Unlocking Reactive Apps: Overcoming Scala Challenges
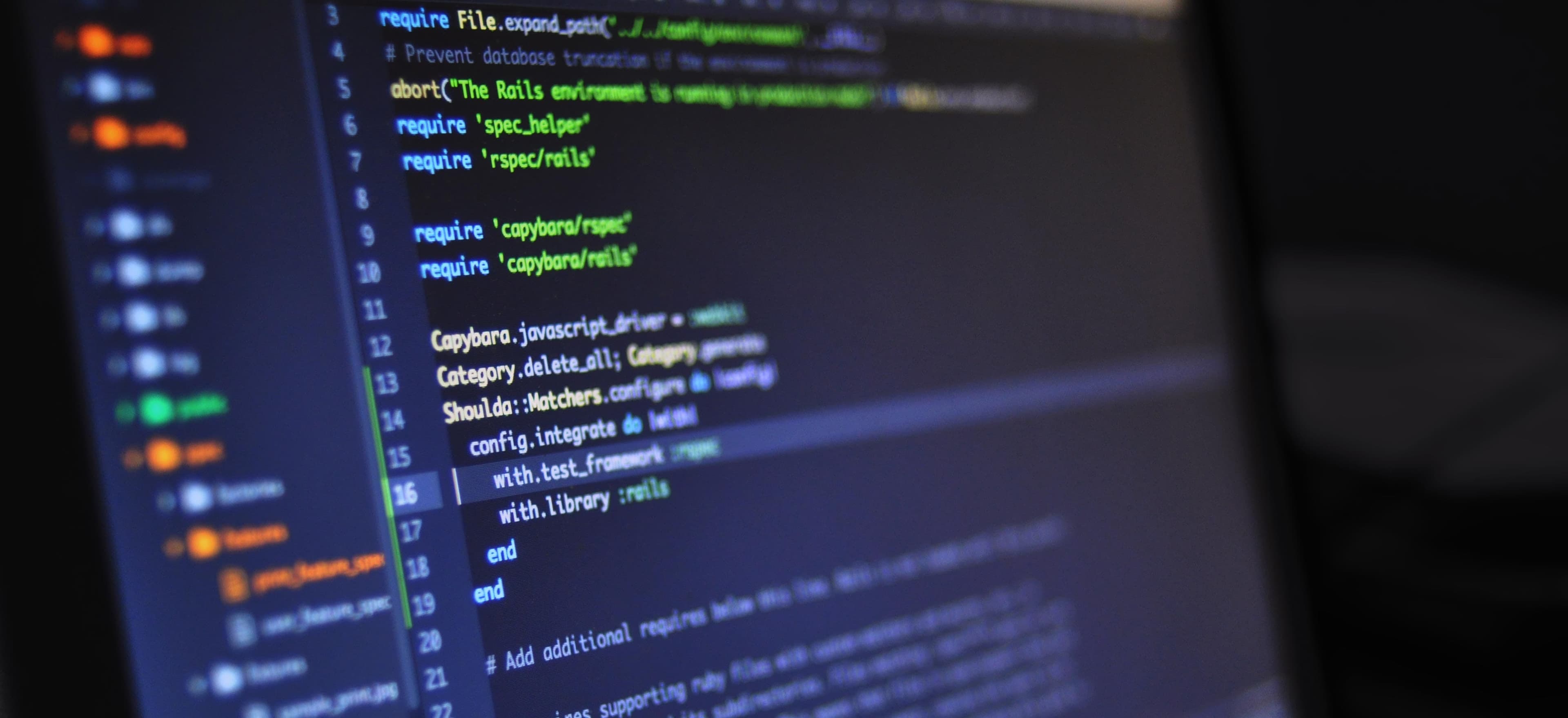
- Published on
Building Responsive and Scalable Applications with Scala
Scala has gained popularity in recent years due to its powerful features that allow developers to build highly scalable and responsive applications. However, building reactive applications in Scala comes with its own set of challenges. In this blog post, we will explore these challenges and discuss strategies to overcome them, empowering you to build robust and efficient reactive applications in Scala.
What are Reactive Applications?
Reactive applications are designed to be responsive, resilient, elastic, and message-driven. They are built to handle a large number of concurrent users and high throughput, providing a seamless and efficient user experience. Scala's support for functional programming, immutable data structures, and asynchronous programming make it an ideal choice for building reactive applications.
