Mastering Java EE Async Operations: Challenges & Solutions
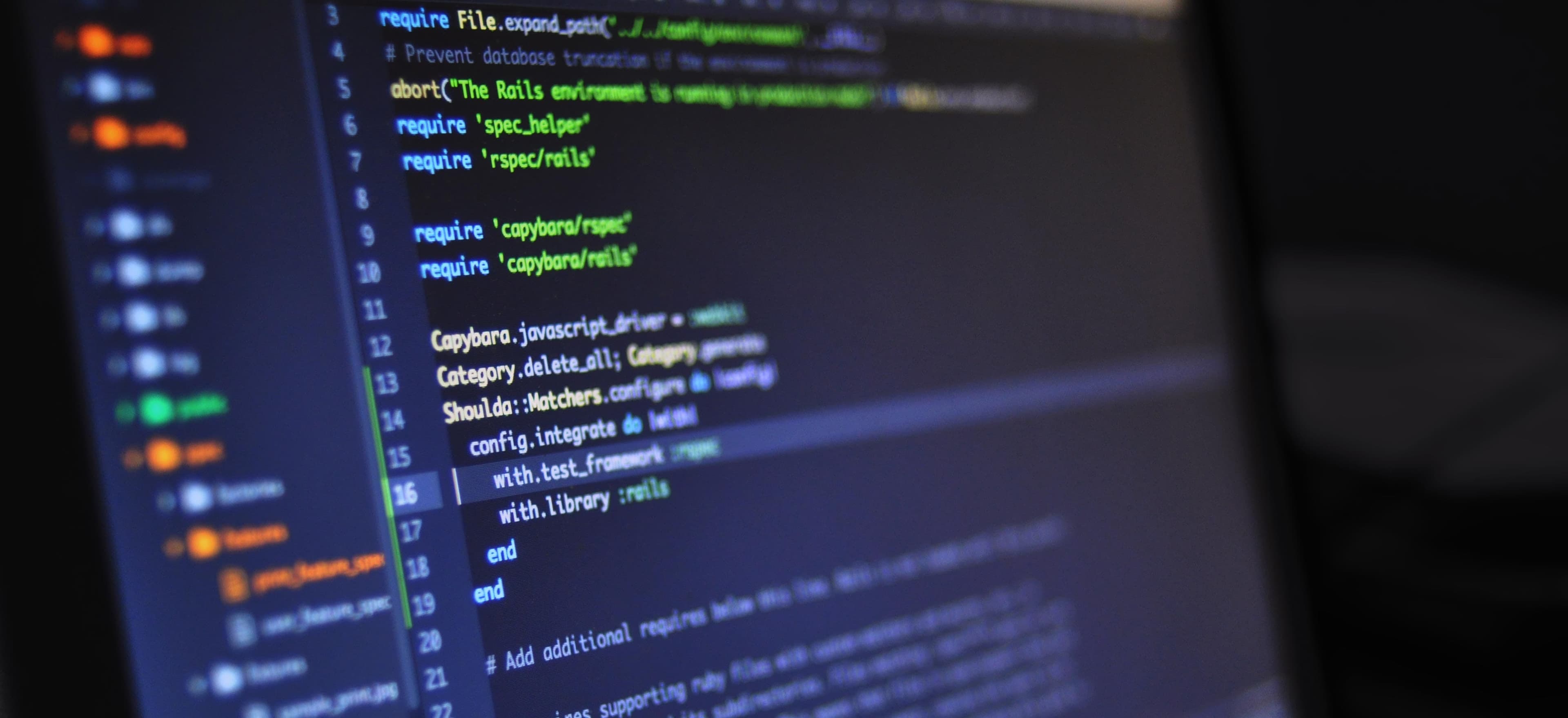
- Published on
Mastering Java EE Async Operations: Overcoming Challenges & Implementing Solutions
As a Java developer, you know the importance of writing efficient and responsive applications. In today's fast-paced digital world, users expect applications to provide quick responses and handle multiple tasks simultaneously. This is where asynchronous operations come into play. In this article, we will dive deep into mastering asynchronous operations in Java EE, exploring the challenges developers face and implementing effective solutions.
