Ace Your Interview: Master Top 40 Java 8 Questions Explained
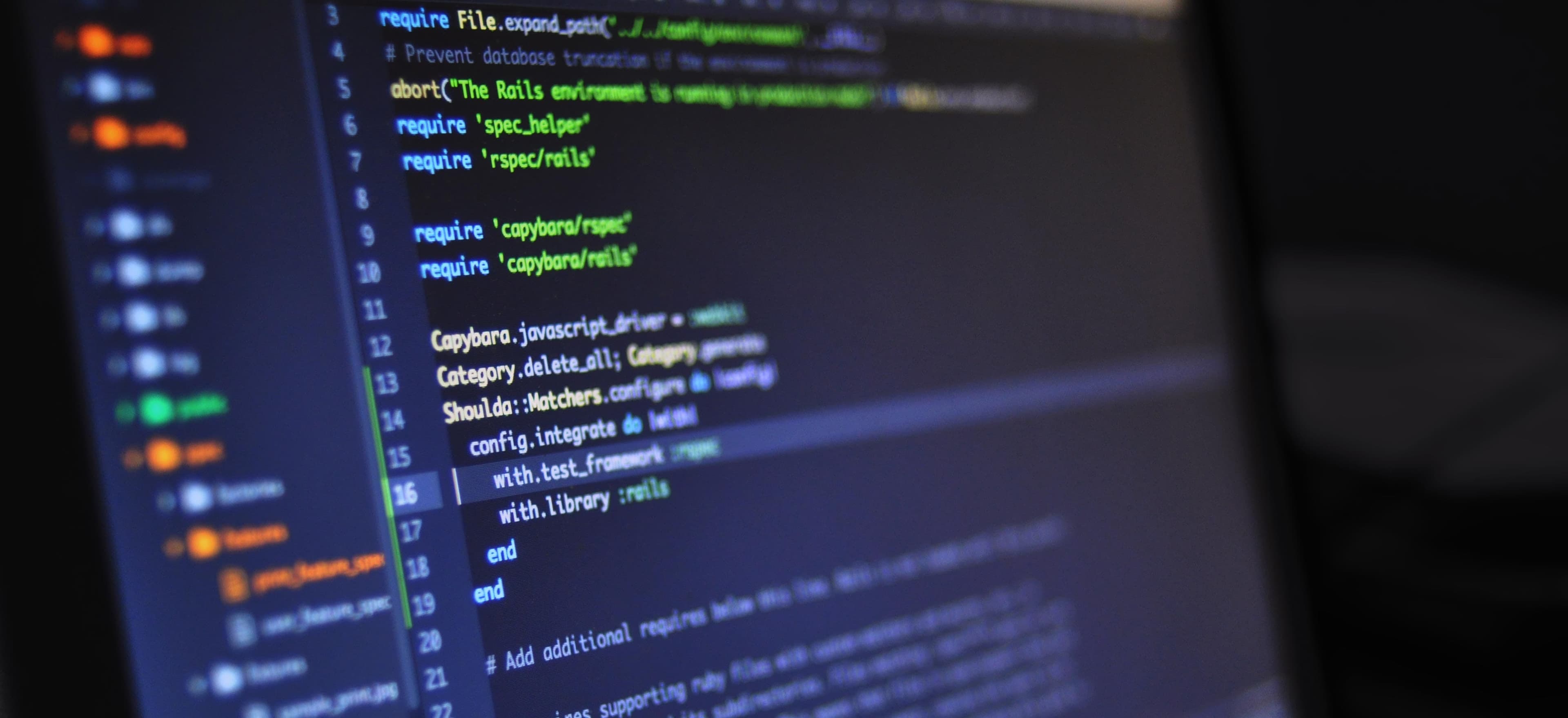
- Published on
Top 40 Java 8 Interview Questions and Answers - Explained
As a Java developer, preparing for a job interview can be daunting, especially when it comes to Java 8, which introduced several new features and enhancements. To help you ace your next interview, I've compiled a list of the top 40 Java 8 interview questions, along with detailed explanations and code examples.
1. What are the main features of Java 8?
Java 8 introduced several groundbreaking features, including lambda expressions, the Stream API, default methods in interfaces, the java.time package for date and time manipulation, and the Optional class.
2. How are lambda expressions used in Java 8?
Lambda expressions enable you to treat functionality as a method argument, or code as data. They provide a concise way to represent a method implementation using a more compact syntax. Here's an example of a lambda expression that adds two numbers:
BinaryOperator<Integer> add = (a, b) -> a + b;
3. Explain the Stream API in Java 8.
The Stream API provides a new abstraction for processing sequences of elements. It allows for functional-style operations on streams of elements such as map, filter, and reduce, which can be performed in parallel. Here's a simple example of using the Stream API to filter even numbers and collect them into a list:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6);
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
4. What are default methods in interfaces?
Default methods enable the addition of new methods to interfaces without breaking existing implementations. This allows interfaces to be extended without causing compatibility issues with existing code. For example:
interface MyInterface {
default void myMethod() {
System.out.println("Default method in interface");
}
}
5. How does the java.time package improve date and time handling in Java 8?
The java.time package provides a comprehensive set of classes for date and time manipulation, which address the shortcomings of the legacy java.util.Date and java.util.Calendar classes. It introduces new classes like LocalDate, LocalTime, and LocalDateTime, and handles concepts like time zones and daylight saving time more effectively.
6. What is the Optional class in Java 8 used for?
The Optional class is a container that may or may not contain a non-null value. It helps in avoiding null pointer exceptions by forcing the programmer to handle the absence of a value explicitly. Here's a simple example of using the Optional class:
Optional<String> opt = Optional.of("Hello");
System.out.println(opt.orElse("Default Value"));
7. How do you use method references in Java 8?
Method references provide a way to refer to methods or constructors without invoking them. They are a shorthand notation when passing methods as lambda expressions. For example, you can use a method reference to System.out::println instead of a lambda expression when printing elements of a stream.
8. What are the new functional interfaces in Java 8?
Java 8 introduced a set of functional interfaces in the java.util.function package, such as Predicate, Function, Supplier, and Consumer, which provide target types for lambda expressions and method references.
9. What are the advantages of using the parallel streams in Java 8?
Parallel streams leverage multiple cores of the CPU to process the data in a stream concurrently, potentially providing significant performance improvements for computationally intensive operations on large datasets.
10. Explain the new Date and Time API in Java 8.
The new Date and Time API in Java 8 provides classes that are immutable and thread-safe, making them superior to the legacy Date and Calendar classes. It also provides a clear separation of date and time, making it easier to work with date and time values.
If you're preparing for a Java interview, solid knowledge of Java 8 features and enhancements is crucial. Understanding these key concepts and being able to provide clear and concise explanations will undoubtedly set you apart from other candidates.
For more in-depth explanations and practice questions, check out the Java 8 tutorial provided by Oracle and the official Java documentation.
Good luck with your interview preparation, and remember, practice makes perfect!
Checkout our other articles