Maximizing Efficiency: How to Squeeze More into Data Structures
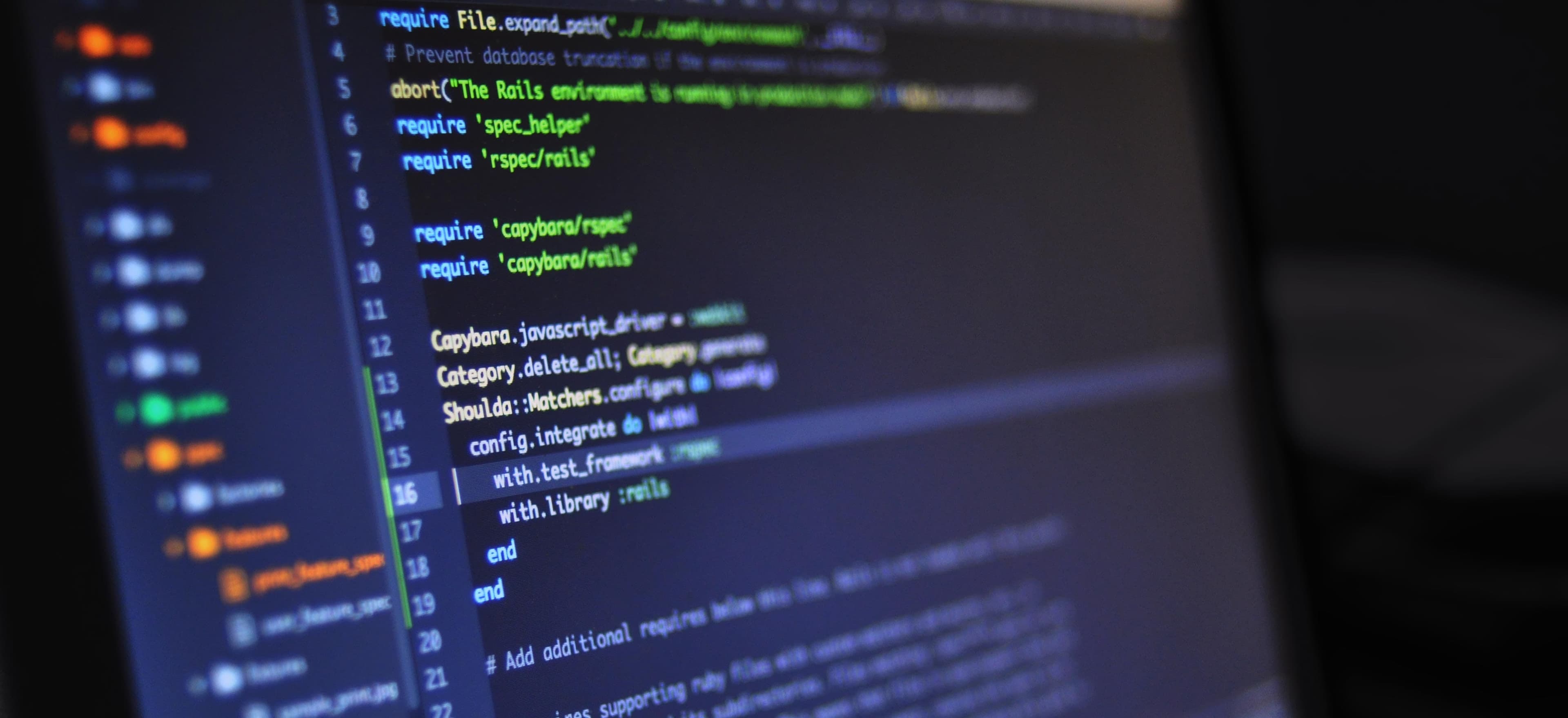
- Published on
Maximizing Efficiency: How to Squeeze More into Java Data Structures
In the world of Java development, efficiency is king. Whether you're building large-scale enterprise systems or compact mobile apps, making the most of the resources at your disposal is paramount. Data structures, the backbone of any application, play a crucial role in achieving this efficiency. In this post, we're diving into ways to maximize the effectiveness of Java data structures, ensuring your applications run faster, consume less memory, and provide an overall smoother user experience.
Understanding Java Data Structures
Before we delve into optimization strategies, it's vital to have a firm grasp on the core data structures available in Java. Arrays, Lists, Maps, and Sets each serve unique purposes and come with their own sets of advantages and drawbacks. Familiarizing yourself with these structures is the first step towards writing more efficient Java code. For an in-depth understanding, Oracle's Java documentation provides a comprehensive overview, which can be found here.
