Spring Integration vs. Apache Camel: Making the Right Choice
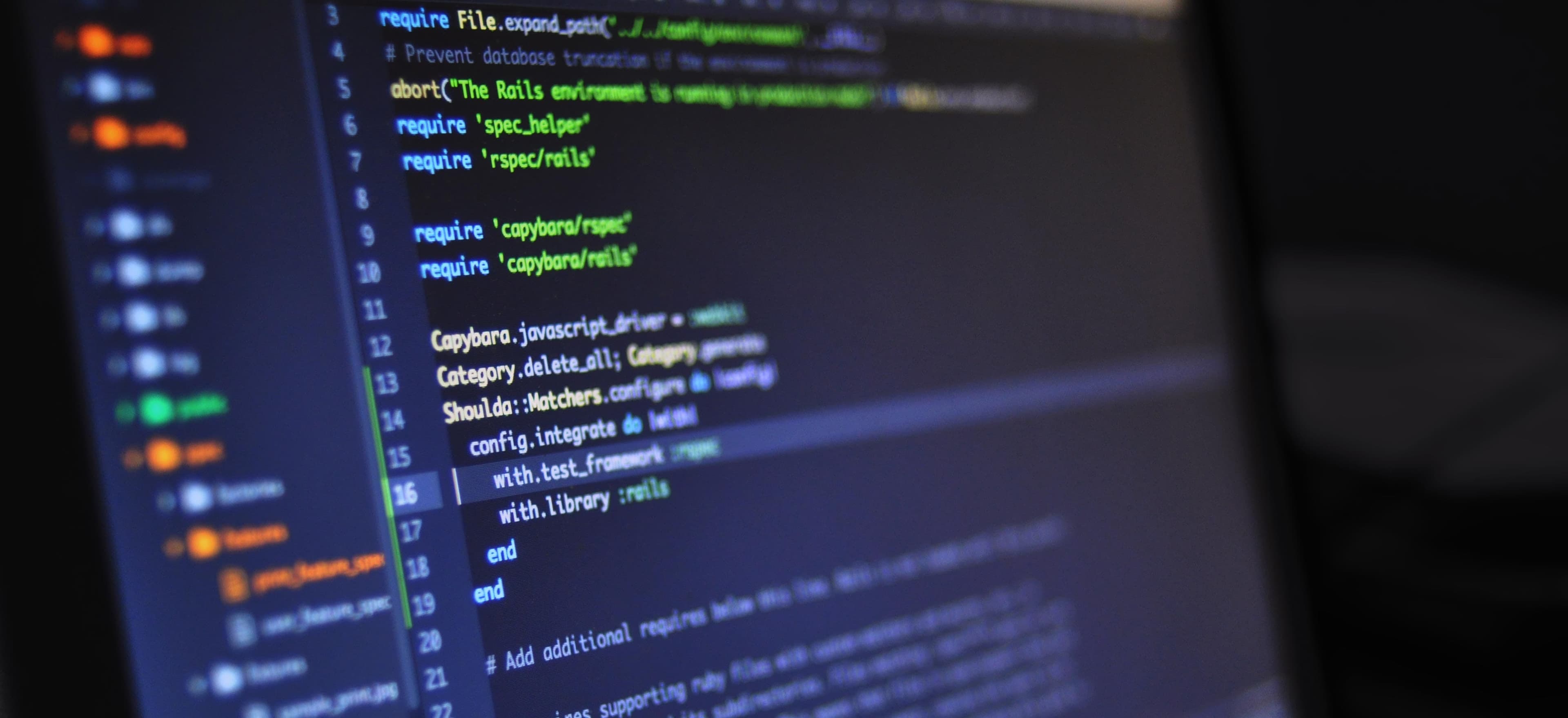
- Published on
Spring Integration vs. Apache Camel: Making the Right Choice
When it comes to integrating systems and applications in a Java environment, developers often find themselves at a crossroads, evaluating the pros and cons of different integration frameworks. In this post, we will dive into the comparison between two popular choices: Spring Integration and Apache Camel. Both frameworks offer powerful tools for building integration solutions, but they have their differences in terms of design philosophy, ease of use, and community support. By the end of this post, you'll be equipped with the knowledge to make an informed decision on which framework best suits your integration needs.