Understanding Asynchronous Operations in Java EE
Asynchronous operations allow an application to perform tasks in a non-blocking manner, freeing up resources and improving overall responsiveness. In the Java EE ecosystem, asynchronous processing can be achieved using various mechanisms such as CompletableFuture
, EJB (Enterprise JavaBeans) async methods, and the @Asynchronous
annotation.
Let's start by looking at a sample code snippet using CompletableFuture
to perform a time-consuming operation asynchronously:
import java.util.concurrent.CompletableFuture;
public class AsyncExample {
public static void main(String[] args) {
CompletableFuture.runAsync(() -> {
// Perform time-consuming operation
// ...
});
// Continue with other tasks
}
}
In this example, the runAsync
method allows the time-consuming operation to be executed asynchronously, while the main thread can continue with other tasks.
Challenges Faced in Asynchronous Operations
Despite the benefits of asynchronous processing, developers often encounter challenges when implementing and managing asynchronous operations in Java EE applications. Some of the common challenges include:
1. Error Handling
Handling errors and exceptions in asynchronous code can be complex, as they are not directly propagated to the calling code. Ensuring proper error handling and effective debugging becomes crucial in asynchronous operations.
2. Resource Management
Managing resources such as database connections, thread pools, and memory allocation in asynchronous operations requires careful consideration to avoid resource leaks and inefficiencies.
3. Debugging and Testing
Debugging and testing asynchronous code poses unique challenges, as traditional debugging techniques may not provide insights into the flow of asynchronous operations.
Solutions to Overcome Asynchronous Operation Challenges
1. Error Handling with CompletableFuture
CompletableFuture provides methods to handle errors and exceptions in asynchronous operations. By utilizing the handle
and exceptionally
methods, developers can effectively manage errors and propagate exceptions to the calling code.
CompletableFuture<Void> future = CompletableFuture.runAsync(() -> {
// Perform asynchronous operation
throw new RuntimeException("Error occurred");
});
future.exceptionally(ex -> {
System.err.println("Error occurred: " + ex.getMessage());
return null;
});
The exceptionally
method allows developers to handle exceptions and perform necessary error recovery or logging.
2. Resource Management with EJB Asynchronous Methods
Enterprise JavaBeans (EJB) provides a reliable mechanism for resource management in asynchronous operations. By utilizing EJB's container-managed features, developers can delegate resource management tasks, such as thread pooling and transaction handling, to the application server.
@Stateless
public class AsyncBean {
@Asynchronous
public void performAsyncOperation() {
// Perform asynchronous operation with managed resource handling
}
}
EJB's container-managed approach ensures efficient resource utilization and avoids common pitfalls associated with manual resource management.
3. Debugging and Testing Strategies
To address the challenges of debugging and testing asynchronous code, developers can leverage tools such as IntelliJ IDEA's async stack traces and CompletableFuture's join
method. These tools provide insights into the execution flow of asynchronous operations and aid in identifying potential issues.
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
// Perform asynchronous operation
return "Result";
});
String result = future.join();
System.out.println("Async operation result: " + result);
The join
method allows the calling code to synchronously wait for the result of the asynchronous operation, simplifying testing and debugging processes.
Final Thoughts
Mastering asynchronous operations in Java EE requires a thorough understanding of the challenges involved and the implementation of effective solutions. By leveraging mechanisms such as CompletableFuture and EJB, and adopting proper error handling and debugging strategies, developers can build highly responsive and efficient applications.
Incorporating asynchronous operations into Java EE applications not only enhances performance but also paves the way for scalable and resilient systems capable of meeting the demands of modern software development.
To delve deeper into best practices for asynchronous operations in Java EE, refer to the official Java EE documentation and explore real-world use cases and solutions.
Implementing the discussed strategies will not only improve the efficiency of your Java EE applications but also equip you with the essential skill set to tackle the complexities of asynchronous operations in the ever-evolving landscape of enterprise software development.
Checkout our other articles