Solving Thymeleaf & AngularJS Router View Clash
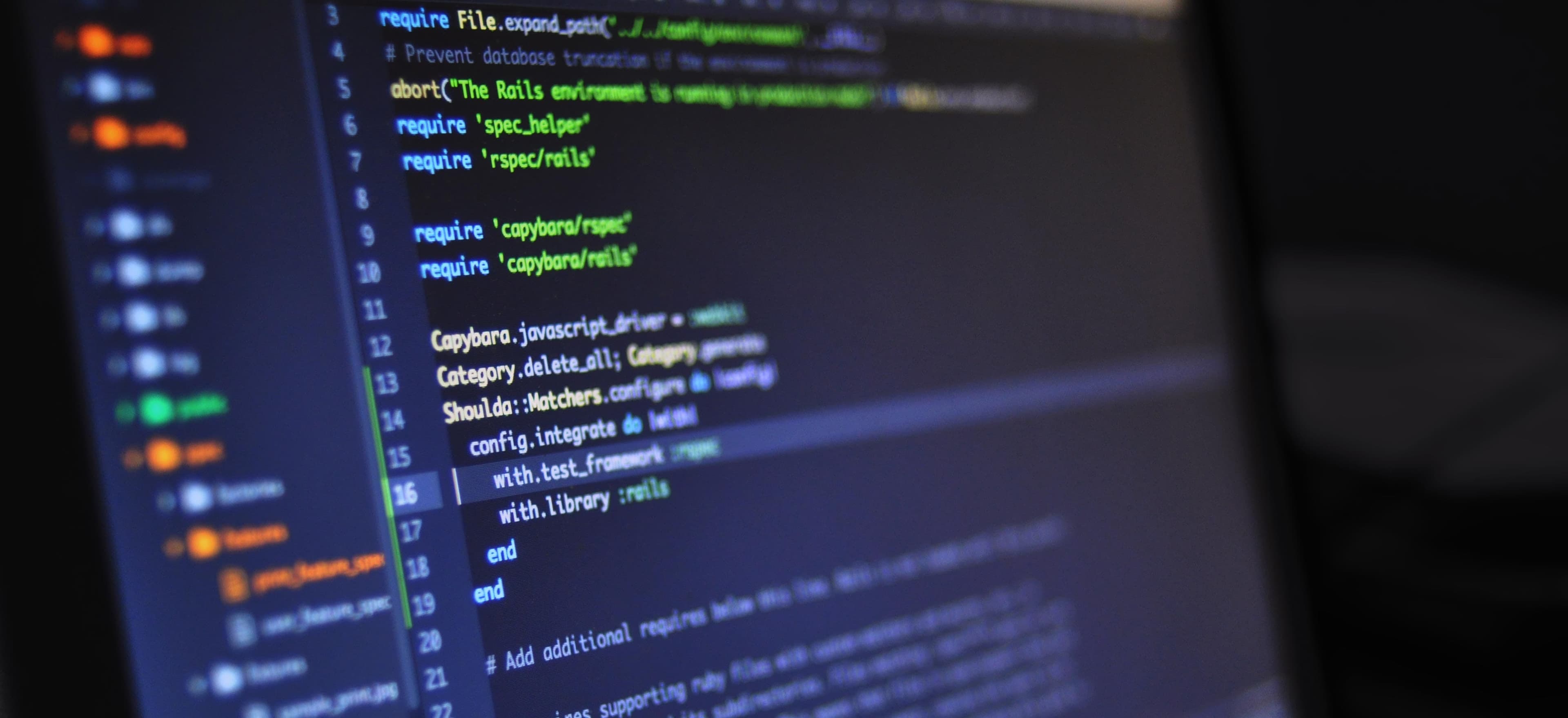
- Published on
Solving the Clash: Integrating Thymeleaf with AngularJS Router View
When combining the power of server-side Java frameworks with client-side JavaScript frameworks, developers often opt for a blend of Spring Boot with Thymeleaf and AngularJS. However, this amalgamation can present a unique challenge: the clash between Thymeleaf templates and AngularJS Routing. This blog post delves into the root of this problem and presents a streamlined solution to seamlessly integrate Thymeleaf and AngularJS Router View, ensuring a robust and dynamic web application development process.
