Monolithic vs. Distributed Kafka: Optimal Architecture
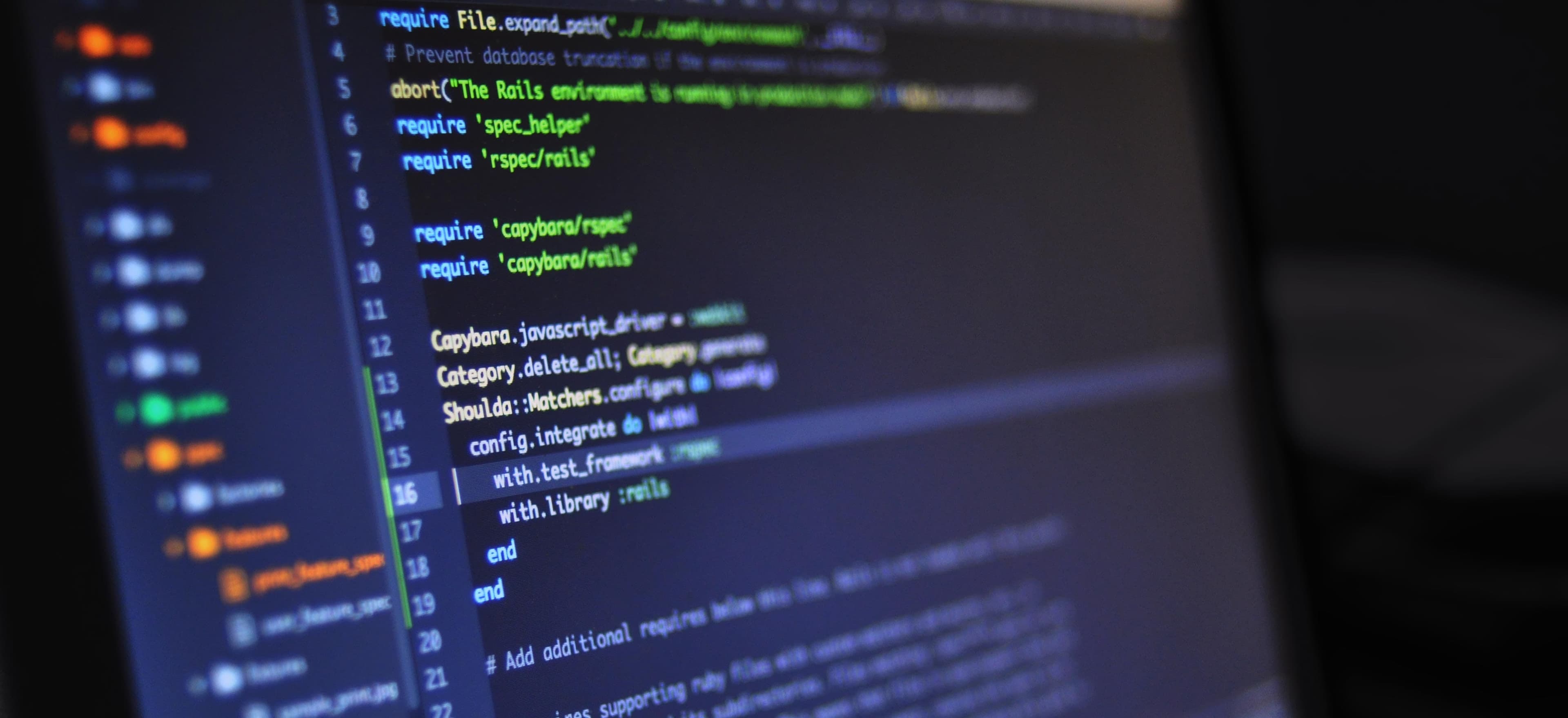
- Published on
Monolithic vs. Distributed Kafka: Optimal Architecture
When it comes to designing a system that handles large volumes of data, Apache Kafka is one of the most popular choices. Kafka is known for its high throughput, fault tolerance, and scalability. However, when architecting a Kafka system, one of the crucial decisions revolves around choosing between a monolithic and distributed architecture.
Monolithic Kafka Architecture
In a monolithic Kafka architecture, all components such as producers, consumers, brokers, and ZooKeeper coexist on a single node. This setup is suitable for small-scale applications with low throughput requirements.
When to Use Monolithic Kafka Architecture
-
Simplicity: It's ideal for simple use cases and when you want to get started quickly with Kafka without the overhead of a distributed setup.
-
Development and Testing: For local development and testing, a monolithic architecture provides an uncomplicated environment.
Code Example - Setting Up a Monolithic Kafka Instance
Properties props = new Properties();
props.put("bootstrap.servers", "localhost:9092");
props.put("acks", "all");
props.put("retries", 0);
props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
Producer<String, String> producer = new KafkaProducer<>(props);
In a monolithic setup, the bootstrap.servers
property points to the local Kafka broker, and all operations are handled within this single instance.
Distributed Kafka Architecture
In a distributed Kafka architecture, the system is designed to handle massive data volumes and high throughput by distributing the load across multiple nodes. This architecture includes multiple Kafka brokers, ZooKeeper for coordination, and may employ the use of Kafka Connect and Kafka Streams for stream processing.
When to Use Distributed Kafka Architecture
-
Scalability: When the volume of data or the rate of data ingestion increases, a distributed setup allows for seamless scalability.
-
Fault Tolerance: Distributed architecture provides fault tolerance, ensuring the system remains operational even if some nodes fail.
-
High Throughput: For use cases where high throughput is a requirement, distributed Kafka is the optimal choice.
Code Example - Configuring a Distributed Kafka Cluster
Properties props = new Properties();
props.put("bootstrap.servers", "broker1:9092,broker2:9092,broker3:9092");
props.put("acks", "all");
props.put("retries", 0);
props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
Producer<String, String> producer = new KafkaProducer<>(props);
In a distributed setup, the bootstrap.servers
property references multiple Kafka brokers, enabling load distribution and fault tolerance.
Making the Choice
Both monolithic and distributed Kafka architectures have their place, and the choice boils down to the specific requirements of your application.
-
Use Monolithic Kafka When: Your use case is small-scale, and you prioritize simplicity and ease of setup.
-
Use Distributed Kafka When: You anticipate or currently demand high scalability, fault tolerance, and throughput.
In conclusion, the optimal architecture for your Kafka system depends on your performance, scalability, and fault tolerance requirements. Understanding the nuances of both monolithic and distributed architectures will empower you to make an informed decision for your specific use case.
Incorporating a suitable Kafka architecture for your system is crucial for its success. Understanding the differences between monolithic and distributed architectures in Apache Kafka plays a pivotal role in powering applications to perform optimally. Whether you opt for a monolithic or distributed setup, ensuring that the chosen architecture aligns with your application’s requirements is the key to leveraging the full potential of Apache Kafka.