Solving Type Boundaries: The Scala Pimp My Library Trick
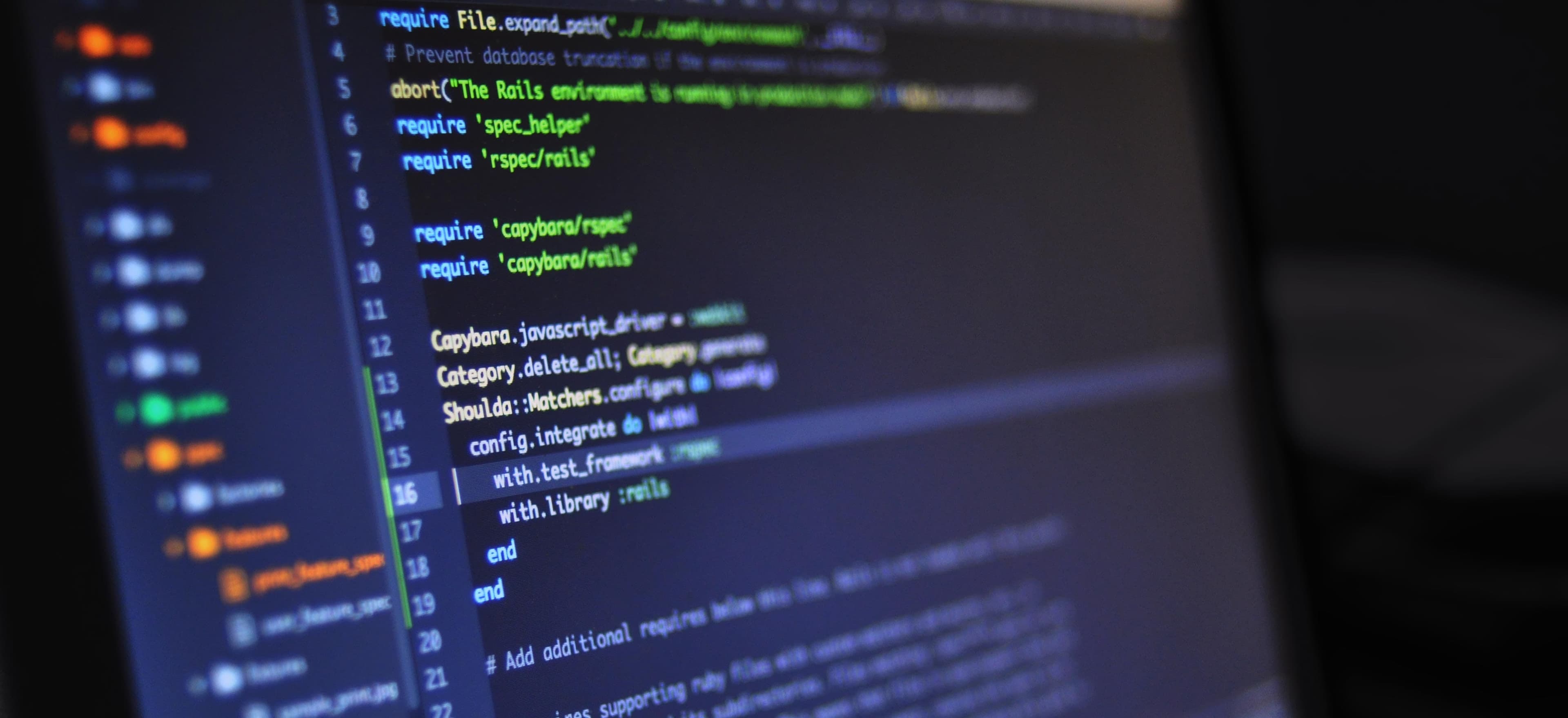
- Published on
Solving Type Boundaries: The Scala Pimp My Library Trick
Scala is a powerful, statically typed language that offers a rich set of features for building robust and scalable applications. One of the key concepts in Scala, as in any typed language, is the notion of type boundaries. It ensures that the types used in a program adhere to certain constraints, which is critical for maintaining code correctness, reliability, and performance. However, there are times when developers might encounter limitations imposed by type boundaries, especially when dealing with existing libraries or built-in types. This is where the "Pimp My Library" pattern comes into play.
In this article, we will explore the "Pimp My Library" pattern and its role in addressing type boundary issues. We'll begin with an overview of type boundaries in Scala, then delve into the "Pimp My Library" pattern, discuss its implementation, and finally explore real-world applications and best practices for using this pattern.
What You Will Learn
- The concept of type boundaries in Scala
- What the "Pimp My Library" pattern is
- How to implement the "Pimp My Library" pattern
- Real-world use cases where this pattern can be highly beneficial
The Basics of Type Boundaries in Scala
Understanding Type Boundaries
In Scala, type boundaries, or type constraints, are used to limit the types that can be used in certain contexts. There are two main forms of type boundaries: upper bounds and lower bounds. An upper bound restricts a type to be a subtype of a specified type, while a lower bound restricts a type to be a supertype of a specified type.
