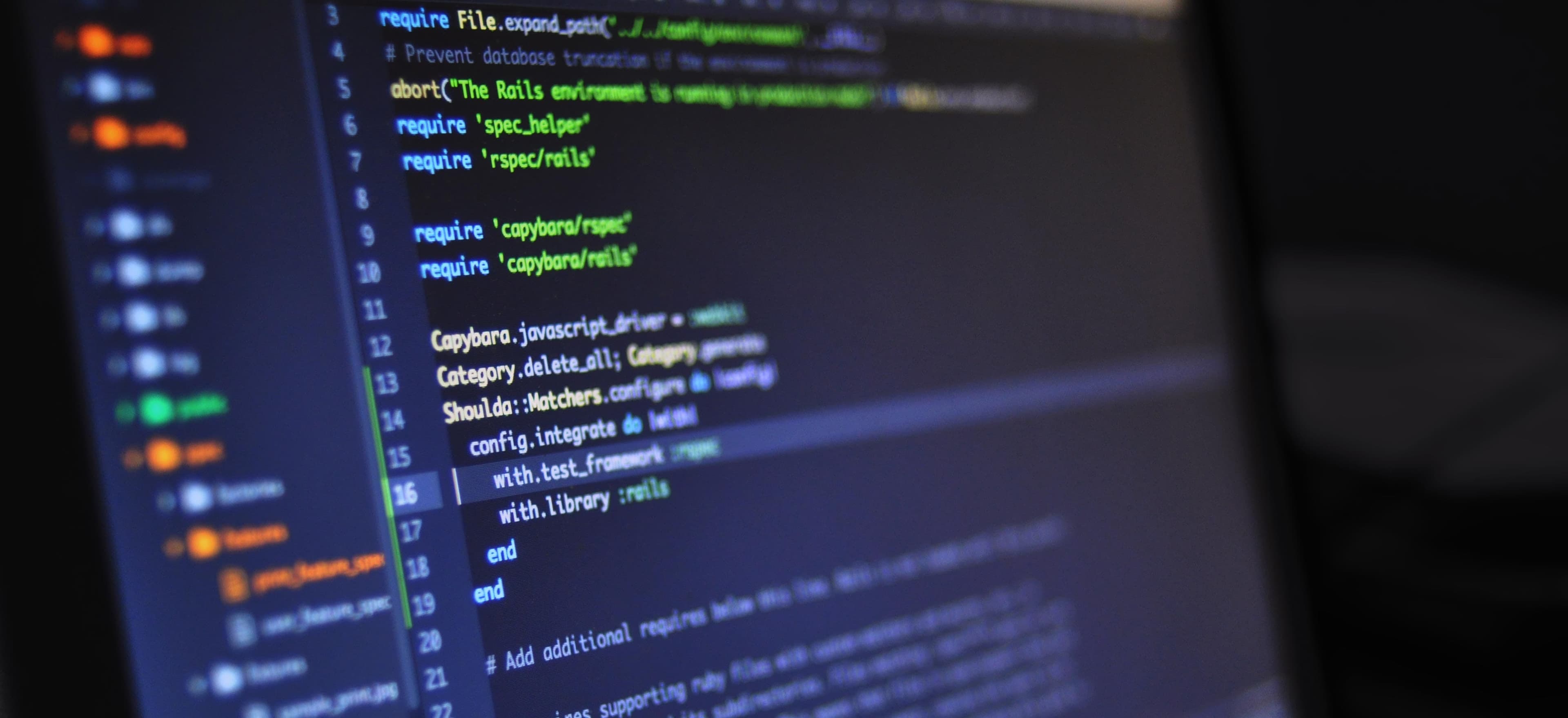
- Published on
Java EE 6 Security: Recipes for Robust Enterprise Apps
In the fast-paced world of enterprise applications, security is paramount. With Java EE 6, developers have a robust set of tools at their disposal to ensure that their applications are shielded from potential threats. This blog post will explore some essential security recipes for creating rock-solid enterprise apps using Java EE 6.
The Importance of Java EE 6 Security
Security is not an afterthought—it's a fundamental component of any enterprise application. Java EE 6 offers a comprehensive suite of security features, empowering developers to implement robust security mechanisms to protect their applications. By leveraging the built-in security capabilities of Java EE 6, developers can fortify their applications and safeguard sensitive data from unauthorized access and malicious attacks.
Recipe 1: Implementing Authentication and Authorization
Authentication and authorization are the building blocks of application security. Java EE 6 provides a powerful authentication and authorization framework that enables developers to control access to resources within their applications. Let's take a look at a code snippet that demonstrates how to configure authentication and authorization in a Java EE 6 application:
@DeclareRoles({"ADMIN", "USER"})
@RolesAllowed({"ADMIN", "USER"})
public class SecuredServlet extends HttpServlet {
// Servlet implementation
}
In this code snippet, the @DeclareRoles
annotation declares the roles that are allowed to access the servlet, while the @RolesAllowed
annotation specifies which roles are permitted to invoke the servlet's methods. This declarative approach simplifies the process of enforcing access control within the application.
Recipe 2: Securing Communication with SSL/TLS
In the era of ubiquitous internet connectivity, secure communication is imperative. Java EE 6 facilitates the implementation of secure communication using SSL/TLS protocols. By enabling SSL/TLS for web applications, developers can encrypt data transmitted between clients and servers, thwarting eavesdropping and tampering attempts. Let's delve into a code snippet that illustrates how to configure SSL/TLS for a Java EE 6 application:
<security-constraint>
<web-resource-collection>
<web-resource-name>Secure Pages</web-resource-name>
<url-pattern>/secure/*</url-pattern>
</web-resource-collection>
<user-data-constraint>
<transport-guarantee>CONFIDENTIAL</transport-guarantee>
</user-data-constraint>
</security-constraint>
In this snippet, the security constraint element specifies that any requests matching the /secure/*
URL pattern must be transmitted over a confidential (encrypted) channel, thereby enforcing secure communication using SSL/TLS.
Recipe 3: Preventing Cross-Site Scripting (XSS) Attacks
Cross-Site Scripting (XSS) attacks pose a significant threat to web applications by injecting malicious scripts into web pages viewed by other users. Java EE 6 equips developers with tools to mitigate XSS vulnerabilities. Let's examine a code snippet demonstrating how to prevent XSS attacks in a Java EE 6 application:
String userInput = request.getParameter("input");
String safeOutput = ESAPI.encoder().encodeForHTML(userInput);
In this code snippet, the ESAPI library is used to encode user input, ensuring that any potentially malicious HTML tags or scripts are neutralized. By applying input validation and output encoding techniques, developers can safeguard their applications against XSS exploits.
A Final Look
Java EE 6 offers a comprehensive suite of security features that empower developers to fortify their enterprise applications against a myriad of security threats. By implementing robust authentication and authorization mechanisms, securing communication with SSL/TLS, and mitigating XSS vulnerabilities, developers can ensure that their Java EE 6 applications are resilient against malicious attacks. As the foundation of secure enterprise applications, Java EE 6 security recipes play a vital role in safeguarding sensitive data and maintaining the integrity of mission-critical systems.
Additional Resources
To dive deeper into Java EE 6 security, consider exploring the official Java EE documentation and Oracle's Secure Coding Guidelines for Java SE for comprehensive insights into best practices and advanced security techniques.
With Java EE 6 security recipes in your arsenal, you can confidently develop robust and secure enterprise applications that withstand the ever-evolving landscape of cyber threats.