Mastering JVM Memory Allocation: A Deep Dive
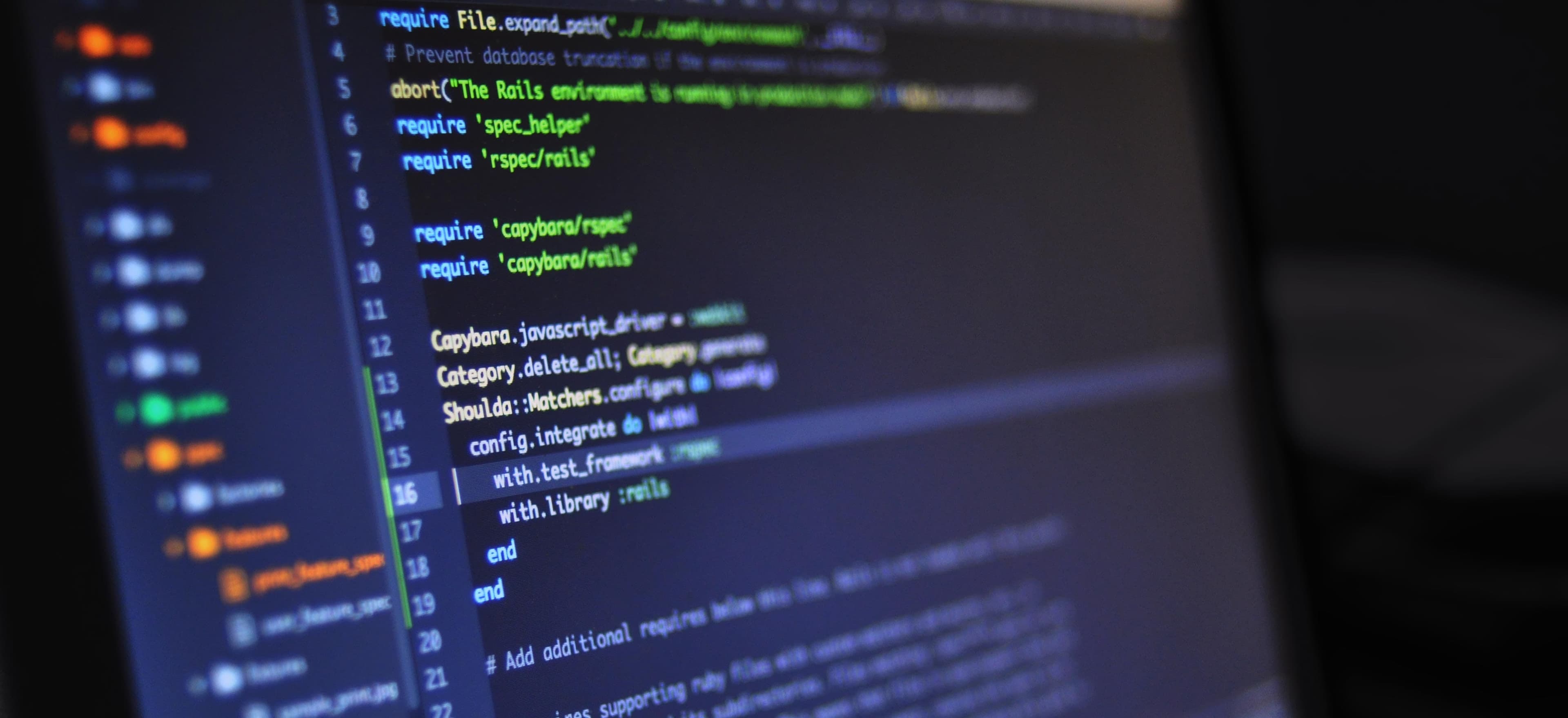
- Published on
Mastering JVM Memory Allocation: A Deep Dive
Java Virtual Machine (JVM) is the cornerstone of Java's high-level performance, offering unique capabilities that allow Java applications to function across different platforms. Understanding JVM memory allocation is essential for any Java developer looking to optimize application performance and scalability. This comprehensive guide delves into the intricacies of JVM memory allocation, equipping you with the knowledge to master this critical component of Java development.
