Java Simplified: Mastering File and Folder Deletion
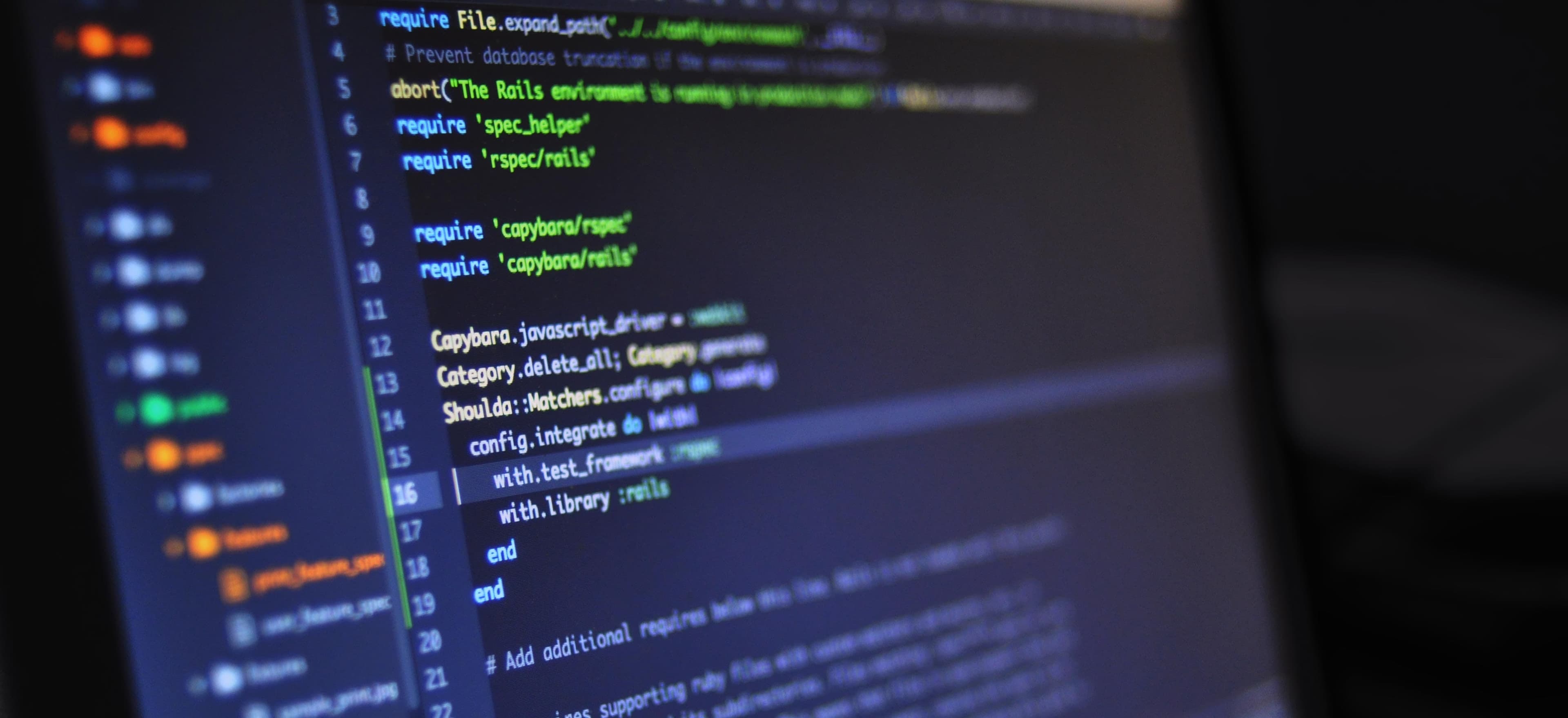
- Published on
Java Simplified: Mastering File and Folder Deletion
When working with Java, manipulating files and folders is a common task. Whether it's for cleaning up temporary files, handling user uploads, or managing your application's data, knowing how to properly delete files and folders is crucial.
In this guide, we'll dive into the various ways to delete files and folders in Java, covering different scenarios, potential pitfalls, and best practices.
Deleting a File
Using File.delete()
The straightforward way to delete a file in Java is by using the delete()
method provided by the File
class. Here's a simple example:
import java.io.File;
public class FileDeletionExample {
public static void main(String[] args) {
File file = new File("path/to/your/file.txt");
if (file.delete()) {
System.out.println("File deleted successfully");
} else {
System.out.println("Failed to delete the file");
}
}
}
This method returns a boolean value to indicate whether the file was successfully deleted. It's important to handle the possible failure case when the file cannot be deleted.
