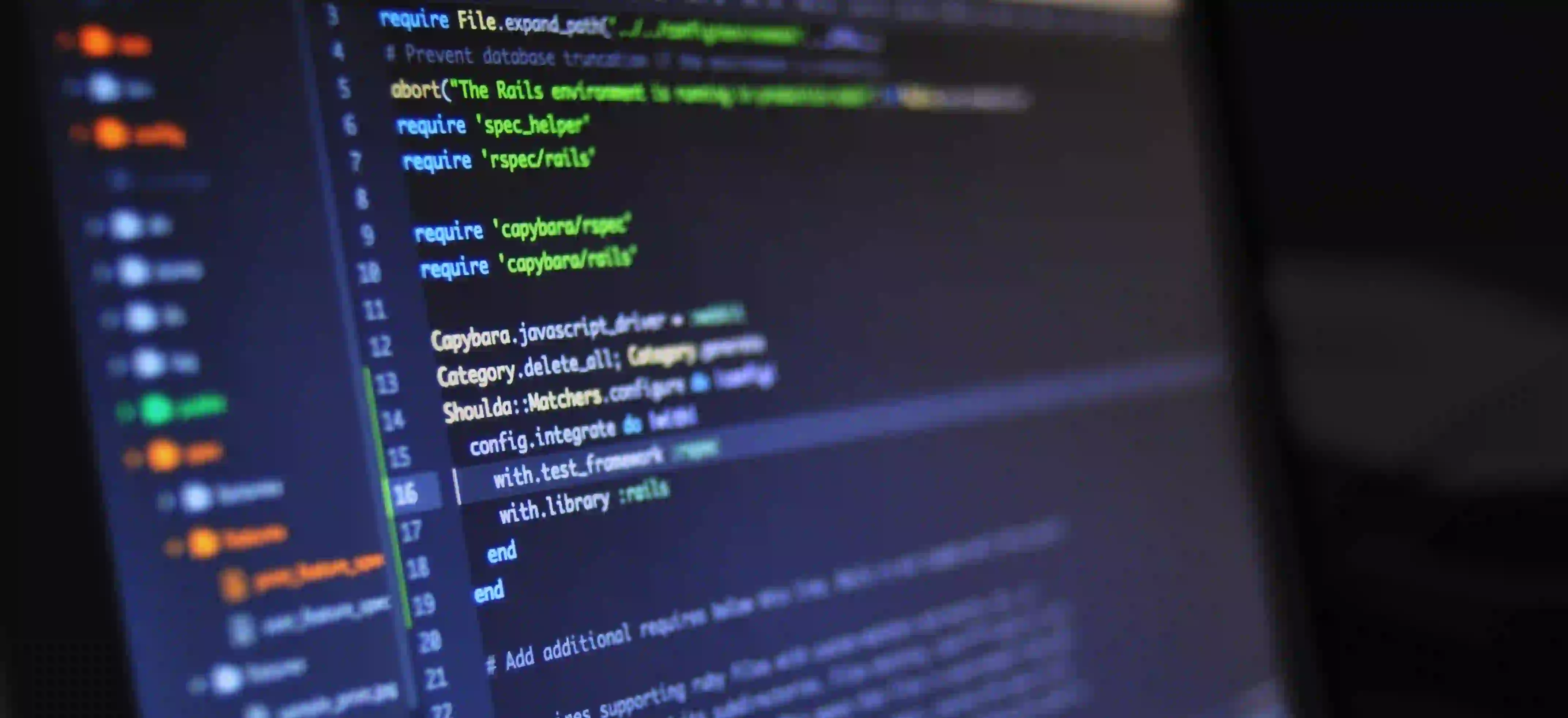
When it comes to securing your digital assets, two key practices come to mind: penetration testing (pen testing) and code review. Both are essential for identifying and mitigating potential security risks in your Java applications. In this blog post, we'll delve into the significance of each practice, their differences, and how they complement each other in ensuring the robustness of your Java codebase.
