Unveiling the Limitations of Stack Traces
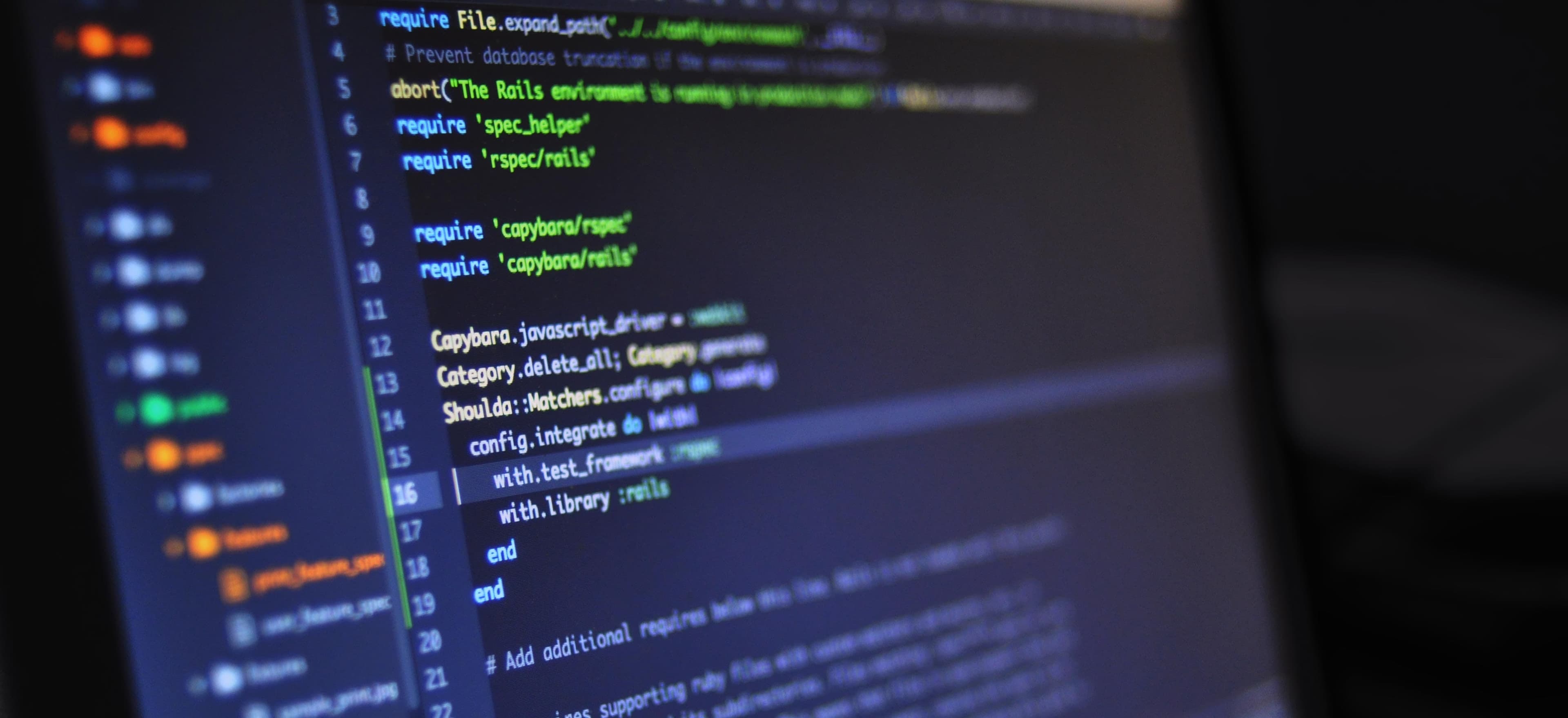
- Published on
Unveiling the Limitations of Stack Traces
When it comes to debugging Java applications, stack traces are invaluable resources. They provide a detailed report of the call stack at a particular point in time, helping developers pinpoint the source of errors in their code. However, despite their importance, stack traces have limitations that can hinder the debugging process. In this article, we will delve into the shortcomings of stack traces and explore alternative approaches to overcome these limitations.