// Example of an upper type boundary
class Container[A <: Number] {
...
}
In the above example, the type parameter A
is constrained to be a subtype of Number
. This ensures that only numeric types can be used as the type parameter for Container
.
Why Type Boundaries Matter
Type boundaries are crucial for maintaining type safety and preventing unexpected type mismatches in a program. By enforcing these constraints, Scala allows developers to write code that is more reliable and easier to reason about. Additionally, type boundaries enable the compiler to perform more advanced type inference and optimization, leading to improved performance of the resulting code.
What is the "Pimp My Library" Pattern?
Introduction to the Pattern
The "Pimp My Library" pattern, often referred to as implicit class conversion, is a Scala programming technique that allows developers to extend existing libraries or built-in types without modifying their source code. The term "pimp" here is used in a tongue-in-cheek manner, referring to the act of enhancing or dressing up something to make it more attractive or powerful.
How It Works
At the core of the "Pimp My Library" pattern is the concept of implicit conversions in Scala. Implicit conversions allow the compiler to automatically convert one type to another when necessary. By leveraging implicit conversions and implicit classes, developers can add new methods or functionalities to existing types without modifying the original type or its companion object.
Implicit classes provide a convenient way to enrich existing types with new methods. When an implicit class is in scope, the compiler automatically applies the implicit conversion to wrap the original type with the implicit class, making the additional methods available.
Implementing the "Pimp My Library" Pattern
Step-by-Step Guide
To illustrate the implementation of the "Pimp My Library" pattern, let's consider extending the String
class with a new method called isEmptyOrWhiteSpace
. This method will check if the string is either empty or consists of whitespace characters only.
object StringUtil {
implicit class StringOps(val s: String) {
def isEmptyOrWhiteSpace: Boolean = {
s.trim.isEmpty
}
}
}
In the example above, an implicit class StringOps
is defined with a single method isEmptyOrWhiteSpace
, which leverages the existing trim
and isEmpty
methods of the String
class to achieve the desired functionality.
Through the use of implicit classes, the isEmptyOrWhiteSpace
method becomes seamlessly available for any String
instance in the scope where the implicit class is defined.
Additional Examples
In addition to extending the String
class, developers can utilize the "Pimp My Library" pattern in various scenarios. For instance, custom filters can be added to collections, new mathematical operations can be introduced for numeric types, or external APIs can be integrated more naturally into Scala applications.
Real-World Applications
Common Use Cases
The "Pimp My Library" pattern offers a range of practical applications in real-world scenarios. One common use case is in data processing, where custom extensions to existing types can facilitate complex data transformations and manipulations. Additionally, when working with legacy code, the pattern can be employed to augment and modernize outdated libraries or APIs.
Another scenario where the pattern shines is in simplifying the interaction with external services or APIs. By enriching the existing types with domain-specific methods, developers can streamline the integration process and improve the overall readability of the code.
Benefits and Drawbacks
The primary advantage of using the "Pimp My Library" pattern is the enhanced modularity it brings to the codebase. By separating the extension methods from the original type definitions, developers can manage and organize their code more effectively. Additionally, the pattern promotes reusability by allowing the same set of extensions to be applied across different projects or modules.
However, it is essential to be mindful of the potential drawbacks, particularly related to code comprehension and implicit conversions. Overuse of implicit conversions may lead to code that is harder to understand, especially for developers who are not intimately familiar with all the implicit conversions in use.
Best Practices and Tips
When implementing the "Pimp My Library" pattern, it's important to adhere to certain best practices to ensure that the code remains readable and maintainable.
-
Naming Conventions: Choose method and class names that clearly indicate their purpose and association with the original type. This helps in making the extended functionality more intuitive to use.
-
Implicit Classes Usage: Exercise caution when defining implicit classes to avoid unintended conversions. Generally, it's recommended to limit the scope of implicit classes to avoid unexpected behavior.
-
Avoid Excessive Overloading: While the "Pimp My Library" pattern allows for extensive customizations, it's advisable to avoid overloading existing types with an excessive number of additional methods. Doing so can clutter the interface and result in a less cohesive API.
Closing Remarks
In conclusion, the "Pimp My Library" pattern offers an elegant and powerful way to overcome type boundary limitations in Scala. By leveraging implicit conversions and implicit classes, developers can enhance existing types with new functionalities, thereby improving code extensibility and readability.
Understanding type boundaries and the "Pimp My Library" pattern is crucial for Scala developers looking to create flexible and expressive codebases. With careful application and consideration of best practices, this pattern can greatly enhance the modularity and reusability of Scala code.
As you delve further into Scala development, don't hesitate to experiment with the "Pimp My Library" pattern in your projects. By embracing this pattern, you'll be well-equipped to tackle the challenges of type boundaries and create more elegant and scalable solutions in your Scala applications.
Further Reading
- Official Scala Documentation on Implicit Conversions
- Blog Post: The "Pimp My Library" Pattern in Depth