Understanding JVM Memory Model
At the heart of Java's runtime environment is the JVM, a powerhouse that executes Java bytecode. The JVM memory model is a sophisticated mechanism designed to manage and allocate memory for Java applications efficiently. It comprises several key areas:
- Heap Memory: This is where objects are dynamically allocated. It's further divided into the Young Generation (for new objects) and Old Generation (for objects with longer lifetimes).
- Stack Memory: Each thread in your application has a private JVM stack, created at the same time as the thread, where local variables and method calls are stored.
- Method Area: This zone stores class structure information, including runtime constant pool, field, and method data.
- Runtime Constant Pool: A part of the method area, it contains constants and references to types and fields.
- Native Method Stacks: These are reserved for native code outside the JVM.
Understanding these components is pivotal in managing memory in Java applications and preventing memory leaks and OutOfMemoryErrors.
Diving Into Heap Memory
Heap memory is a focal point for Java developers, owing to its role in object storage and garbage collection. The segmentation of heap memory into the Young and Old generations is a strategic design that optimizes garbage collection efficiency, as most objects are short-lived.
Young Generation
This area is the birthplace for new objects. It's subdivided into:
- Eden Space: Where new objects are created.
- Survivor Spaces (S0 and S1): Spaces that hold objects that have survived the garbage collection in the Eden space.
Objects that survive garbage collection in the Young generation are moved to the Old generation. The garbage collection process in this area is fast and occurs frequently.
public class YoungGenDemo {
public static void main(String[] args) {
byte[] block1 = new byte[1024 * 1024]; // 1MB block allocated in Eden space
byte[] block2 = new byte[1024 * 1024]; // Another 1MB block, also in Eden
// block1 and block2 are potential candidates for garbage collection if not used further
}
}
In the example above, we create two 1MB objects that are allocated in the Eden space. These objects are potential garbage collection candidates, showcasing how quickly objects can transition through memory spaces in the Young generation.
Old Generation
The Old Generation, or Tenured Generation, stores long-living objects. Garbage collection (also known as Major GC or Full GC) in this area is less frequent but more intensive than in the Young generation, often leading to noticeable application pause times.
public class OldGenDemo {
private static final List<byte[]> container = new ArrayList<>();
public static void main(String[] args) {
while (true) {
container.add(new byte[1024 * 1024]); // Continuously adding 1MB objects to the list
try {
Thread.sleep(500); // Pause to simulate application activity
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
}
The above snippet illustrates continuous allocation of objects, eventually promoting them to the Old Generation. This demonstrates the journey of objects through JVM memory spaces and underscores the importance of monitoring and managing long-living objects for optimal performance.
Garbage Collection & Performance Tips
Garbage Collection (GC) in JVM is a mechanism that frees up memory by removing objects that are no longer in use. While Java abstracts much of the GC process, understanding how it works can greatly impact the performance of your applications. For a deep dive into Java's garbage collection mechanisms and how to tune them, Oracle's official documentation is an invaluable resource (Oracle's Garbage Collection Guide).
Performance Tips
- Monitor and Tune GC: Use tools like VisualVM or the GC logs to monitor GC activity and tune it to reduce pause times.
- Optimize Object Allocation: Be mindful of object creation and scope. Use local variables and minimize object scope when possible to aid quicker garbage collection.
- Memory Leak Identification: Utilize profiling tools to identify and fix memory leaks. Common causes include static collections, unclosed resources, and circular references.
public class MemoryLeakExample {
private static final Map cache = new HashMap();
public void addToCache(String key, Object value) {
cache.put(key, value);
}
// Remember to clear cache or remove entries when they are no longer needed!
}
In the MemoryLeakExample
above, objects added to cache
without a removal strategy can lead to memory leaks. It's critical to implement cache eviction policies to prevent such issues.
The Closing Argument
Mastering JVM memory allocation is a nuanced but rewarding endeavor that enhances the performance and scalability of Java applications. By understanding the JVM memory model, monitoring and tuning garbage collection, and adopting best practices for memory management, developers can significantly optimize their Java applications.
For further reading and advanced topics, another excellent resource is the official Java SE documentation (Java SE Documentation). Whether you're troubleshooting a memory leak, tuning garbage collector settings, or simply aiming to write more memory-efficient Java code, the wealth of knowledge available will guide you towards mastering JVM memory allocation.
Remember, optimizing JVM memory allocation is a continual process of learning, monitoring, experimenting, and refining. Embrace it, and you’ll be well on your way to becoming a Java performance maestro.
Checkout our other articles