MVC 1.0 & Java EE 8: Master Facelets with Ease!
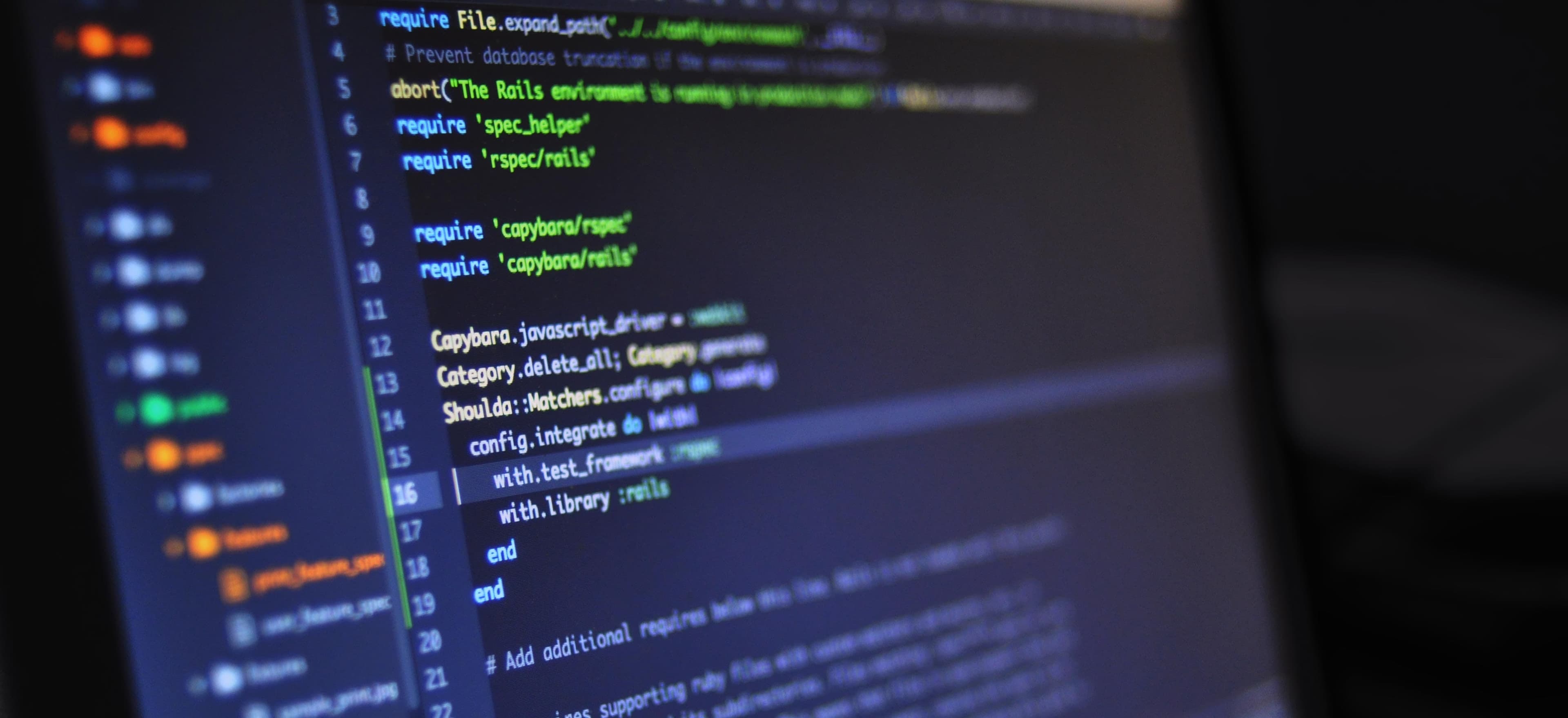
- Published on
Mastering Facelets in MVC 1.0 with Java EE 8
In the world of web development, creating dynamic and responsive web applications is a top priority. Java EE 8 provides an ideal platform for building such applications, and MVC 1.0 with Facelets is a powerful combination for achieving this goal. In this article, we will dive into the concept of Facelets and how it can be mastered with ease in MVC 1.0.
