Overcoming Scala's Learning Curve for Modern App Dev
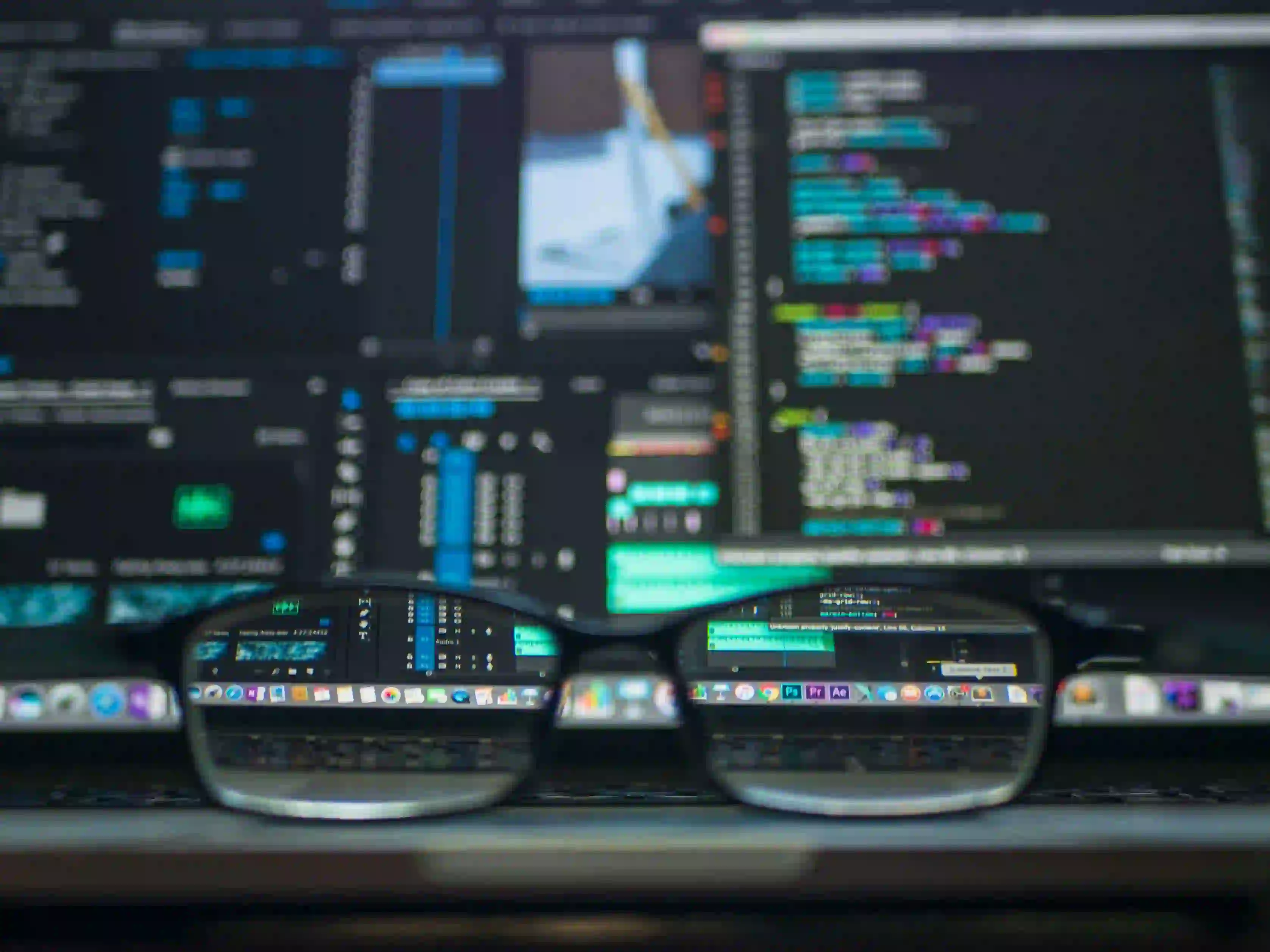
Overcoming Scala's Learning Curve for Modern App Development
Scala, a high-level, statically-typed programming language, is notable for its concise syntax and sophisticated features that enable developers to write robust, type-safe code efficiently. However, its enriched type system and functional programming paradigms often present a steep learning curve for newcomers, particularly those transitioning from more traditional, imperative programming languages like Java. In this blog post, we'll delve into strategies for overcoming Scala's learning curve, ensuring you can leverage its powerful features in your modern app development endeavors.