The Anatomy of a Stack Trace
Before delving into the limitations, let's first understand the basic structure of a stack trace. When an exception is thrown in a Java application, the JVM generates a stack trace, which consists of a series of method calls that led to the exception. Each line in the stack trace represents a method call, with the topmost line indicating the method where the exception was thrown.
Exception in thread "main" java.lang.NullPointerException
at com.example.MyClass.myMethod(MyClass.java:10)
at com.example.MyClass.main(MyClass.java:6)
In the above example, the stack trace indicates that a NullPointerException
occurred in the myMethod
of MyClass
, which was called from the main
method.
Limitations of Stack Traces
Limited Context
One of the primary limitations of stack traces is their lack of context. While they provide information about the sequence of method calls leading to an exception, they do not offer insights into the state of the application at each method invocation. This makes it challenging to understand the underlying cause of the exception, especially in complex codebases.
No Visibility into Variable Values
Stack traces also do not reveal the values of variables at each method invocation. As a result, developers are unable to inspect the specific data that might have led to the exception. This can make it difficult to reproduce and diagnose the issue, especially when the codebase is large and unfamiliar.
Overabundance of Information
On the contrary, stack traces can sometimes overwhelm developers with excessive information, particularly in large applications with numerous nested method calls. Parsing through a lengthy stack trace to identify the root cause of an exception can be time-consuming and prone to errors.
Inability to Capture Control Flow
Stack traces capture the call stack at a specific point in time, but they do not provide a comprehensive view of the application's control flow. As a result, it can be challenging to trace the logical sequence of method invocations leading to an exception, making it harder to understand the code's behavior.
Overcoming the Limitations
While stack traces are indispensable for identifying the origin of exceptions, they are not the only tool at a developer's disposal. To overcome the limitations discussed above, consider leveraging the following approaches:
Logging
Incorporating comprehensive logging within your application can provide a wealth of contextual information that complements stack traces. Log messages can capture variable values, method entry and exit points, and other critical information that enhances the understanding of the application's behavior.
public void myMethod(int userId) {
log.info("Entering myMethod with userId: " + userId);
// Method logic
log.info("Exiting myMethod");
}
By strategically placing log statements throughout your codebase, you can gain visibility into the application's state and control flow, supplementing the information provided by stack traces.
Exception Wrapping and Contextual Information
Sometimes, it's beneficial to wrap exceptions and enrich them with additional contextual information before rethrowing them. By including relevant details such as parameter values or intermediate state, you can provide more comprehensive insights into the cause of an exception.
try {
// Risky operation
} catch (Exception e) {
throw new CustomException("An error occurred while processing data for user " + userId, e);
}
Wrapping exceptions in this manner can augment the information available in stack traces, facilitating a more thorough diagnosis of issues.
Stack Trace Analysis Tools
Several tools and libraries exist that can analyze and visualize stack traces to provide a clearer representation of the control flow and method invocations. These tools can help developers navigate and comprehend complex stack traces, reducing the time and effort required to identify the root cause of exceptions.
My Closing Thoughts on the Matter
While stack traces serve as fundamental diagnostic tools in Java development, they have inherent limitations that necessitate complementary approaches to effective debugging. By combining the use of stack traces with logging, exception wrapping, and stack trace analysis tools, developers can enhance their ability to identify and resolve issues in their applications, leading to more robust and maintainable codebases.
In conclusion, the limitations of stack traces should not hinder the debugging process but rather serve as prompts to explore alternative strategies for gaining deeper insights into the behavior of Java applications.
For further insights into Java application debugging, consider exploring frameworks like Spring Boot that offer advanced debugging and monitoring capabilities.
Happy debugging!