Mastering WebSocket: Fixing Java Client-Server Sync
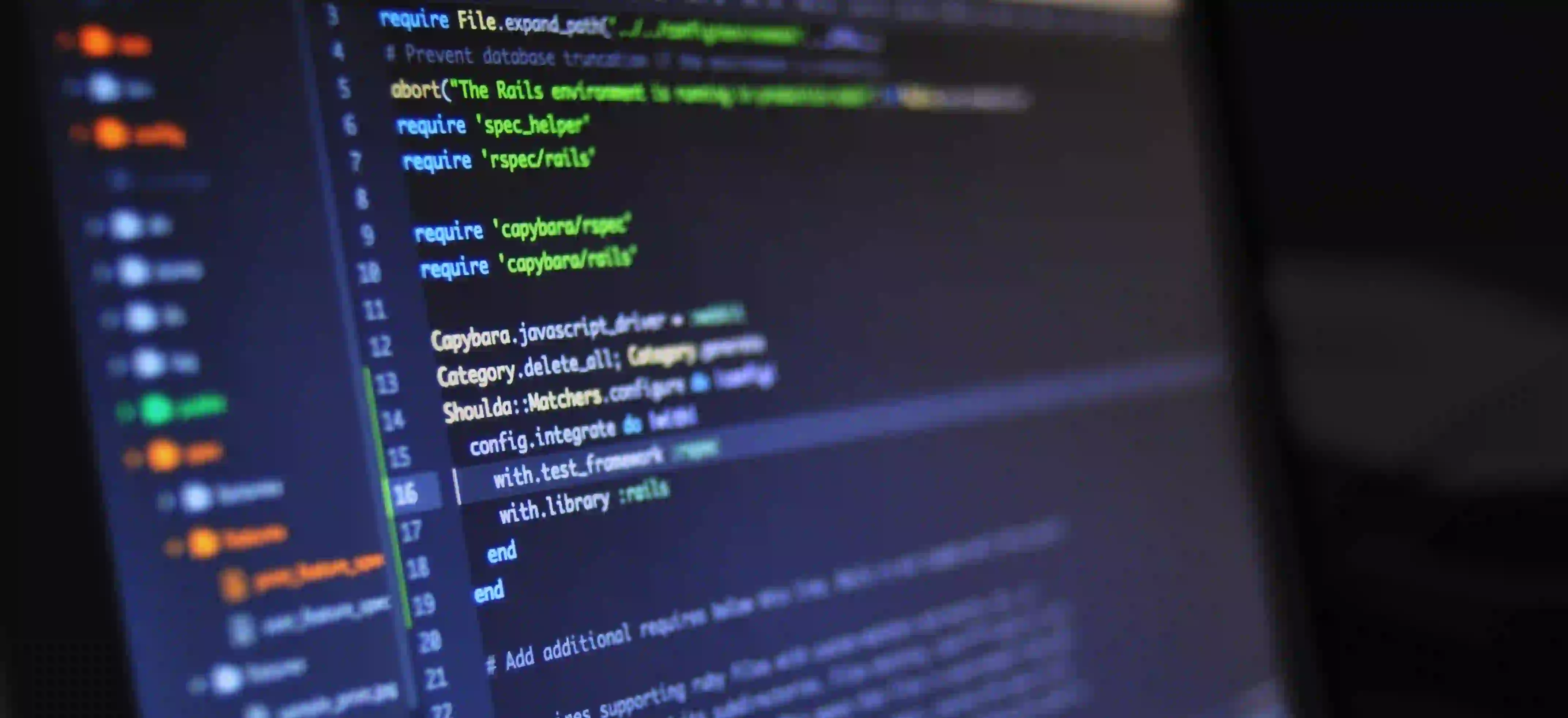
Mastering WebSocket: Fixing Java Client-Server Sync
WebSocket is a powerful communication protocol that allows full-duplex communication channels over a single TCP connection. This means it enables real-time data transfer between a client and a server. In the world of Java development, WebSocket plays a crucial role in building interactive and synchronized web applications.