Using Files.delete()
With the introduction of the NIO (New I/O) API in Java 7, the Files
class provides a more versatile way to delete a file using the delete()
method from the java.nio.file
package:
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.io.IOException;
public class FileDeletionExample {
public static void main(String[] args) {
Path path = Paths.get("path/to/your/file.txt");
try {
Files.delete(path);
System.out.println("File deleted successfully");
} catch (IOException e) {
System.out.println("Failed to delete the file: " + e.getMessage());
}
}
}
This way, you can handle the deletion operation within a try-catch block to catch any potential IOException
that may occur during the deletion process.
Deleting a Folder and Its Contents
When it comes to deleting an entire folder along with its contents, the process becomes a bit more involved. Let's explore a few different approaches.
Using Apache Commons IO
Apache Commons IO library provides a convenient way to recursively delete a directory and its contents:
import org.apache.commons.io.FileUtils;
import java.io.File;
import java.io.IOException;
public class FolderDeletionExample {
public static void main(String[] args) {
File directory = new File("path/to/your/directory");
try {
FileUtils.deleteDirectory(directory);
System.out.println("Folder and its contents deleted successfully");
} catch (IOException e) {
System.out.println("Failed to delete the folder: " + e.getMessage());
}
}
}
By utilizing FileUtils.deleteDirectory()
, you can efficiently handle the deletion of a folder and its entire contents, including subdirectories and files.
Using Files.walkFileTree()
With the standard Java NIO package, you can achieve similar functionality using the Files.walkFileTree()
method to traverse the directory and delete its contents recursively:
import java.io.IOException;
import java.nio.file.*;
import java.nio.file.attribute.BasicFileAttributes;
public class FolderDeletionExample {
public static void main(String[] args) {
Path directory = Paths.get("path/to/your/directory");
try {
Files.walkFileTree(directory, new SimpleFileVisitor<Path>() {
@Override
public FileVisitResult visitFile(Path file, BasicFileAttributes attrs) throws IOException {
Files.delete(file);
return FileVisitResult.CONTINUE;
}
@Override
public FileVisitResult postVisitDirectory(Path dir, IOException exc) throws IOException {
Files.delete(dir);
return FileVisitResult.CONTINUE;
}
});
System.out.println("Folder and its contents deleted successfully");
} catch (IOException e) {
System.out.println("Failed to delete the folder: " + e.getMessage());
}
}
}
By using the Files.walkFileTree()
method along with a custom FileVisitor
, you can achieve fine-grained control over the deletion process while handling files and subdirectories recursively.
Deleting Files and Folders With Java 8 Stream API
Starting from Java 8, you can take advantage of the Stream API to perform file deletion operations more succinctly:
Deleting Files in a Directory
import java.io.IOException;
import java.nio.file.*;
import java.util.stream.Stream;
public class FileDeletionExample {
public static void main(String[] args) {
Path directory = Paths.get("path/to/your/directory");
try (Stream<Path> walk = Files.walk(directory)) {
walk.filter(Files::isRegularFile)
.forEach(file -> {
try {
Files.delete(file);
} catch (IOException e) {
System.out.println("Failed to delete file: " + file.toString());
}
});
System.out.println("All files in the directory deleted successfully");
} catch (IOException e) {
System.out.println("Failed to delete files: " + e.getMessage());
}
}
}
Here, we utilize the Files.walk()
method along with the Stream API to filter out regular files and delete them within the specified directory.
Deleting Directories
import java.io.IOException;
import java.nio.file.*;
public class FolderDeletionExample {
public static void main(String[] args) {
Path directory = Paths.get("path/to/your/directory");
try {
Files.walk(directory)
.sorted(Comparator.reverseOrder())
.map(Path::toFile)
.forEach(File::delete);
System.out.println("Directory and its contents deleted successfully");
} catch (IOException e) {
System.out.println("Failed to delete the directory: " + e.getMessage());
}
}
}
In this example, we use the Files.walk()
method to traverse the directory, then use the Stream API to perform a reverse-order deletion of the directory and its contents.
A Final Look
In Java, effectively managing file and folder deletion is essential for maintaining an organized file system and ensuring proper resource cleanup. Whether you're dealing with individual files or entire directory structures, Java provides several methods and libraries to simplify the deletion process.
By mastering the techniques presented in this guide, you can confidently handle file and folder deletion operations in your Java applications while adhering to best practices and efficient resource management.
In conclusion, as you continue to work with Java, understanding the intricacies of file and folder deletion will serve you well in navigating various file manipulation scenarios, ultimately contributing to the robustness of your applications.