Understanding Scala: The Basics
Before we tackle the learning curve, let's briefly summarize what makes Scala stand out. Scala runs on the Java Virtual Machine (JVM), offering seamless interoperability with Java. This means you can use Java libraries and frameworks in your Scala projects, providing a vast ecosystem to support your development.
Scala encourages immutable data structures and variables, leading to safer, more predictable code. It also supports both object-oriented programming (OOP) and functional programming (FP) paradigms, allowing developers to choose the best approach for their project or even combine the two.
For developers accustomed to Java, Scala's concise syntax can initially seem unfamiliar. However, this conciseness reduces boilerplate code, which can enhance productivity once you're past the learning curve.
Example: Hello World in Scala
object HelloWorld {
def main(args: Array[String]): Unit = {
println("Hello, Scala world!")
}
}
Contrast this with the Java equivalent, and you'll notice fewer lines of code and no explicit class declaration required for this simple program.
Strategy 1: Start with the Familiar
Transitioning Java developers should leverage their existing knowledge. Understanding that Scala and Java share the JVM and can interoperate will make the journey less daunting. Begin by writing Scala code that resembles your Java code, then gradually refactor it to adopt Scala idioms and features.
Resource: Scala Documentation provides a great starting point, with guides specifically aimed at Java developers looking to transition.
Strategy 2: Embrace Functional Programming
Functional Programming (FP) is a core aspect of Scala. While FP can be challenging for developers steeped in the imperative paradigm, embracing it unlocks Scala's true potential. Start with the basics: immutable data structures, pure functions (functions without side effects), and higher-order functions (functions that take functions as parameters).
Example: Higher-Order Functions in Scala
val numbers = List(1, 2, 3, 4, 5)
// Defining a higher-order function that takes an Int, a function from Int to Int, and applies it
def applyFuncToList(nums: List[Int], func: Int => Int): List[Int] = nums.map(func)
// Using the function
val doubledNumbers = applyFuncToList(numbers, _ * 2)
println(doubledNumbers) // Output: List(2, 4, 6, 8, 10)
This example demonstrates how Scala's support for first-class functions makes it easy to transform data with less code compared to Java.
Resource: Functional Programming Principles in Scala is an excellent course for understanding Scala's FP concepts.
Strategy 3: Make Use of the Scala Community
The Scala community is vibrant and supportive. Numerous forums, Scala user groups, and conferences are available for beginners and experts alike. Engaging with the community can provide insights, encouragement, and practical advice as you navigate Scala's learning curve.
Strategy 4: Build Real Projects
Practice is crucial. Start with small projects or contribute to open-source Scala projects. This hands-on experience is invaluable, forcing you to confront and solve real-world problems with Scala, solidifying your understanding and skills along the way.
Strategy 5: Learn to Love the Type System
Scala's powerful type system is one of its most sophisticated features, offering a level of safety and expressiveness that's hard to match. Embrace the type system, learning how to use it to your advantage. This means getting comfortable with advanced concepts like variance, type bounds, and implicit values and conversions.
Example: Type Bounds in Scala
abstract class Animal {
def makeSound: String
}
class Dog extends Animal {
def makeSound: String = "Bark!"
}
class Cat extends Animal {
def makeSound: String = "Meow!"
}
// A generic method that accepts instances of Animal or its subclasses
def printAnimalSound[A <: Animal](animal: A): Unit = {
println(animal.makeSound)
}
In this example, <: Animal
denotes an upper type bound, ensuring that the printAnimalSound
method can accept any Animal
or its subclass, making use of Scala's type system to enforce this constraint at compile time.
A Final Look
Scala's learning curve can be steep, especially for those new to functional programming or coming from a different programming background. However, by leveraging existing Java knowledge, embracing functional programming, utilizing community resources, engaging in hands-on projects, and mastering Scala's type system, you can navigate this learning curve effectively. The payoff is the ability to write concise, robust, and type-safe code that can scale from simple scripts to complex enterprise applications.
Scala's blend of OOP and FP paradigms, coupled with its JVM interoperability, makes it a powerful tool in the modern developer's toolkit. While the journey to Scala proficiency may require time and effort, the benefits in terms of productivity, code safety, and application performance are well worth it.
Remember, becoming proficient in Scala is a journey, not a sprint. Be patient with yourself, and celebrate your milestones along the way. Happy coding!
Further Reading:
- For an in-depth guide on Scala's syntax and features, the official Scala book is a comprehensive resource.
- To explore more about Scala's interoperability with Java and how you can leverage your Java skills in Scala, check out Scala for Java Developers by Baeldung.