Spring Integration: Seamless Integration with Spring Ecosystem
Spring Integration, as the name suggests, is a natural extension of the Spring ecosystem. It provides a set of abstractions and implementations for building messaging and integration solutions. One of the key strengths of Spring Integration is its seamless integration with the Spring framework, leveraging concepts such as dependency injection and aspect-oriented programming for building message-driven architectures.
Example of Spring Integration Code
@MessagingGateway
public interface OrderGateway {
@Gateway(requestChannel = "orderChannel")
void placeOrder(Order order);
}
@Service
public class OrderProcessor {
@ServiceActivator(inputChannel = "orderChannel")
public void processOrder(Order order) {
// Processing logic here
}
}
In the above code, the OrderGateway
interface and OrderProcessor
class demonstrate how Spring Integration simplifies the implementation of messaging gateways and service activators, providing a clear and concise way to handle message processing.
Apache Camel: Domain-Specific Language for Integration
On the other hand, Apache Camel takes a different approach by providing a domain-specific language (DSL) for expressing integration patterns. Camel offers a wide range of components and connectors to interact with various systems and protocols, allowing developers to define routes and mediation rules in a highly expressive and readable manner.
Example of Apache Camel Route
from("file:inputDir?noop=true")
.to("jms:queue:orders");
The above code snippet showcases the simplicity and readability of Apache Camel's DSL. The route specifies a simple file-to-JMS queue integration, illustrating how Camel abstracts away the complexities of integration logic into a concise and easy-to-understand format.
Feature Comparison
Spring Integration Features
- Tight Integration with Spring: Leverages Spring's powerful features for building enterprise integration solutions.
- Declarative Configuration: Supports configuration through XML or Java annotations, promoting a clear separation of concerns.
- Extensive Enterprise Patterns: Provides a rich set of enterprise integration patterns out of the box, making it easier to implement complex integration scenarios.
Apache Camel Features
- Expressive DSL: Offers a fluent and expressive DSL for defining integration routes, making the code highly readable and maintainable.
- Rich Component Ecosystem: Boasts a wide range of components and connectors for interacting with diverse systems and protocols, promoting flexibility and interoperability.
- Built-in Error Handling: Includes comprehensive error handling and retry mechanisms, ensuring robustness in integration workflows.
Considerations for Choosing the Right Framework
Integration Paradigm
When deciding between Spring Integration and Apache Camel, it's essential to consider the integration paradigm that aligns with your project requirements. Spring Integration's seamless integration with the Spring ecosystem makes it an attractive choice for projects already leveraging the Spring framework for other components. On the other hand, Apache Camel's expressive DSL and extensive component ecosystem are advantageous for scenarios requiring diverse system interactions and complex routing logic.
Community and Support
Both Spring Integration and Apache Camel have active and supportive communities, offering online resources, forums, and frequent updates. However, considering the specific use cases and industry adoption of each framework can provide valuable insights into long-term support and maintenance considerations for your integration solution.
Learning Curve
The learning curve associated with each framework is another critical factor. Spring Integration, being closely tied to the Spring framework, can be more approachable for developers familiar with Spring concepts. Conversely, developers with experience in Enterprise Integration Patterns (EIP) or those looking for a highly expressive integration DSL may find Apache Camel more intuitive to work with.
Wrapping Up
In conclusion, the choice between Spring Integration and Apache Camel boils down to the alignment of their features and design philosophy with the specific integration needs of your project. While Spring Integration excels in seamless Spring ecosystem integration and extensive enterprise patterns, Apache Camel shines in its expressive DSL and rich component ecosystem. By carefully evaluating the integration paradigm, community support, and learning curve, you can make an informed decision that sets the stage for a robust and efficient integration solution.
In the dynamic landscape of integration frameworks, both Spring Integration and Apache Camel continue to evolve, offering cutting-edge tools for developers to build scalable and resilient integration solutions. Ultimately, the right choice between the two frameworks will empower your team to drive innovation and seamless integration across your enterprise systems.
Empower your Java integration endeavors with the capabilities of these powerful frameworks, and stay ahead in the realm of seamless system communication and collaboration.
For more in-depth understanding and exploration, check out the resources on Spring Integration and Apache Camel.
Fill your tech arsenal with the best integration frameworks and make informed decisions for seamless system interoperability. Happy integrating!