Understanding the Clash
Before diving into the solution, it's critical to understand why Thymeleaf and AngularJS router view clash in the first place. Thymeleaf is a server-side Java template engine widely used for rendering HTML views. AngularJS, on the other hand, is a client-side framework known for its powerful routing capabilities that allow developers to build Single Page Applications (SPAs).
The clash arises primarily because both Thymeleaf and AngularJS use similar syntax for their expressions: ${...}
for Thymeleaf and {{...}}
for AngularJS. When Angular tries to route and render templates, Thymeleaf, processing the server-side HTML, may inadvertently parse AngularJS expressions, leading to errors or undesired outcomes.
The Solution Path
To resolve this conflict, developers can employ a few strategies to ensure that Thymeleaf processes the server-side templates without interfering with AngularJS routing and expressions.
Step 1: Configure AngularJS to Use a Different Interpolation Symbol
The first and simpler approach is to configure AngularJS to use a different set of brackets for its expressions, thus avoiding clashes with Thymeleaf's ${...}
syntax.
var myApp = angular.module('myApp', [], function($interpolateProvider) {
$interpolateProvider.startSymbol('[[');
$interpolateProvider.endSymbol(']]');
});
This snippet changes AngularJS's interpolation symbols from {{...}}
to [[...]]
. With this change, Angular expressions can coexist within Thymeleaf templates without being prematurely parsed by Thymeleaf.
Step 2: Leveraging Thymeleaf’s th:inline="none"
Another effective strategy involves utilizing Thymeleaf's capability to selectively disable its parsing within specific sections of an HTML page. This can be achieved by applying th:inline="none"
within your script tags.
<script th:inline="none">
var app = angular.module('myApp', ['ngRoute']);
app.config(function($routeProvider) {
$routeProvider
.when('/', {
templateUrl : 'pages/home.html',
controller : 'mainController'
})
// more routes here
});
</script>
By specifying th:inline="none"
, you tell Thymeleaf not to parse the content within the <script>
tag, thus preventing it from processing AngularJS expressions or router configurations.
Step 3: Integration Harmony
After implementing the above adjustments, the next step is to correctly structure your Thymeleaf templates and AngularJS routes to ensure smooth integration. Ideally, Thymeleaf should handle the initial page load, serving as the entry point. Subsequent internal navigation and partial page updates should then be managed by AngularJS through its routing mechanism.
An example of the AngularJS route configuration:
app.config(['$routeProvider', function($routeProvider) {
$routeProvider
.when('/home', {
templateUrl: 'views/home.html',
controller: 'HomeController'
})
.otherwise({
redirectTo: '/home'
});
}]);
For in-depth AngularJS documentation, including routing and SPA best practices, visit AngularJS's official guide.
In contrast, a simple Thymeleaf template might look something like this:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Integration Example</title>
</head>
<body>
<div ng-app="myApp">
<div ng-view></div>
</div>
<script src="angular.js"></script>
<script src="angular-route.js"></script>
<!-- Your AngularJS app scripts here -->
</body>
</html>
This template serves as the main layout, where Thymeleaf's duty primarily lies in loading the initial HTML structure and including necessary scripts and stylesheets. AngularJS then takes over for SPA functionality through its routing and view injection.
A Final Look
Integrating Thymeleaf with AngularJS router view can initially seem daunting due to their syntactic similarities and processing conflicts. However, by leveraging AngularJS's ability to customize interpolation symbols and utilizing Thymeleaf's th:inline="none"
directive, developers can create a harmonious integration that leverages the strengths of both technologies.
This solution not only solves the immediate conflict but also paves the way for more complex integrations between Java-based server-side frameworks and JavaScript-based client-side frameworks. As web development continues to evolve, understanding how to navigate these integrations effectively will be invaluable.
For more insights into Thymeleaf and its capabilities, be sure to explore the Thymeleaf official documentation. Here, you’ll find a wealth of information on Thymeleaf's syntax, features, and best practices for developing dynamic web applications.