Cracking the Code: Why Coding Conventions are Crucial
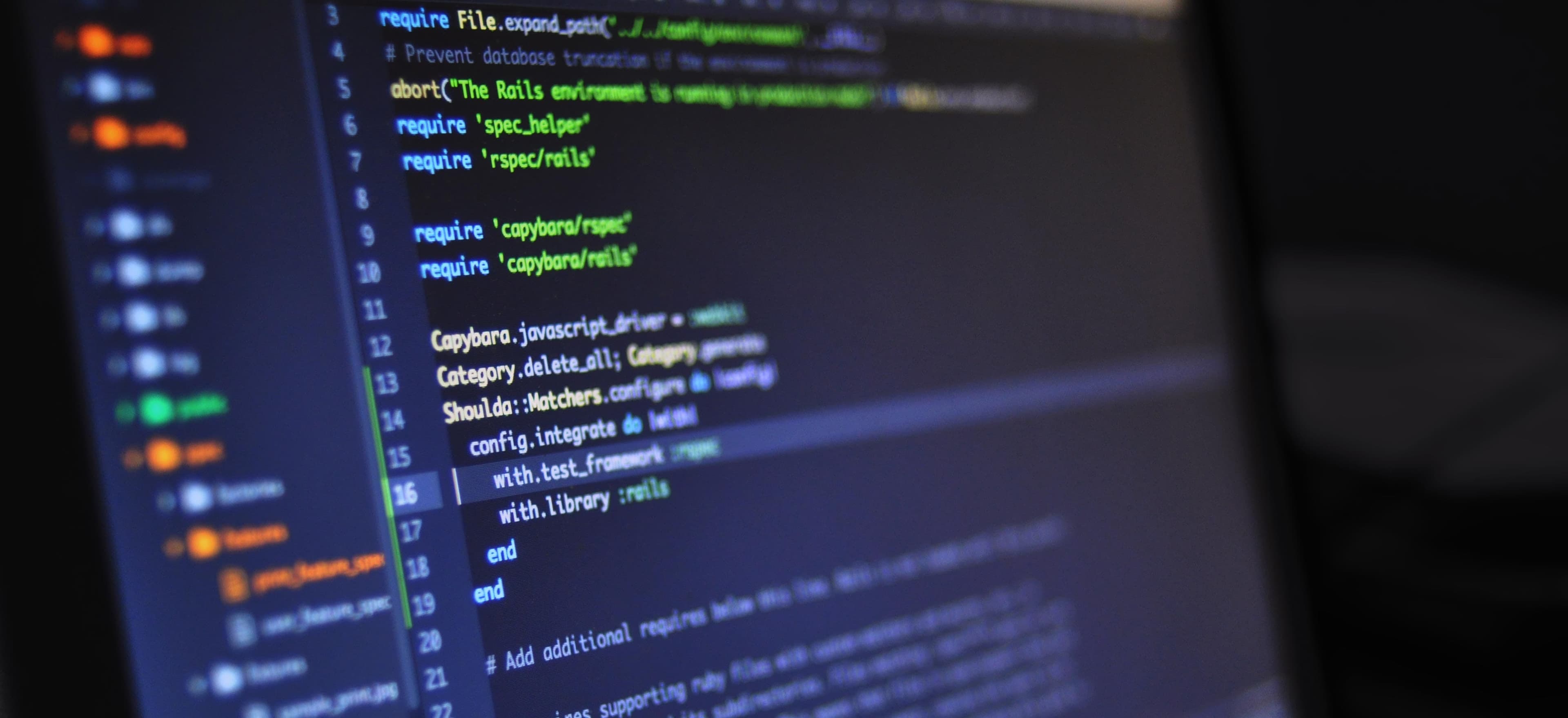
- Published on
Cracking the Code: Why Coding Conventions are Crucial
In the world of programming, the importance of coding conventions cannot be overstated. While it may seem like a mundane topic, adhering to a set of coding standards can significantly impact the readability, maintainability, and overall quality of a codebase. In this article, we'll delve into the reasons why coding conventions are crucial in Java development and how they can elevate your programming endeavors.
What are Coding Conventions?
Coding conventions encompass a set of guidelines, rules, and standards for writing code in a particular programming language. These conventions cover various aspects of code, including naming conventions, formatting, comments, and other stylistic choices. Adhering to coding conventions ensures that code written by different developers is consistent and easily comprehensible.
Readability and Maintainability
One of the primary benefits of following coding conventions is the enhancement of code readability. When code is easy to read and understand, it becomes more maintainable, and the likelihood of introducing bugs during maintenance decreases. Consistent naming conventions and formatting make it easier for developers to navigate through the codebase and comprehend the functionality of different components.
Collaboration and Team Efficiency
In a collaborative development environment, where multiple developers are working on the same codebase, adherence to coding conventions is paramount. Consistent coding styles streamline collaboration by reducing the time spent on deciphering unfamiliar code structures. This leads to improved team efficiency and facilitates seamless code integration.
Code Reviews and Quality Assurance
Coding conventions play a vital role in code reviews and quality assurance processes. Consistently formatted code with descriptive naming conventions and well-placed comments simplifies the review process, enabling reviewers to focus on the logic and functionality rather than deciphering the code's structure.
Java Coding Conventions
Java, being a widely used programming language, has established coding conventions outlined by Oracle in the Java Code Conventions document. Adhering to these conventions not only aligns your code with industry best practices but also ensures consistency across Java projects. Let's explore some key aspects of Java coding conventions.
Naming Conventions
Classes and Interfaces
public class MyClass {
// Class implementation
}
public interface MyInterface {
// Interface definition
}
Classes and interfaces should be named using PascalCase to enhance readability and distinguish them from methods and variables.
Variables
int myVariable = 10;
String myString = "Hello, World!";
Variables should be named using camelCase to denote a clear distinction from class and interface names.
Code Formatting
Proper indentation and code formatting significantly impact the readability and organization of Java code. Let’s take a look at an example of well-formatted code:
public class Example {
public void performAction() {
if (condition) {
statement1;
statement2;
} else {
alternativeStatement;
}
}
}
The use of consistent indentation and braces placement enhances code clarity and makes it easier to follow the control flow.
Comments
// This is a single-line comment
/*
* This is a multi-line comment
* Providing additional context or explanation
*/
Java supports both single-line and multi-line comments, and they should be used effectively to document the code and provide insights into its functionality.
Why Follow Java Coding Conventions?
Consistency Across Projects
Adhering to Java coding conventions ensures consistency not only within a single project but also across multiple projects. This uniformity simplifies the onboarding process for new developers joining the team and promotes a cohesive codebase across the organization.
Tool Integration and Automation
Many development tools and frameworks in the Java ecosystem rely on coding conventions to optimize various processes. IDEs use coding standards to auto-format code, static analysis tools leverage conventions to identify potential issues, and build tools can enforce coding standards as part of the build process.
Industry Best Practices
The Java coding conventions, established by Oracle, align with industry best practices and reflect the collective wisdom of experienced Java developers and architects. Adhering to these conventions not only maintains code consistency but also ensures that your code meets industry standards.
Wrapping Up
In conclusion, coding conventions are essential for fostering a robust and maintainable codebase. In the context of Java development, adherence to coding conventions, such as those outlined in the Java Code Conventions document, ensures consistency, readability, and alignment with industry best practices. By following coding conventions, developers can streamline collaboration, simplify code reviews, and elevate the overall quality and maintainability of their code. Embracing coding conventions is not just a best practice; it's a fundamental aspect of professional and efficient software development in Java.