Strategy 1: Choose the Right Data Structure
The most fundamental step in optimizing your use of data structures in Java is selecting the most appropriate one for your needs. This decision should be based on the operations your application performs most frequently. For example, if you need fast access to elements by index, an ArrayList
might be your best bet. However, if your primary operation is to maintain a sorted collection of unique elements, a TreeSet
could be more fitting.
Example: Using a LinkedList
for Frequent Insertions/Deletions
import java.util.LinkedList;
public class LinkedListExample {
public static void main(String[] args) {
LinkedList<String> names = new LinkedList<>();
names.add("Alice");
names.add("Bob");
// Insertion at the beginning is efficient in a LinkedList
names.addFirst("Zoe");
// Deletion is also efficient
names.removeLast();
System.out.println(names);
}
}
This example illustrates the use of a LinkedList
when frequent insertions and deletions are expected. Unlike an ArrayList
, a LinkedList
excels at adding or removing elements without having to shift the rest of the elements, making it a more efficient choice in such scenarios.
Strategy 2: Optimize Capacity and Load Factor
Especially relevant for data structures like HashMap
and ArrayList
, understanding and tweaking the capacity and load factor can lead to significant performance gains.
-
ArrayList Capacity: An
ArrayList
in Java grows dynamically, but resizing is a costly operation. Pre-specifying the capacity of anArrayList
when its size is known in advance can avoid costly resizing operations. -
HashMap Load Factor and Initial Capacity: Similarly, a
HashMap
's performance can be largely affected by its load factor and initial capacity. The load factor dictates when the map is resized based on the number of key-value pairs it contains relative to its capacity. Adjusting these parameters can optimize performance by reducing the need for rehashing.
Example: Optimizing an ArrayList
import java.util.ArrayList;
public class OptimizeArrayList {
public static void main(String[] args) {
// Assume we roughly know we'll be storing approximately 1000 elements
ArrayList<String> largeList = new ArrayList<>(1000);
// Populate the ArrayList...
}
}
By initializing the ArrayList
with an initial capacity close to its expected size, we minimize the need for resizing as elements are added.
Strategy 3: Custom Data Structures for Specialized Needs
Sometimes, the standard data structures available in Java aren't perfectly suited to your specific needs. In such cases, implementing a custom data structure might be the way to go. A tailored data structure can be optimized for your particular use case, potentially offering substantial efficiency improvements.
Example: Building a Custom Stack
public class IntStack {
private int[] stack;
private int top;
private int size;
public IntStack(int size) {
this.size = size;
stack = new int[size];
top = -1;
}
public void push(int item) {
if (top + 1 < size) {
top++;
stack[top] = item;
} else {
System.out.println("Stack overflow");
}
}
public int pop() {
if (top >= 0) {
int item = stack[top];
top--;
return item;
} else {
System.out.println("Stack underflow");
return -1;
}
}
// Additional methods like isEmpty, isFull, peek could be added...
}
This example demonstrates how we can implement a basic stack optimized for int
storage. This custom data structure could be particularly efficient if your application heavily relies on basic integer operations, as it avoids the overhead of object wrapper classes (e.g., Integer).
Strategy 4: Use Streams and Optional for Cleaner Code
Java 8 introduced Streams and the Optional class, which can not only make your code cleaner but also more efficient in certain scenarios. For instance, Streams enable efficient filtering, sorting, and mapping operations on collections without the need for verbose loop constructs. For further reading on Java Streams, I highly recommend this guide published by Oracle: Java Streams Guide.
Example: Filtering a List Using Streams
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class StreamFilterExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "Diane");
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("A"))
.collect(Collectors.toList());
System.out.println(filteredNames); // Output: [Alice]
}
}
This example highlights how a Stream can be used to efficiently filter a list based on a condition, producing cleaner code than traditional iteration methods.
In Conclusion, Here is What Matters
Efficiently utilizing data structures is a cornerstone of optimal Java programming. By carefully selecting the appropriate data structure for your needs, optimizing parameters such as capacity and load factor, considering custom data structures when necessary, and leveraging modern features like Streams and Optional, you can significantly enhance your Java application's performance and resource usage. Remember, the key to mastering Java efficiency lies in understanding not just 'how' but also 'why' a particular data structure or strategy is the best fit for your specific scenario.