Challenges of Building Reactive Applications in Scala
While Scala offers a powerful set of tools for building reactive applications, developers often encounter challenges related to concurrency, asynchronous programming, and performance optimization. Let's delve into some of these challenges and explore how to address them effectively.
Concurrency Management
Concurrency management is a critical aspect of building reactive applications. Scala offers various concurrency primitives such as Future
, Akka actors
, and scalaz.concurrent.Task
to manage concurrent computations. However, coordinating and synchronizing concurrent tasks while maintaining responsiveness can be a daunting task.
One effective strategy to address this challenge is to utilize the Actor
model provided by Akka. Actors provide a high-level abstraction for managing concurrent tasks, allowing developers to focus on message passing and let the underlying framework handle the concurrency details. By leveraging Akka actors, developers can build scalable and responsive applications while managing concurrency effectively.
import akka.actor.{Actor, ActorSystem, Props}
class MyActor extends Actor {
def receive = {
case message: String => println(s"Received message: $message")
}
}
val system = ActorSystem("MyActorSystem")
val myActor = system.actorOf(Props[MyActor], "myActor")
myActor ! "Hello, Actor"
In the above code snippet, we define an Actor
using Akka and send a message to it. Akka's actor model simplifies concurrency management and enables developers to build reactive applications with ease.
Asynchronous Programming
Asynchronous programming is fundamental to building reactive applications that can handle a high degree of concurrency. Scala provides powerful abstractions such as Future
and Promise
for asynchronous programming. However, dealing with callback hell and composing complex asynchronous operations can make the code difficult to reason about and maintain.
To address this challenge, developers can leverage libraries like Monix
or ZIO
, which provide composable and expressive abstractions for asynchronous programming. These libraries offer constructs such as Task
and IO
that enable developers to compose asynchronous operations in a clear and concise manner, simplifying the complexity of asynchronous code.
import monix.eval.Task
val task: Task[String] = Task("Hello, Monix")
val result: Task[String] = task.map(_.toUpperCase)
result.runToFuture.foreach(println)
The above code snippet demonstrates the use of Task
from the Monix library to perform asynchronous operations and compose the results. By utilizing libraries like Monix, developers can write clean and maintainable asynchronous code, overcoming the challenges associated with asynchronous programming in Scala.
Performance Optimization
Building reactive applications often involves optimizing performance to ensure responsiveness and scalability. Scala's expressive and concise syntax, combined with its support for functional programming, provides a solid foundation for writing high-performance code. However, optimizing the performance of concurrent and asynchronous operations can be challenging, especially when dealing with large-scale applications.
To address performance optimization challenges, developers can employ techniques such as non-blocking I/O, caching, and load balancing. Scala's ecosystem offers libraries like Akka HTTP
for building high-performance, asynchronous, and scalable web services. By utilizing these libraries and employing performance optimization techniques, developers can ensure that their reactive applications perform efficiently under high loads.
Wrapping Up
Building reactive applications in Scala presents various challenges related to concurrency management, asynchronous programming, and performance optimization. By leveraging the powerful abstractions provided by libraries like Akka, Monix, and leveraging performance optimization techniques, developers can overcome these challenges and build robust and efficient reactive applications in Scala.
With the increasing demand for responsive and scalable applications, mastering the art of building reactive applications in Scala is becoming essential for modern developers. By understanding and addressing the challenges discussed in this blog post, developers can unlock the full potential of Scala for building highly responsive and scalable applications, pushing the boundaries of what is achievable in the world of reactive programming.
Reactive programming in Scala offers a world of possibilities, and overcoming its challenges opens the door to creating innovative and highly performant applications that meet the demands of today's digital landscape.
Start building reactive applications in Scala and unleash the power of concurrency, asynchronous programming, and performance optimization to create responsive and scalable applications that truly shine in the modern digital era.
So, push the boundaries, embrace the challenges, and unlock the true potential of reactive applications in Scala.
Now, it's your turn to dive into the world of reactive programming with Scala and conquer the challenges that come your way, ultimately emerging as a master of building responsive and scalable applications.
Get ready to revolutionize the way you build applications and embark on an exciting journey into the realm of reactive programming with Scala.
Are you ready to embrace the power of reactive programming in Scala? The possibilities are endless, and the future is in your hands. Let's embark on this journey together and pave the way for a new era of responsive and scalable applications with Scala.
Embrace the challenges, unleash the power, and build the future of reactive applications with Scala!