The Importance of Penetration Testing
Penetration testing, often referred to as pen testing, is a proactive approach to identifying vulnerabilities in a software system by simulating real-world cyber attacks. This process involves a systematic analysis of the system for any weaknesses, technical flaws, or vulnerabilities that malicious hackers could exploit.
Why Pen Testing Matters
- Real-world Simulation: Pen testing replicates the tactics and techniques used by attackers in a controlled environment, providing a realistic assessment of your system's security posture.
- Risk Identification: It helps in identifying potential security gaps that could lead to data breaches, service disruptions, or unauthorized access.
- Compliance Requirements: Many industry standards and regulations, such as PCI DSS and HIPAA, mandate regular pen testing to demonstrate the security of applications and infrastructure.
Sample Pen Testing in Java
public class SamplePenTest {
public boolean login(String username, String password) {
// Authentication logic
}
// Other sensitive methods and functionalities
}
In the Java code snippet above, a simple login
method is susceptible to common vulnerabilities like SQL injection, sensitive data exposure, or insecure authentication. Pen testing focuses on uncovering and addressing such vulnerabilities.
The Significance of Code Review
Code review, on the other hand, involves a thorough examination of the source code to find programming errors, security loopholes, or deviations from best practices. It aims to ensure the overall quality and security of the codebase, thus mitigating potential risks at an early stage of the development lifecycle.
Why Code Review Matters
- Bugs and Vulnerability Detection: It helps in identifying software defects, security vulnerabilities, and performance issues in the code.
- Knowledge Sharing: Code reviews facilitate knowledge sharing and ensure that best practices are followed across the development team.
- Early Issue Detection: By catching issues early in the development process, code review reduces the cost and effort of fixing problems in later stages.
Sample Code Review in Java
public class SampleCodeReview {
public void processData(String input) {
// Insecure direct object references
File file = new File(input);
// Input validation and sanitization missing
// ...
}
// Other potential security pitfalls
}
In the above Java code snippet, the processData
method lacks input validation and is susceptible to insecure direct object references. Through code review, these issues can be identified and rectified before deployment.
Complementary Roles of Pen Testing and Code Review
While pen testing and code review serve distinct purposes, they are complementary in nature and together form a robust security strategy for your Java applications.
- Identifying Different Layers of Vulnerabilities: Pen testing typically focuses on identifying vulnerabilities at the network and system levels, while code reviews deeply analyze the application code for logic flaws and implementation-level vulnerabilities.
- Full-Coverage Assurance: When both practices are employed, they provide comprehensive coverage, ensuring that the application is secure not only at the surface level but also at its core.
Example Integration
// Incorporating Security-Focused Code Reviews
public class SecureCodeExample {
// Code implementation
// Security-focused code review comments and suggestions
}
By integrating security-focused code reviews within the development lifecycle, potential vulnerabilities identified during the code review process can be verified through pen testing, further ensuring the application's resilience against security threats.
Leveraging Automated Tools
In both pen testing and code review, automated tools play a pivotal role in streamlining the process and identifying common vulnerabilities. For Java applications, utilizing tools like FindBugs, PMD, Checkmarx, and OWASP dependency-check can significantly enhance the effectiveness of both practices.
Sample Usage of Automated Tools
// Using FindBugs to identify potential code issues
@Nonnull
public String processInput(@CheckForNull String input) {
// Method implementation
}
The aforementioned code snippet demonstrates the integration of the @Nonnull
and @CheckForNull
annotations, which can be examined by automated tools to enforce null-safety and enhance code quality.
Lessons Learned: Balancing Security Measures
In conclusion, both pen testing and code review are indispensable pillars of a robust security strategy for your Java applications. While pen testing serves to identify vulnerability at the system level, code review delves deep into the code to mitigate implementation-level risks. Integrating both practices allows for a holistic approach to securing your digital assets, providing assurance that your Java applications are resilient against potential threats.
By leveraging automated tools in conjunction with manual assessments, developers and security professionals can effectively identify and address security gaps, making your Java applications more secure and resistant to cyber threats.
Remember, security should be a proactive effort - integrate both pen testing and code review into your development processes to construct a sturdy defense for your digital assets.