Top Strategies for Comparing Manifest Files in Groovy
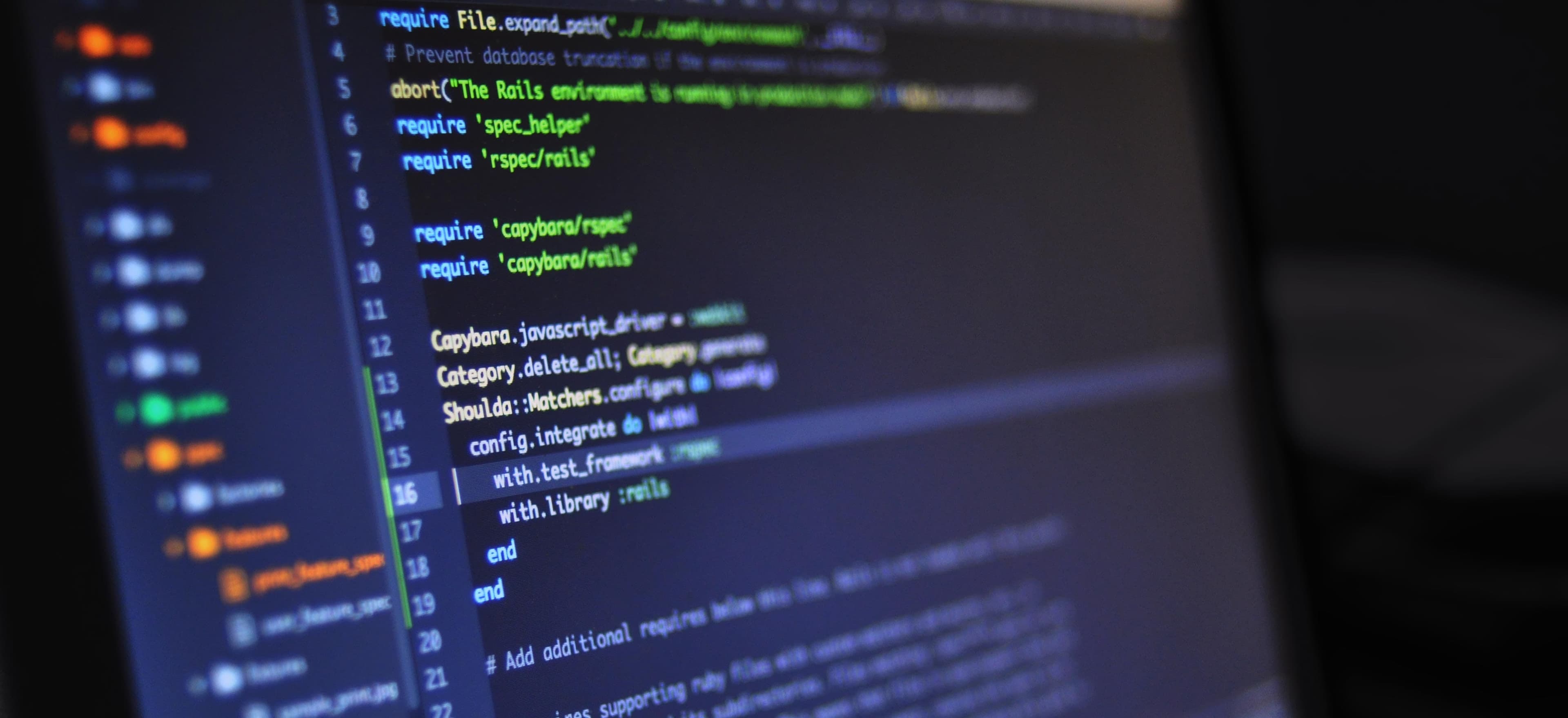
- Published on
Top Strategies for Comparing Manifest Files in Groovy
When working with Java applications, it's essential to ensure that the manifest files are correctly configured. Groovy, being a powerful and dynamic language for the Java Virtual Machine (JVM), offers several strategies for comparing manifest files. In this blog post, we'll explore some top strategies for achieving this, along with code examples to demonstrate each approach.
Strategy 1: Using Groovy's built-in File Comparison
One of the simplest ways to compare two manifest files is by utilizing Groovy's built-in file comparison capabilities. This method involves reading the contents of each manifest file and performing a line-by-line textual comparison.
Let's take a look at a sample Groovy code snippet for this approach:
def file1 = new File("path/to/manifest1.mf")
def file2 = new File("path/to/manifest2.mf")
def contents1 = file1.text
def contents2 = file2.text
if (contents1 == contents2) {
println "Manifest files are identical"
} else {
// Additional logic for handling the differences
println "Manifest files are different"
}
In this example, we read the contents of both manifest files using Groovy's File
class and compare their textual contents. This approach, however, only provides a basic textual diff and does not delve into the specifics of the manifest file structure.
Strategy 2: Using Apache Commons IO for Detailed Comparison
For a more detailed comparison, we can leverage the Apache Commons IO library to compare the manifest file attributes. This approach allows us to compare manifest file attributes such as Manifest-Version
, Main-Class
, and any custom attributes defined in the manifest.
Here's an example of how we can use Apache Commons IO to achieve this:
@Grab('commons-io:commons-io:2.6')
import org.apache.commons.io.FileUtils
def file1 = new File("path/to/manifest1.mf")
def file2 = new File("path/to/manifest2.mf")
def attributes1 = new Manifest(file1.newInputStream()).mainAttributes
def attributes2 = new Manifest(file2.newInputStream()).mainAttributes
if (attributes1 == attributes2) {
println "Manifest file attributes are identical"
} else {
// Additional logic for handling the attribute differences
println "Manifest file attributes are different"
}
In this code snippet, we use Apache Commons IO to read the manifest file attributes and compare them. This allows for a more structured and detailed comparison of the manifest files, taking into account their specific attributes.
These are just a couple of strategies for comparing manifest files in Groovy. Keep in mind that the approach you choose may vary depending on the specific requirements of your application and the level of granularity needed for the comparison.
Final Considerations
Comparing manifest files is a crucial aspect of Java application development, and Groovy provides a variety of strategies to achieve this. Whether you opt for a simple textual comparison or a more detailed attribute-based approach, Groovy's flexibility and powerful libraries make it a great choice for handling manifest file comparisons.
Remember to choose an approach that best suits your application's requirements and complexity. As always, testing your code with various manifest file scenarios is essential to ensure the accuracy and reliability of the comparison strategies.
For more in-depth details on working with manifest files in Java and Groovy, check out Oracle's official documentation and Apache Commons IO documentation.
Start implementing these strategies today to bolster the efficiency and reliability of your Java applications!