What are Facelets?
Facelets is a view technology for building JavaServer Faces (JSF) applications. It allows for the creation of template-based, composite, and reusable UI components. Facelets enhances the development experience by providing a simple yet robust way to create dynamic web pages.
Setting up a Java EE 8 Project
Let's start by setting up a new Java EE 8 project. You can use any IDE such as IntelliJ IDEA or Eclipse to create a new Maven or Gradle project with Java EE 8 support.
Once the project is set up, make sure to include the necessary dependencies for MVC 1.0 and Facelets in your pom.xml
or build.gradle
file.
pom.xml
<dependency>
<groupId>javax.mvc</groupId>
<artifactId>javax.mvc-api</artifactId>
<version>1.0-pr</version>
</dependency>
<dependency>
<groupId>org.glassfish</groupId>
<artifactId>javax.faces</artifactId>
<version>2.3.13</version>
</dependency>
build.gradle
implementation 'javax.mvc:javax.mvc-api:1.0-pr'
implementation 'org.glassfish:javax.faces:2.3.13'
By adding these dependencies, we ensure that our project is ready to leverage MVC 1.0 and Facelets for building modern web applications.
Creating a Facelets Template
One of the key features of Facelets is the ability to create reusable templates. Let's create a simple template called main.xhtml
which will serve as the base layout for our web pages.
main.xhtml
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html"
xmlns:f="http://xmlns.jcp.org/jsf/core">
<h:head>
<title>My Java EE 8 App</title>
</h:head>
<h:body>
<h1>Welcome to my Java EE 8 App</h1>
<div id="content">
<ui:insert name="content">Default content goes here</ui:insert>
</div>
</h:body>
</html>
In this template, we use Facelets tags such as <ui:insert>
to define a placeholder for the content of individual web pages. This template can then be reused across multiple pages, providing a consistent look and feel.
Using the Facelets Template in MVC 1.0
Now that we have our template in place, let's create a simple MVC 1.0 controller and a view that utilizes the Facelets template.
Creating a Controller
import javax.mvc.Controller;
import javax.mvc.Models;
import javax.mvc.View;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.inject.Inject;
@Controller
@Path("hello")
public class HelloController {
@Inject
private Models models;
@GET
@View("hello.jsp")
public void sayHello() {
models.put("message", "Hello, World!");
}
}
In this controller, we use annotations provided by MVC 1.0 to define a controller endpoint and the associated view. We inject the Models
object to pass data to the view.
Creating a View with Facelets
Let's create a view file called hello.jsp
which uses our previously defined Facelets template.
hello.jsp
<ui:composition template="/WEB-INF/views/main.xhtml"
xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html"
xmlns:f="http://xmlns.jcp.org/jsf/core"
xmlns:ui="http://xmlns.jcp.org/jsf/facelets">
<ui:define name="content">
<h2>${message}</h2>
</ui:define>
</ui:composition>
In this view file, we use Facelets tags such as <ui:composition>
and <ui:define>
to incorporate our main.xhtml
template and define the specific content to be inserted into the placeholder. We also use Expression Language (EL) to display the message passed from the controller.
Running the Application
With everything in place, you can now deploy your Java EE 8 application to a server such as Payara or WildFly and access the hello
endpoint in your browser. You will see the message "Hello, World!" rendered using the Facelets template.
Key Takeaways
By mastering Facelets with MVC 1.0 in Java EE 8, you can create elegant and maintainable web applications with ease. The combination of MVC 1.0 and Facelets provides a solid foundation for building modern, component-based web applications. As you delve deeper into Facelets, you'll discover its extensive capabilities for creating rich and interactive user interfaces within the Java EE ecosystem.
Start exploring the world of Facelets and MVC 1.0 in Java EE 8, and unleash the power of dynamic web application development!