Mastering Java Interview Questions: A Specifically Tough One!
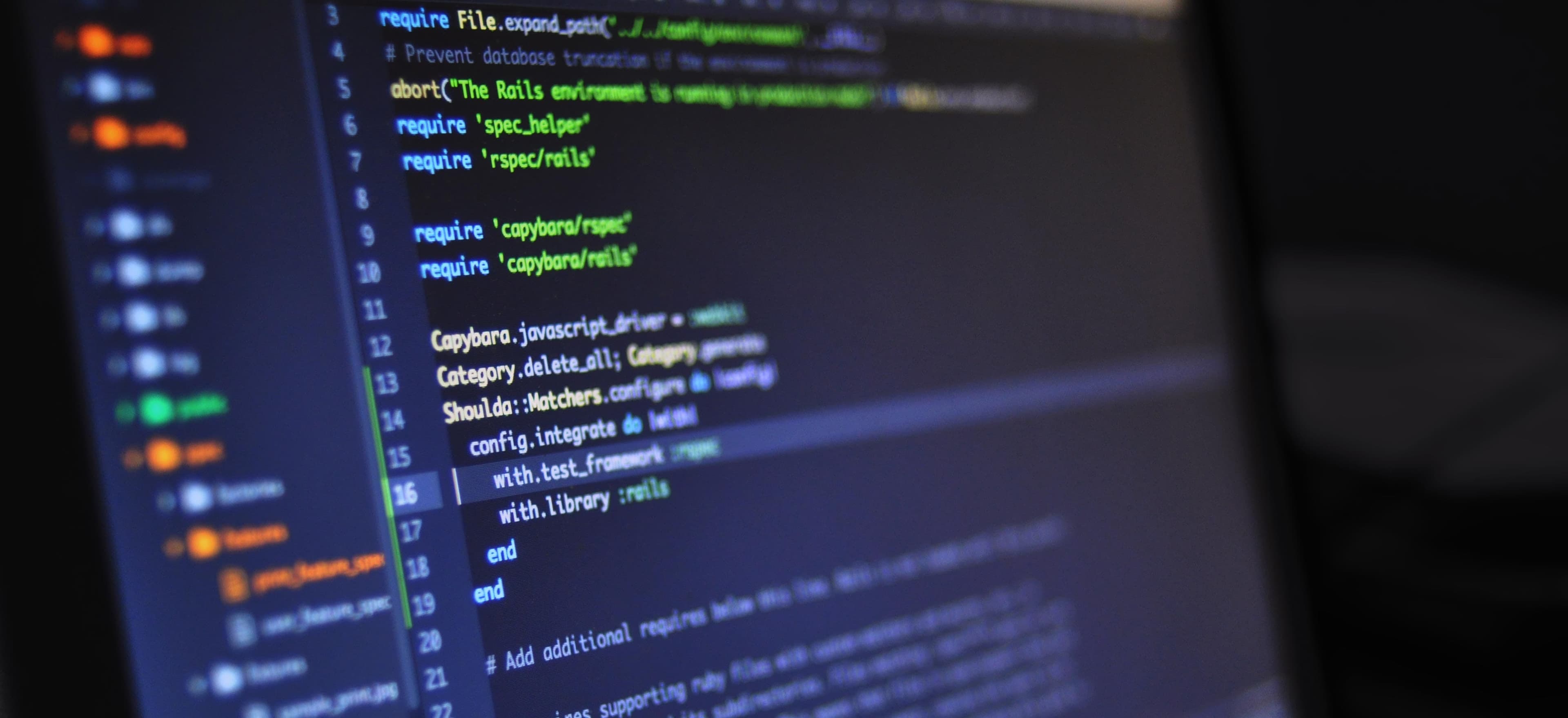
- Published on
Mastering Java Interview Questions: A Specifically Tough One!
As a Java developer, preparing for interviews requires a deep understanding of the language, its features, and its best practices. In this article, we'll explore a particularly tough Java interview question and break down the thought process behind solving it. We'll cover concepts such as multithreading, synchronization, and volatile keyword to tackle the challenge head-on.
The Tough Interview Question: Volatile Keyword Usage
The question that often catches Java developers off guard is around the volatile
keyword. Understanding its purpose and correct usage is crucial for writing thread-safe code in Java. Let's delve into the question:
Interviewer's Question: Can you explain the purpose of the volatile
keyword in Java and provide a scenario where using volatile
is appropriate?
Understanding the Volatile Keyword
The volatile
keyword in Java is used to indicate that a variable's value may be changed by multiple threads. It ensures that any read or write operation on the variable is directly performed on the main memory, rather than in the thread's local cache. This prevents visibility and ordering issues that can occur in multithreaded environments.
When a variable is declared with the volatile
keyword, it guarantees that any thread accessing the variable sees the most recent write. This is particularly important in scenarios where multiple threads are involved and the variable's value may be updated concurrently.
Example Code with Volatile Keyword
Let's consider a scenario where the volatile
keyword is crucial. Below is a simplified example of a shared variable accessed by multiple threads without using volatile
:
public class SharedResource {
private boolean flag = false;
public void setFlag() {
flag = true;
}
public boolean isFlagSet() {
return flag;
}
}
In a multithreaded environment, if one thread calls setFlag()
and another thread calls isFlagSet()
, there's no guarantee that the change made by setFlag()
will be immediately visible to the other thread due to caching. This can lead to unexpected behavior.
Now, let's modify the flag
variable to use the volatile
keyword:
public class SharedResource {
private volatile boolean flag = false;
// other methods remain the same
}
By marking the flag
variable as volatile
, we ensure that any update to flag
is immediately visible to all threads. This eliminates the visibility issue and ensures consistent behavior across all threads.
Explaining the Scenario
In a scenario where multiple threads are updating and accessing a shared variable, using the volatile
keyword is appropriate when you need to enforce visibility of changes to the variable across all threads. This is essential for maintaining data consistency and synchronization in multithreaded environments.
Wrap Up
Mastering tough Java interview questions requires a deep understanding of language features and best practices. The volatile
keyword is a crucial aspect of writing thread-safe code in Java, and its correct usage is fundamental to understanding multithreading concepts.
Understanding the purpose of volatile
, its implications on visibility and ordering, and knowing when to use it in multithreaded scenarios can set you apart in Java interviews. Practice writing code that leverages the volatile
keyword and grasp its significance in maintaining thread safety.
Remember, interview questions like these are not only about providing the correct answer but also about demonstrating your understanding and thought process. With a solid grasp of the volatile
keyword and its practical implications, you'll be better equipped to tackle tough Java interview questions with confidence.