The Challenge of Client-Server Synchronization
One of the common challenges developers face with WebSocket in Java is ensuring synchronization between the client and server. When dealing with real-time data, maintaining synchronization is essential for delivering a seamless user experience. Without proper synchronization, the client and server can fall out of sync, leading to issues such as delayed updates, missed messages, or inconsistent data.
Using Java WebSocket API
In Java, the standard way to work with WebSocket is through the Java WebSocket API, which provides a set of interfaces and classes for creating WebSocket endpoints. The API allows developers to build WebSocket endpoints for both clients and servers, making it a versatile tool for implementing real-time communication in Java applications.
Here's a simple example of how to create a WebSocket endpoint using the Java WebSocket API:
@ServerEndpoint("/websocket")
public class WebSocketServer {
@OnOpen
public void onOpen(Session session) {
// Handle connection opening
}
@OnMessage
public void onMessage(String message, Session session) {
// Handle incoming messages
}
@OnClose
public void onClose(Session session) {
// Handle connection closing
}
}
In this example, we define a WebSocket server endpoint using the @ServerEndpoint
annotation and implement methods to handle the opening, receiving, and closing of connections using the @OnOpen
, @OnMessage
, and @OnClose
annotations, respectively.
Fixing Sync Issues
To fix synchronization issues between the client and server in a Java WebSocket application, there are several strategies and best practices that developers can follow:
1. Implement Heartbeat Mechanism
Implementing a heartbeat mechanism can help ensure that the connection between the client and server remains active. By exchanging periodic heartbeat messages, the server can detect when a client connection is lost and take appropriate action, such as closing the connection or attempting to reconnect.
// Server-side heartbeat implementation
@OnMessage
public void onMessage(String message, Session session) {
if (message.equals("heartbeat")) {
// Update last received heartbeat timestamp
} else {
// Handle regular messages
}
}
In this example, the server checks incoming messages and distinguishes heartbeat messages from regular messages. If a heartbeat message is received, the server updates the last received heartbeat timestamp, allowing it to track the liveliness of the client connection.
2. Use Message Acknowledgments
Message acknowledgments can be used to ensure that messages sent from the client are successfully received and processed by the server. When a client sends a message to the server, it can include a unique identifier for the message. The server then acknowledges the receipt of the message by sending a confirmation back to the client.
// Server-side message acknowledgment
@OnMessage
public void onMessage(String message, Session session) {
// Process the message
// Send acknowledgment back to the client
}
By implementing message acknowledgments, developers can ensure that critical messages are not lost in transit and that both the client and server are aware of the status of each message.
3. Handle Reconnection Logic
In real-world scenarios, client-server connections can be interrupted due to network issues, client-side errors, or server restarts. Implementing reconnection logic on the client side can help mitigate these issues by automatically attempting to reconnect to the server when a connection is lost.
// Client-side reconnection logic
public void connectToWebSocketServer() {
// Establish WebSocket connection
// Handle connection errors and attempt reconnection
}
By incorporating reconnection logic in the client-side code, developers can enhance the robustness of the WebSocket communication and provide a more reliable user experience.
Closing Remarks
WebSocket is a valuable tool for building real-time communication capabilities in Java applications. By addressing synchronization issues between the client and server using strategies such as implementing a heartbeat mechanism, using message acknowledgments, and handling reconnection logic, developers can create seamless and responsive WebSocket-based applications.
In conclusion, mastering WebSocket in Java involves not only understanding the fundamentals of the Java WebSocket API but also implementing synchronization mechanisms to ensure a smooth and reliable real-time communication experience for users.
To delve deeper into WebSocket concepts and best practices, consider exploring the Java WebSocket API documentation and WebSocket RFC for a better understanding of the protocol specifications.
By mastering these techniques and continuously improving your understanding of WebSocket, you can elevate your Java development skills and build robust, real-time applications that delight users with synchronized, responsive interactions.
Remember, in the world of real-time communication, synchronization is key, and mastering WebSocket is the gateway to unlocking a world of seamless, interactive possibilities in Java development!