Simplify Your Coding: Master Multi-Project Builds with Gradle
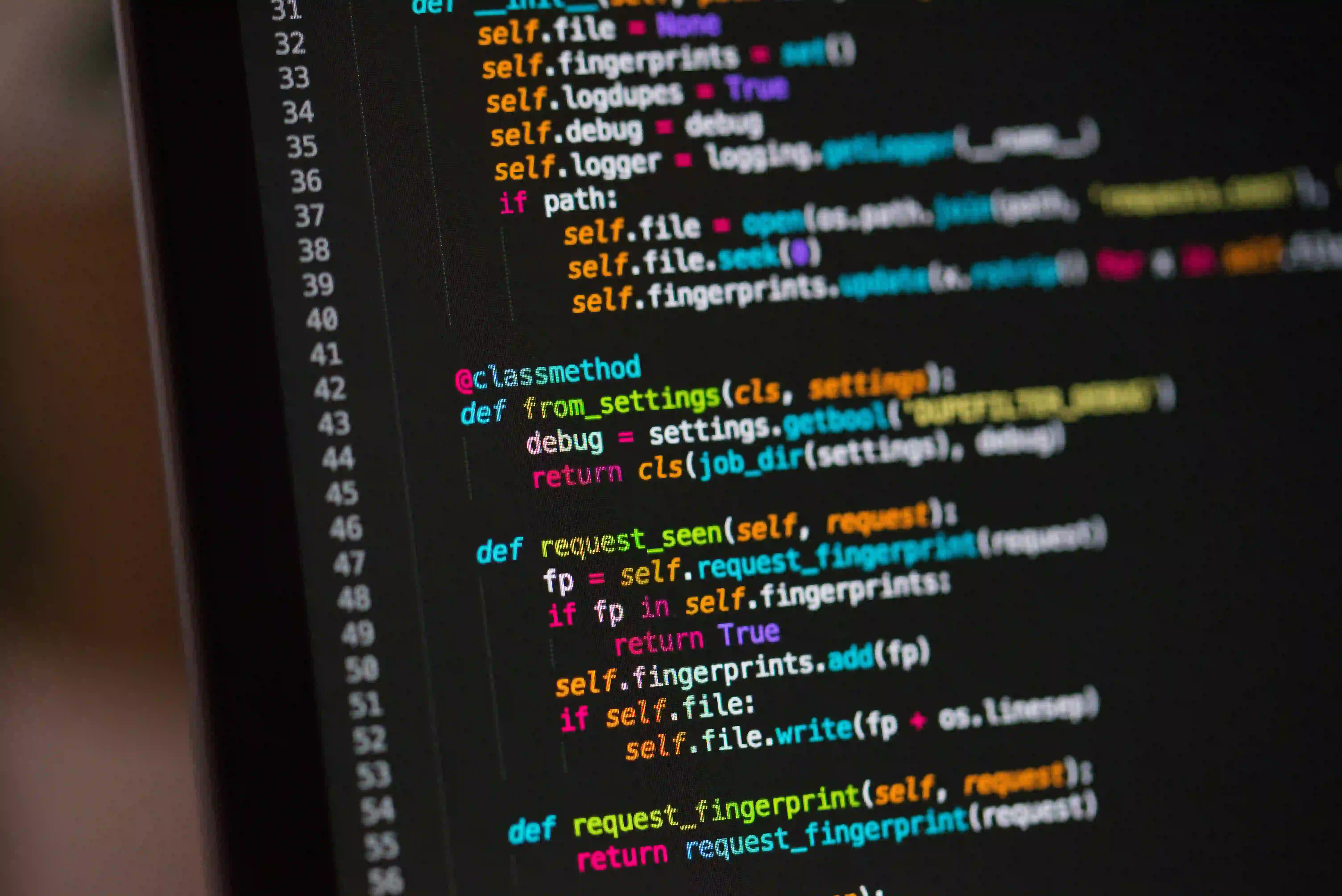
Simplify Your Coding with Gradle
As a Java developer, managing multiple projects within a single codebase can be a challenging task. Thankfully, Gradle, a powerful build automation tool, offers a solution to this problem. In this blog post, we will explore how to use Gradle to master multi-project builds, simplify your coding process, and improve your overall development experience.
What is Gradle?
Gradle is an advanced build automation tool that provides support for multi-project builds, allowing you to manage and build multiple interdependent projects as a single entity. It uses a Groovy-based domain-specific language (DSL) or Kotlin for scripting, and it offers tremendous flexibility and extensibility, making it an ideal choice for complex build scenarios.
Setting Up a Multi-Project Build
In this section, we will discuss how to set up a basic multi-project build using Gradle. Let's assume we have a simple Java application with the following project structure:
rootProject
āāā app
ā āāā build.gradle
āāā core
āāā build.gradle
The rootProject
is the main project that contains two sub-projects: app
and core
. To set up a multi-project build, we need to create a settings.gradle
file in the root project directory and include the sub-projects:
// settings.gradle
include 'app', 'core'
With this simple configuration, Gradle is now aware of the sub-projects, and we can start defining the build configurations for each project.
Defining Project Dependencies
One of the key benefits of using Gradle for multi-project builds is the ability to define and manage project dependencies effectively. Let's take a look at how we can define dependencies between the app
and core
projects.
// app/build.gradle
dependencies {
implementation project(':core')
}
In this example, we are declaring a dependency in the app
project on the core
project using the project()
notation. This allows the app
project to automatically include the output of the core
project in its build path, simplifying the management of project dependencies.
Sharing Common Configuration
Another advantage of using Gradle for multi-project builds is the ability to share common configurations across projects. For instance, if we want both the app
and core
projects to use the same version of a dependency, we can define it in the root build.gradle
file and have both projects inherit the configuration.
// rootProject/build.gradle
ext {
junitVersion = '5.7.1'
}
subprojects {
repositories {
mavenCentral()
}
dependencies {
testImplementation "org.junit.jupiter:junit-jupiter-api:$junitVersion"
testRuntimeOnly "org.junit.jupiter:junit-jupiter-engine:$junitVersion"
}
}
By defining the junitVersion
and the common dependencies in the root build.gradle
file, both the app
and core
projects will inherit these configurations, eliminating the need for duplicating the same dependencies in multiple places.
Building and Running Tasks
Gradle makes it easy to define and run tasks across multiple projects. For instance, if we want to build and run tests for all the projects in the codebase, we can use the following command:
$ ./gradlew build
This command will execute the build
task for all the projects, ensuring that each project is built and tested correctly.
Bringing It All Together
In this blog post, we have explored how Gradle can simplify the management of multi-project builds in Java development. By leveraging Gradle's powerful features such as project dependencies, shared configurations, and unified task execution, you can streamline your coding process and improve the organization of your codebase.
If you're interested in learning more about Gradle and its capabilities, I highly recommend checking out the official Gradle documentation at gradle.org.
In conclusion, Gradle offers a comprehensive solution for managing multi-project builds in Java, and mastering it can significantly improve your development workflow. So why not give it a try and see the difference it can make in simplifying your coding tasks?